How to Set Up Firebase Authentication in Next.js: Easy Guide
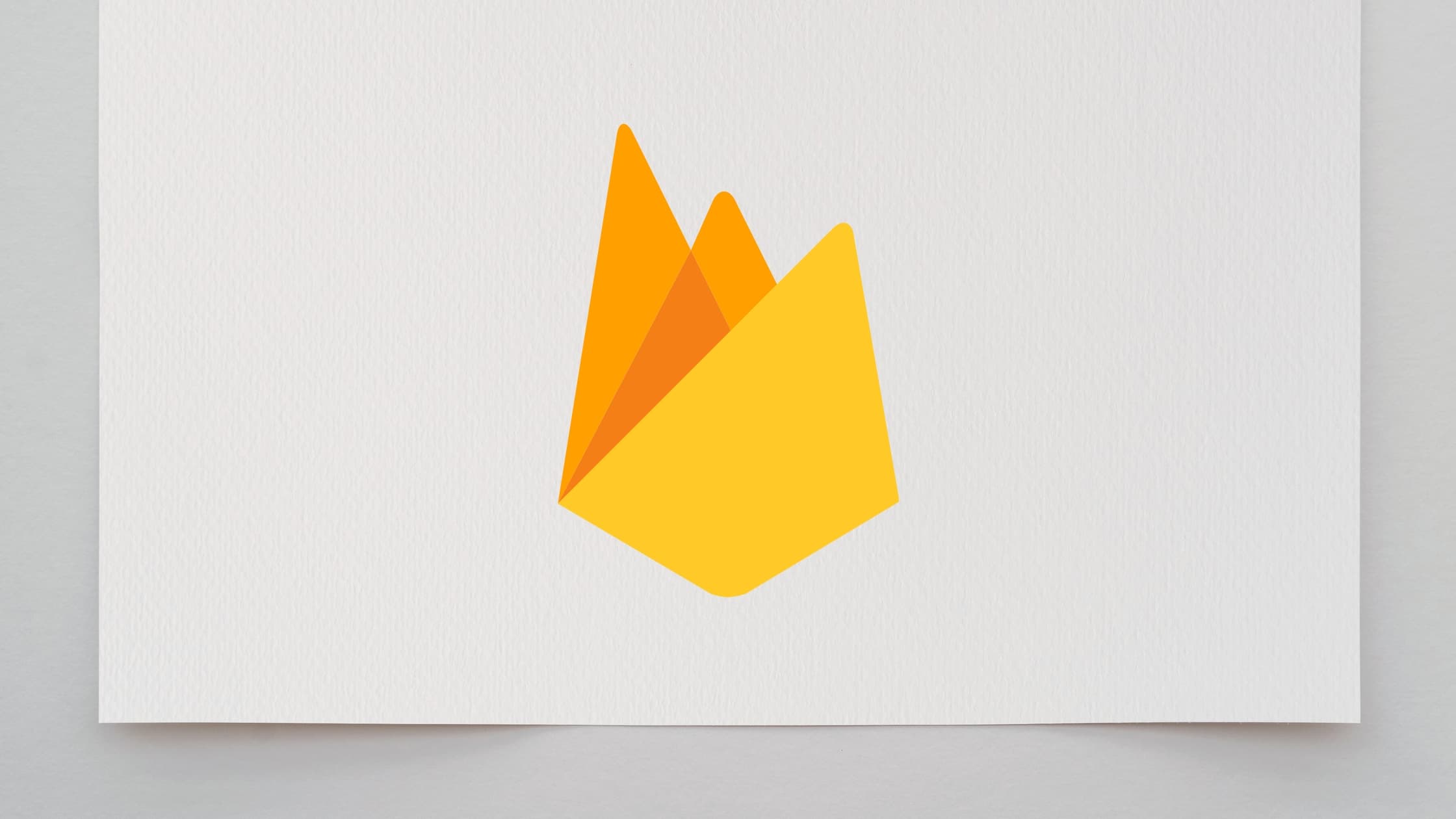
Table Of Contents
- Setting Up Firebase Authentication in a Next.js Application
- 1. Introduction
- 2. Prerequisites
- 3. Setting Up Firebase
- 4. Creating the Authentication Context
- 5. Integrating the AuthProvider in Next.js
- 6. Creating the Authentication Components
- 7. Handling Protected Routes
- 8. Deploying to Vercel with Environment Variables
- .env
- 9. FAQs
- 10. Additional Resources
- Donate
- Conclusion
Setting Up Firebase Authentication in a Next.js Application
1. Introduction
In this guide, we will walk you through the process of integrating Firebase Authentication into a Next.js application. You will learn how to set up Firebase, create an authentication context, protect routes, and deploy your application with secure environment variables.
