Simplify State Management in React with Zustand: Easy Alternative to Redux
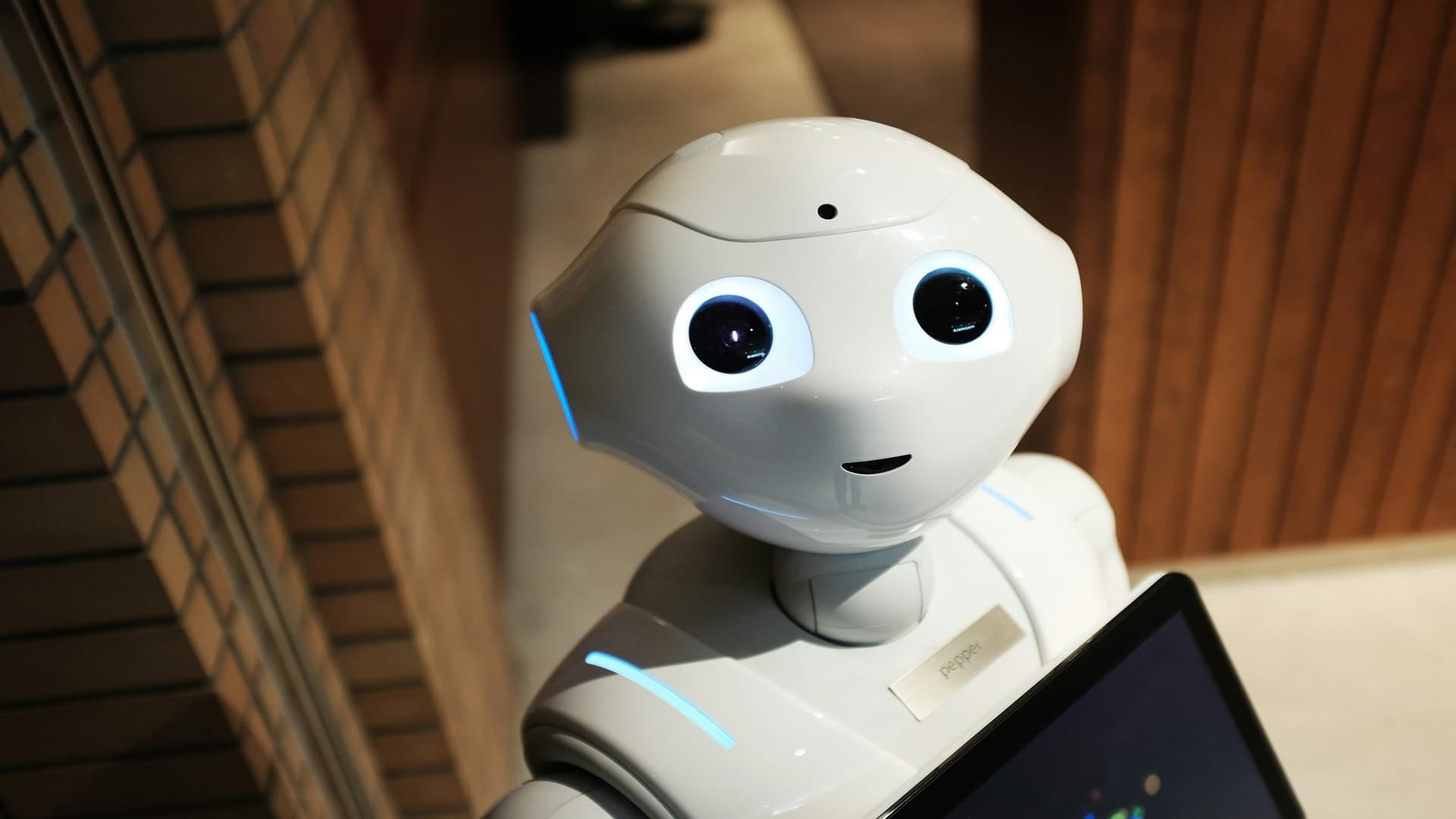
Table Of Contents
- Simplifying State Management in React with Zustand
- What is Zustand?
