Creating a Custom Breadcrumb Component in Next.js
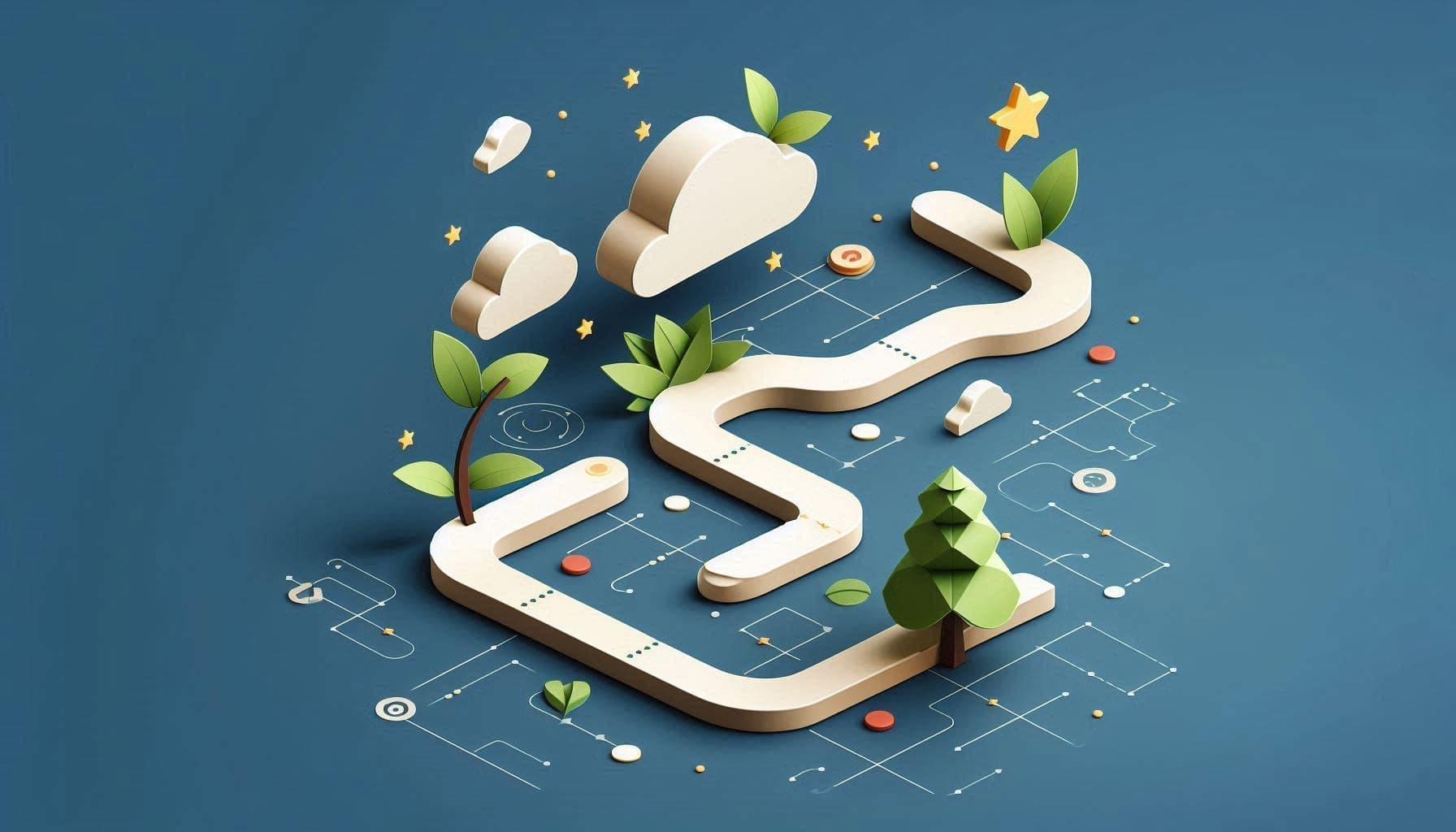
Breadcrumb Navigation in Next.js

Breadcrumb navigation is a key feature for enhancing user experience in web applications. It allows users to understand their current location within the app and easily navigate back to previous pages. In this guide, we'll walk through creating a dynamic breadcrumb component in a Next.js application.
Prerequisites
Before starting, ensure you have the following:
- Node.js, npm, and TypeScript installed.
- A basic Next.js project set up with TypeScript.