2. Prerequisites
Before we begin, ensure you have the following:
- Basic knowledge of Next.js.
- A Firebase project set up in the Firebase console.
- Node.js and npm installed on your local machine.
3. Setting Up Firebase
First, you need to install the necessary Firebase packages and initialize Firebase in your Next.js project.
Install Firebase packages:
npm install firebase react-firebase-hooks
Initialize Firebase in firebase.js
:
// src/firebase.js
import { initializeApp } from "firebase/app";
import { getAuth } from "firebase/auth";
const firebaseConfig = {
apiKey: process.env.NEXT_PUBLIC_FIREBASE_API_KEY,
authDomain: process.env.NEXT_PUBLIC_FIREBASE_AUTH_DOMAIN,
projectId: process.env.NEXT_PUBLIC_FIREBASE_PROJECT_ID,
storageBucket: process.env.NEXT_PUBLIC_FIREBASE_STORAGE_BUCKET,
messagingSenderId: process.env.NEXT_PUBLIC_FIREBASE_MESSAGING_SENDER_ID,
appId: process.env.NEXT_PUBLIC_FIREBASE_APP_ID,
};
const app = initializeApp(firebaseConfig);
export const auth = getAuth(app);
advertisement
4. Creating the Authentication Context
Next, we'll create an AuthContext
to manage the authentication state across your application.
Create the AuthContext
:
// src/context/AuthContext.js
import { createContext, useContext, useEffect, useState } from "react";
import { onAuthStateChanged } from "firebase/auth";
import { auth } from "../firebase";
const AuthContext = createContext();
export const AuthProvider = ({ children }) => {
const [user, setUser] = useState(null);
useEffect(() => {
const unsubscribe = onAuthStateChanged(auth, (user) => {
setUser(user);
});
return () => unsubscribe();
}, []);
return <AuthContext.Provider value={{ user }}>{children}</AuthContext.Provider>;
};
export const useAuth = () => useContext(AuthContext);
5. Integrating the AuthProvider in Next.js
To make the authentication context available throughout your application, wrap your component tree with the AuthProvider
.
Wrap your application with the AuthProvider
in the layout file:
// src/app/layout.js
import { AuthProvider } from "../context/AuthContext";
function MyApp({ Component, pageProps }) {
return (
<AuthProvider>
<Component {...pageProps} />
</AuthProvider>
);
}
export default MyApp;
6. Creating the Authentication Components
Now, let's build the components to handle user sign-up, login, and logout. These components will use the Firebase Authentication methods to manage user sessions.
Create the AuthForm
component:
// src/components/AuthForm.js
import { useState } from "react";
import { auth } from "../firebase";
import { createUserWithEmailAndPassword, signInWithEmailAndPassword } from "firebase/auth";
const AuthForm = () => {
const [email, setEmail] = useState("");
const [password, setPassword] = useState("");
const [isSignUp, setIsSignUp] = useState(true);
const handleSubmit = async (e) => {
e.preventDefault();
if (isSignUp) {
await createUserWithEmailAndPassword(auth, email, password);
} else {
await signInWithEmailAndPassword(auth, email, password);
}
};
return (
<form onSubmit={handleSubmit}>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
placeholder="Email"
required
/>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
placeholder="Password"
required
/>
<button type="submit">{isSignUp ? "Sign Up" : "Login"}</button>
<button type="button" onClick={() => setIsSignUp(!isSignUp)}>
{isSignUp ? "Already have an account? Login" : "New user? Sign Up"}
</button>
</form>
);
};
export default AuthForm;
advertisement
7. Handling Protected Routes
To ensure certain pages are only accessible to authenticated users, we'll create a ProtectedRoute
component.