- Why Use Zustand?
- Key Concepts of Zustand
- Setting Up Zustand in a React project
- 1. Install Zustand
- 2. Create a Store
- 3. Use the Store in a React Component
- 4. Adding Zustand to a React app
- Advanced Concepts: Selectors and Middlewares
- Selectors
- Middleware
- Resources
- FAQ
- What is Zustand?
- How is Zustand different from Redux?
- Do I need to use Zustand for every React project?
- Does Zustand support TypeScript?
- Can I persist state with Zustand?
- Does Zustand work with server-side rendering (SSR)?
- Can I combine Zustand with other state management tools?
- How does Zustand improve performance?
- Donate
- Conclusion
Simplifying State Management in React with Zustand
Managing state in React applications can become complex as apps grow in size. Libraries like Redux provide robust solutions, but they come with a learning curve and additional boilerplate. If you're looking for a simpler, more lightweight alternative, Zustand is a fantastic choice for managing state with minimal setup.
In this post, we'll explore Zustand, break down its key concepts, and walk through an example of how to use it in a React project.
What is Zustand?
Zustand (German for "state") is a small, fast, and flexible state management library for React. It simplifies state management by using hooks and a minimal API that gets the job done without unnecessary complexity. It's lightweight, with minimal boilerplate, and doesn't require a lot of configuration, making it an appealing alternative to Redux.
Why Use Zustand?
- Simplicity: Zustand uses React hooks and a minimal API, making it much easier to learn and integrate.
- Global State: Like Redux, Zustand provides a way to manage global state without prop drilling.
- Flexibility: You can combine Zustand with other libraries or use it for both global and local state.
- Small Size: The library is only 1KB in size, so it won’t bloat your app.
Key Concepts of Zustand
-
Store: Zustand’s store is where all your state lives. Unlike Redux, you don't need actions or reducers to modify the store. Zustand uses a functional approach for both creating and updating state.
-
Set and Get: Zustand’s set method is used to update the state, while the get method is used to access the current state.
-
Selectors: Zustand allows you to select slices of state, meaning you can only re-render components when the relevant state changes, improving performance.
advertisement
Setting Up Zustand in a React project
Let’s walk through a simple example of how to integrate Zustand into a React project to manage global state.
1. Install Zustand
Start by installing the Zustand library:
npm install zustand
2. Create a Store
In Zustand, the store is a simple function. Let's create a store that keeps track of a counter and has methods to increment and decrement it.
// src/store/counterStore.js
import { create } from 'zustand';
const useCounterStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
export default useCounterStore;
In this example:
-
We initialize the
count
state to0
. -
increment
anddecrement
increment and decrement functions use set to update the count value in the store.
3. Use the Store in a React Component
Now, let’s create a simple counter component that uses the Zustand store.
// src/components/Counter.js
import React from 'react';
import useCounterStore from '../store/counterStore';
const Counter = () => {
const { count, increment, decrement } = useCounterStore();
return (
<div className="flex flex-col items-center space-y-4">
<h1 className="text-3xl font-bold">Count: {count}</h1>
<div className="flex space-x-4">
<button
className="px-4 py-2 bg-green-500 text-white rounded"
onClick={increment}
>
Increment
</button>
<button
className="px-4 py-2 bg-red-500 text-white rounded"
onClick={decrement}
>
Decrement
</button>
</div>
</div>
);
};
export default Counter;
Here’s how it works:
-
We use
useCounterStore()
to access the count state and theincrement
anddecrement
increment and decrement functions. -
Each button triggers the corresponding function to update the state.
4. Adding Zustand to a React app
Finally, import and use the Counter
component in your main application file:
// src/App.js
import React from 'react';
import Counter from './components/Counter';
function App() {
return (
<div className="App">
<Counter />
</div>
);
}
export default App;
Now, running your app will display a counter with buttons to increment or decrement the value.