- (Optional) TailwindCSS for styling.
Step 1: Create the Breadcrumb Component
Begin by creating a new component named Breadcrumb.tsx
in your project. This component will dynamically generate the breadcrumb links based on the current path.
// /components/Breadcrumb.tsx
'use client'
import React, { ReactNode } from 'react'
import { usePathname } from 'next/navigation'
import Link from 'next/link'
type TBreadCrumbProps = {
homeElement: ReactNode,
separator: ReactNode,
containerClasses?: string,
listClasses?: string,
activeClasses?: string,
capitalizeLinks?: boolean
}
const Breadcrumb = ({ homeElement, separator, containerClasses, listClasses, activeClasses, capitalizeLinks }: TBreadCrumbProps) => {
const paths = usePathname() ?? '' // Handle null or undefined paths
const pathNames = paths.split('/').filter(path => path)
return (
<div>
<ul className={containerClasses}>
<li className={listClasses}><Link href={'/'}>{homeElement}</Link></li>
{pathNames.length > 0 && separator}
{
pathNames.map((link, index) => {
let href = `/${pathNames.slice(0, index + 1).join('/')}`
let itemClasses = paths === href ? `${listClasses} ${activeClasses}` : listClasses
let itemLink = capitalizeLinks ? link[0].toUpperCase() + link.slice(1, link.length) : link
return (
<React.Fragment key={index}>
<li className={itemClasses} >
<Link href={href}>{itemLink}</Link>
</li>
{pathNames.length !== index + 1 && separator}
</React.Fragment>
)
})
}
</ul>
</div>
)
}
export default Breadcrumb
In this component, we use the usePathname
hook from next/navigation
to get the current URL path. The path is split into segments, which are then used to generate the breadcrumb links. Each link is created by joining the path segments up to the current segment, allowing for dynamic routing within the breadcrumb.
The component is also designed to be customizable. It accepts several props, such as homeLabel
, separator
, containerClassName
, itemClassName
, activeItemClassName
, and capitalizeItems
, which allow you to control the appearance and behavior of the breadcrumb.
advertisement
Step 2: Integrate the Breadcrumb in the Layout
Next, include the Breadcrumb
component in your layout file, such as app/layout.tsx
, to ensure it's available across all pages of your application.
import Breadcrumb from '@/components/Breadcrumb'
import './globals.css'
import { Inter } from 'next/font/google'
const inter = Inter({ subsets: ['latin'] })
export const metadata = {
title: 'Next.js App',
description: 'Created with Next.js',
}
export default function RootLayout({ children }: { children: React.ReactNode }) {
return (
<html lang="en">
<body className={inter.className}>
<Breadcrumb
homeLabel="Home"
separator={<span> / </span>}
activeItemClassName="text-emerald-600"
containerClassName="flex py-4 bg-gray-100"
itemClassName="mx-2 hover:underline font-medium"
capitalizeItems
/>
{children}
</body>
</html>
)
}
This setup ensures that the breadcrumb component is displayed on every page of your Next.js application. The breadcrumb will automatically update as users navigate through different routes.
Step 3: Apply Styling to the Breadcrumb
You can customize the appearance of the breadcrumb by passing CSS classes to props like containerClasses
, listClasses
, and activeClasses
.
// Example TailwindCSS classes used in Breadcrumb component
// Add these styles directly to the Breadcrumb component via props
<Breadcrumb
homeElement={'Home'}
separator={<span> / </span>}
activeClasses='text-emerald-600'
containerClasses='flex'
listClasses='hover:underline mx-2 font-bold'
capitalizeLinks
/>
Step 4: Test the Breadcrumb Component
Run your Next.js development server and navigate through your application to see the breadcrumb in action. It will dynamically update to reflect the current route.
npm run dev
Open your app in the browser, and you should see the breadcrumb navigation at the top of each page. As you navigate to different routes, the breadcrumb will dynamically reflect your current position within the app's hierarchy.

More JavaScript Articles:
-
Embracing JavaScript: Unveiling the Strengths Beyond Traditional Languages
-
Create a Customizable and Reusable React Hook for Using Local Storage: A Step-by-Step Tutorial
-
Comparing Modern JavaScript Frameworks: React, Angular, and Vue in 2024
advertisement
Support
If you enjoyed this article, please consider making a donation. Your support means a lot to me and allows me to continue providing helpful articles like this one.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Conclusion
You've now created a dynamic breadcrumb component for your Next.js application. This navigation feature not only enhances the user experience but also provides a clear path for users to track their journey through your app. Feel free to extend this component to suit your specific needs and styling preferences.
About the Author
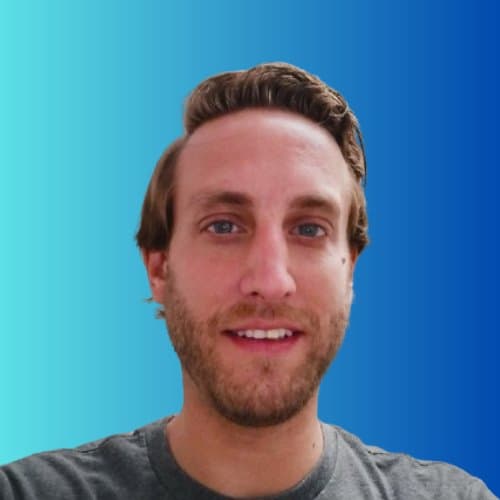
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All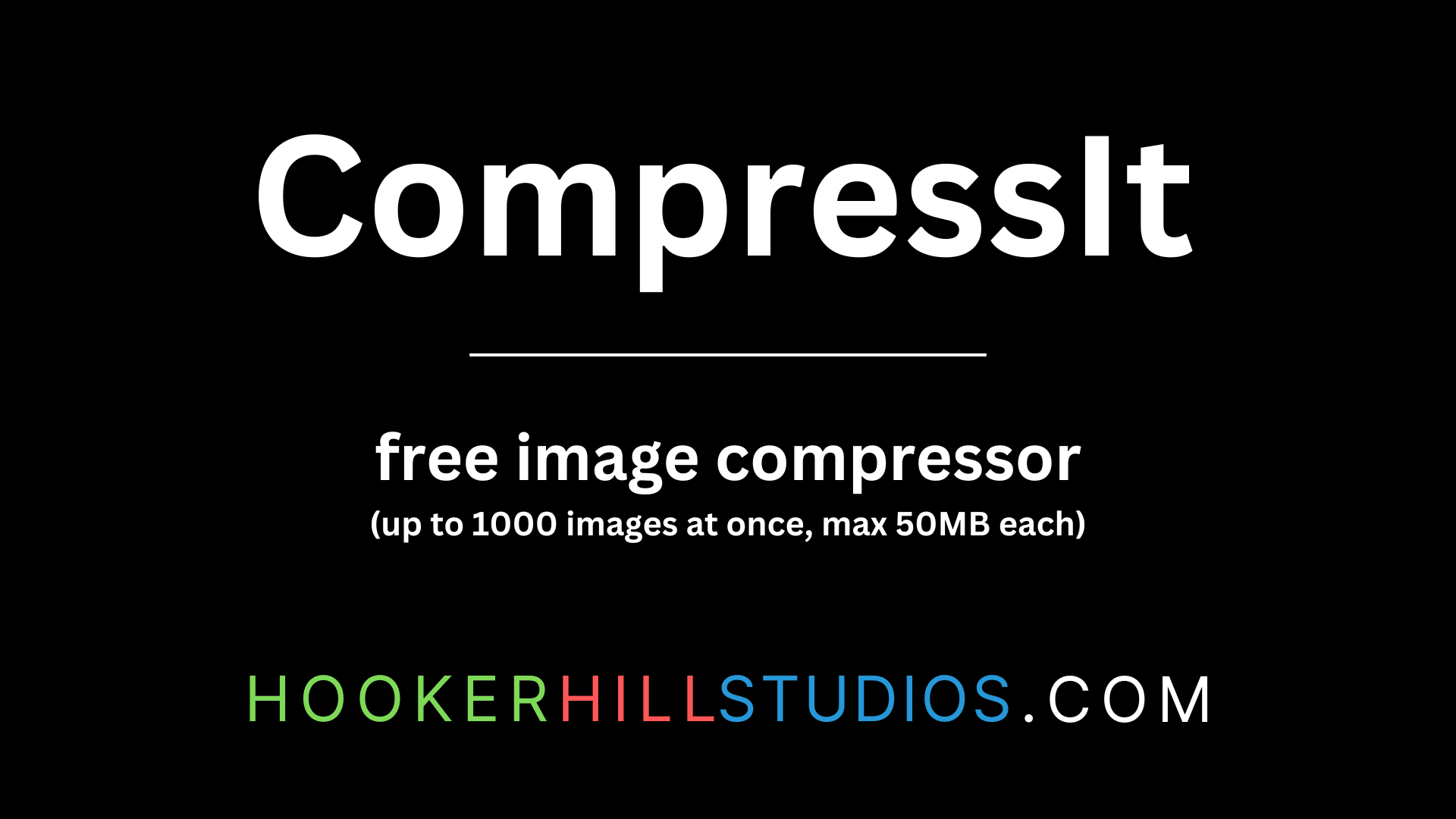