Advanced Concepts: Selectors and Middlewares
Selectors
Zustand allows you to select only the state you need, avoiding unnecessary re-renders. You can create selectors when calling useCounterStore()
to optimize performance:
const count = useCounterStore((state) => state.count);
const increment = useCounterStore((state) => state.increment);
With this approach, the component will only re-render if count
changes.
Middleware
Zustand also supports middlewares such as persistence (e.g., saving state in localStorage
) and devtools for debugging. Here's how you can integrate the persist
middleware:
import { create } from 'zustand';
import { persist } from 'zustand/middleware';
const useCounterStore = create(
persist(
(set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}),
{
name: 'counter-storage', // name for localStorage
}
)
);
advertisement
Resources
Here are some helpful resources to learn more about Zustand and state management in React:
-
Zustand Documentation: The official documentation provides everything you need to get started with Zustand, including examples and advanced features.
https://docs.pmnd.rs/zustand/getting-started/introduction -
React Documentation: Learn more about React’s state management and hooks. Zustand integrates seamlessly with React’s core features.
https://react.dev/learn -
Zustand GitHub Repository: Check out the source code of Zustand and contribute to the library or explore issues and pull requests.
https://github.com/pmndrs/zustand -
Zustand with Middleware: Learn more about using middleware like persist and devtools with Zustand to enhance state management in your app.
https://docs.pmnd.rs/zustand/integrations/persisting-store-data
FAQ
What is Zustand?
Zustand is a lightweight, simple, and fast state management library for React. It allows you to manage both global and local state using hooks without the need for actions, reducers, or boilerplate code like in Redux.
How is Zustand different from Redux?
While both Zustand and Redux are state management libraries, Zustand is much simpler and has a smaller API surface. Redux requires setting up reducers, actions, and middleware, while Zustand focuses on minimal setup with direct state updates via a hook-based API.
Do I need to use Zustand for every React project?
Not necessarily. Zustand is best suited for medium-to-large applications that need global state management. For smaller apps, React's built-in useState
and useReducer
hooks might suffice.
Does Zustand support TypeScript?
Yes, Zustand has built-in TypeScript support, allowing you to define your state and actions with full type safety. You can easily use TypeScript types and interfaces in your store to ensure type checking across your app.
Can I persist state with Zustand?
Yes, Zustand has middleware that allows you to persist state to localStorage
or sessionStorage
. You can use the persist
middleware to save and restore your state across page reloads.
Does Zustand work with server-side rendering (SSR)?
Yes, Zustand works with Next.js and other server-side rendering (SSR) frameworks. You can prefetch state on the server and hydrate it on the client.
Can I combine Zustand with other state management tools?
Yes, Zustand is flexible and can be combined with other libraries. You can use it alongside Context API, React’s built-in hooks, or even with Redux for different parts of your application’s state.
How does Zustand improve performance?
Zustand allows you to selectively subscribe to parts of the state. Components only re-render when the specific slice of state they depend on changes, improving overall performance by preventing unnecessary renders.
advertisement
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Conclusion
Zustand is a powerful yet lightweight state management library that simplifies the way you manage state in React applications. Its minimal API, combined with React hooks, makes it an excellent choice for developers who want to avoid the complexity of Redux while still managing global state efficiently.
By following this guide, you now have a basic understanding of how Zustand works and how you can implement it in your React projects. Try it out in your next project and experience the simplicity!