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024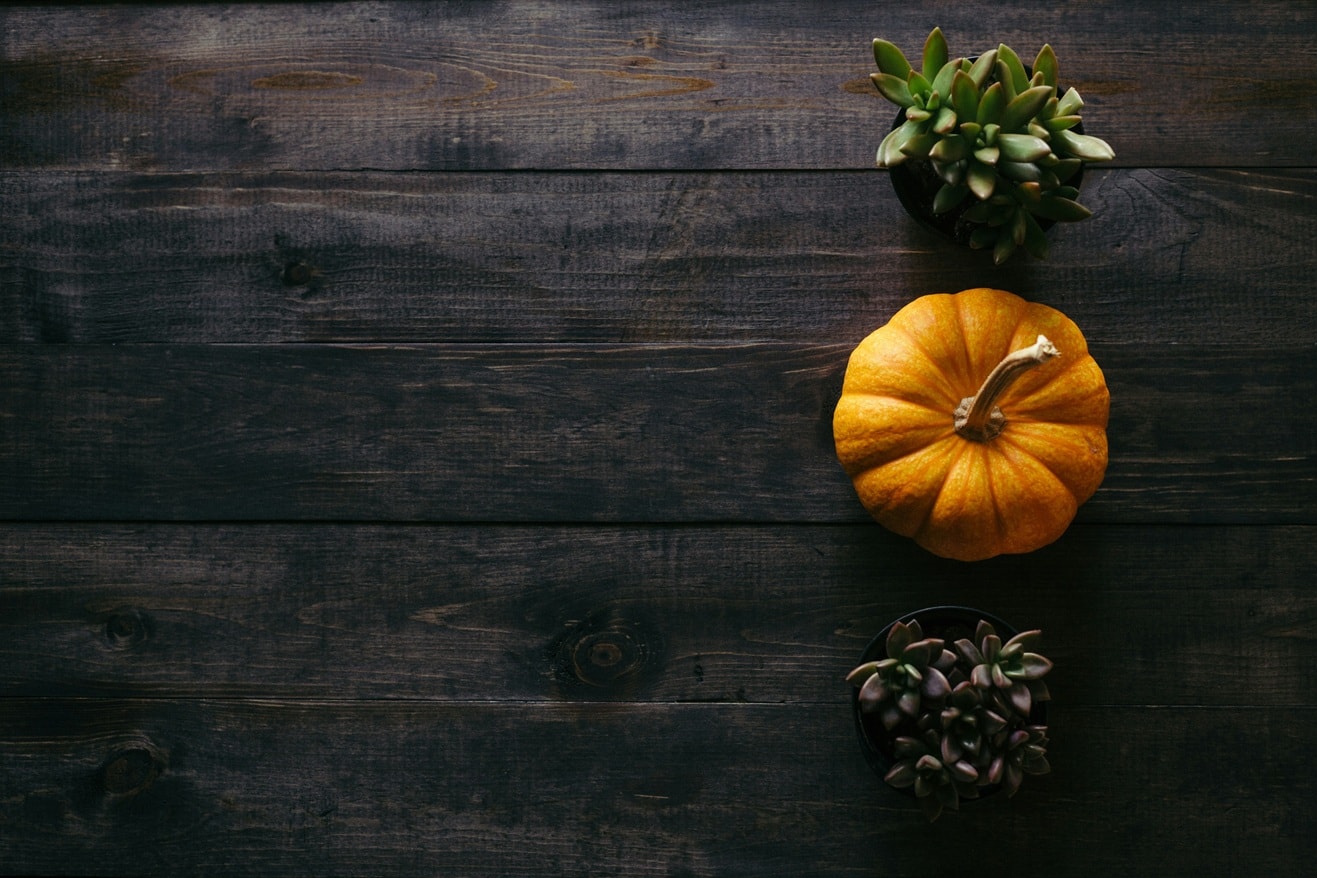
Comments
to join the conversation
Loading comments...