About the Author
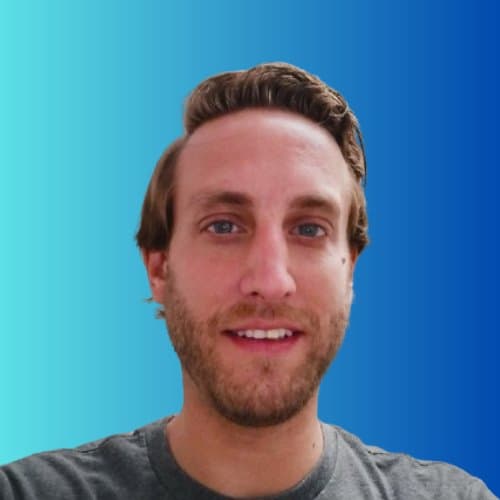
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All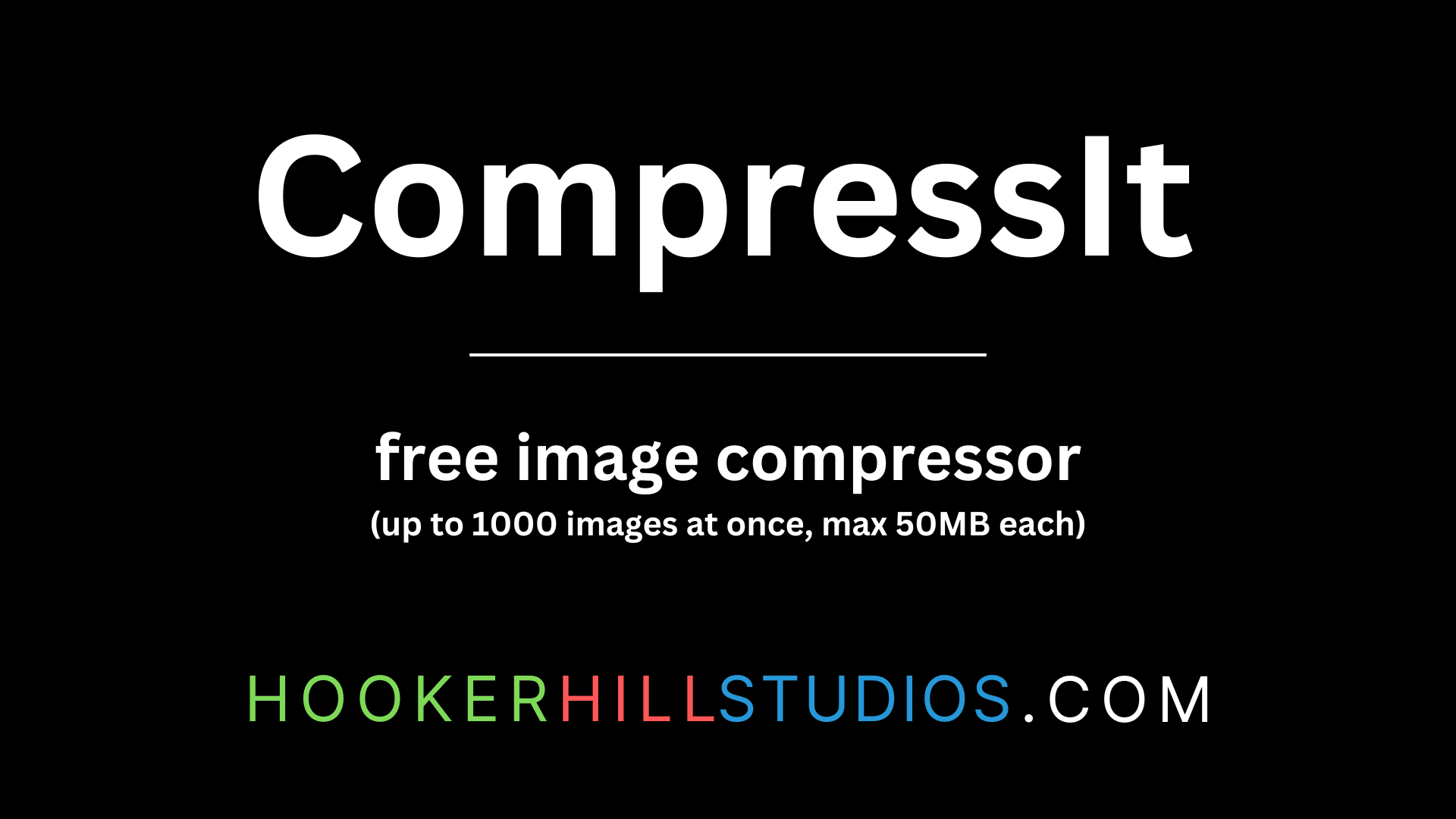
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024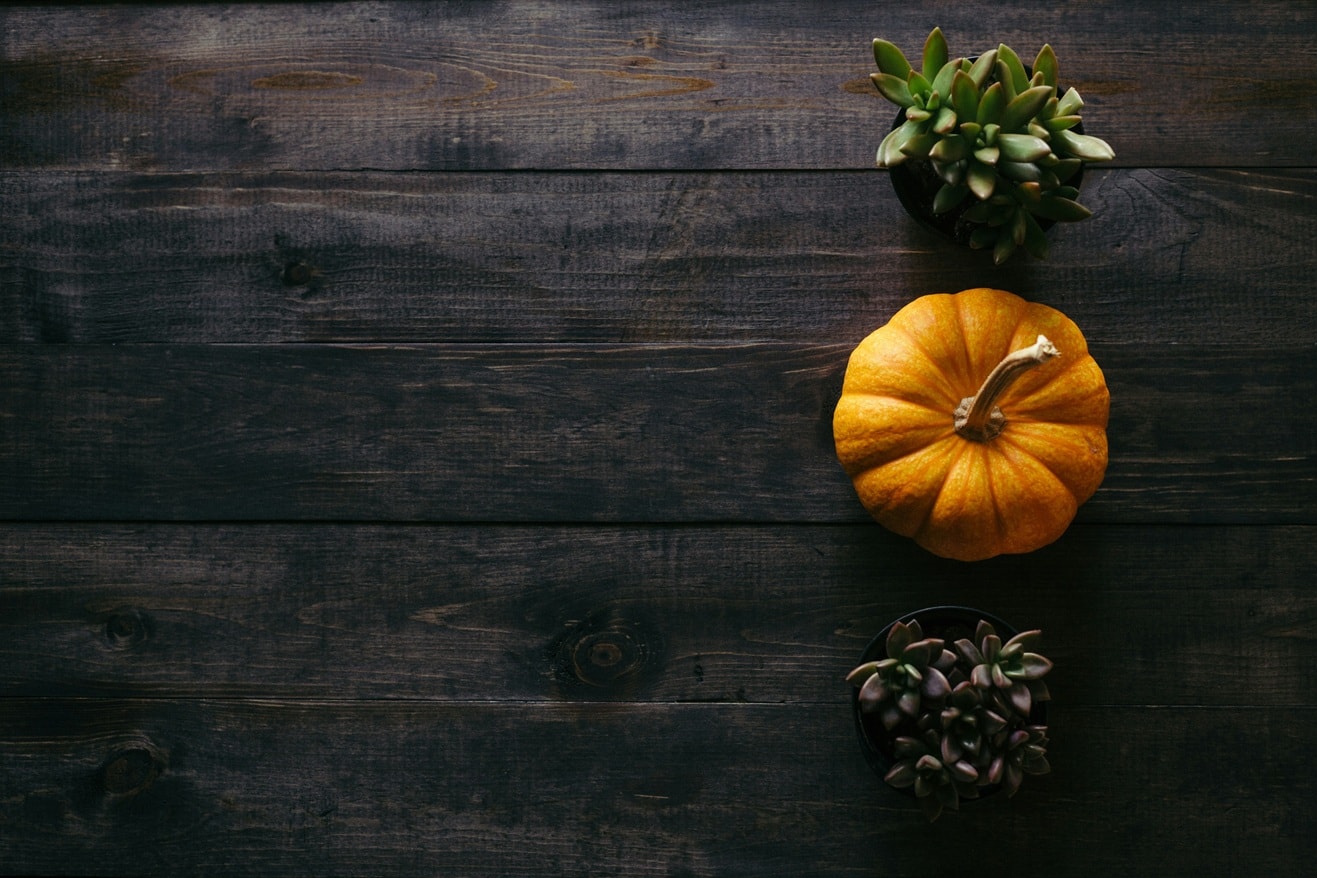
Comments
to join the conversation
Loading comments...