Create the ProtectedRoute
component:
// src/app/page.js
import { useAuth } from "../context/AuthContext";
import AuthForm from "../components/AuthForm";
const Home = () => {
const { user } = useAuth();
return (
<div>
{user ? <h1>Welcome, {user.email}</h1> : <AuthForm />}
</div>
);
};
export default Home;
Apply the ProtectedRoute
to a page:
// src/components/ProtectedRoute.js
import { useAuth } from "../context/AuthContext";
import { useRouter } from "next/router";
import { useEffect } from "react";
const ProtectedRoute = ({ children }) => {
const { user } = useAuth();
const router = useRouter();
useEffect(() => {
if (!user) {
router.push("/login");
}
}, [router, user]);
return user ? children : null;
};
export default ProtectedRoute;
8. Deploying to Vercel with Environment Variables
When deploying to Vercel, it's crucial to secure your Firebase configuration using environment variables.
Set up environment variables in Vercel:
# .env
NEXT_PUBLIC_FIREBASE_API_KEY=YOUR_API_KEY
NEXT_PUBLIC_FIREBASE_AUTH_DOMAIN=YOUR_AUTH_DOMAIN
NEXT_PUBLIC_FIREBASE_PROJECT_ID=YOUR_PROJECT_ID
NEXT_PUBLIC_FIREBASE_STORAGE_BUCKET=YOUR_STORAGE_BUCKET
NEXT_PUBLIC_FIREBASE_MESSAGING_SENDER_ID=YOUR_MESSAGING_SENDER_ID
NEXT_PUBLIC_FIREBASE_APP_ID=YOUR_APP_ID
Deploy to Vercel:
Install vercel
and vercel deploy
.
npm install vercel
Deploy to Vercel:
Deploy your application to Vercel.
vercel deploy --prod
9. FAQs
Q1: How do I handle authentication errors?
A1: You can add error handling in the login
and logout
methods within the AuthContext
by catching exceptions and updating the UI accordingly.
Q2: Can I use Firebase Authentication with server-side rendering in Next.js?
A2: Yes, you can, but it requires additional setup. Typically, you would use Firebase Admin SDK on the server side for handling authentication.
10. Additional Resources
- Next.js Documentation
- Firebase Documentation
- Deploying Next.js on Vercel
- Firebase Authentication in React
advertisement
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Conclusion
Congratulations! You have successfully created and deployed a Next.js app with Firebase Authentication. You can now access your app via the URL provided by Firebase. Feel free to leave a comment below with your thoughts and suggestions.
About the Author
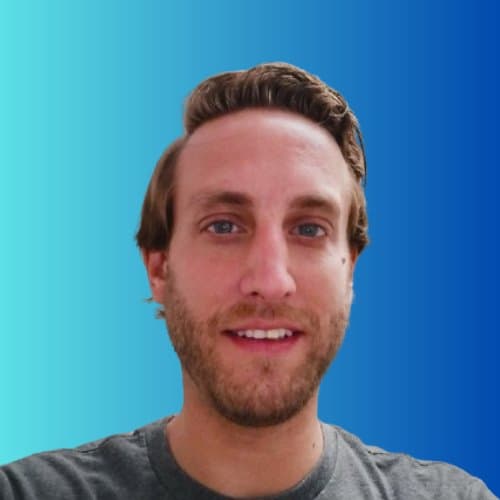
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All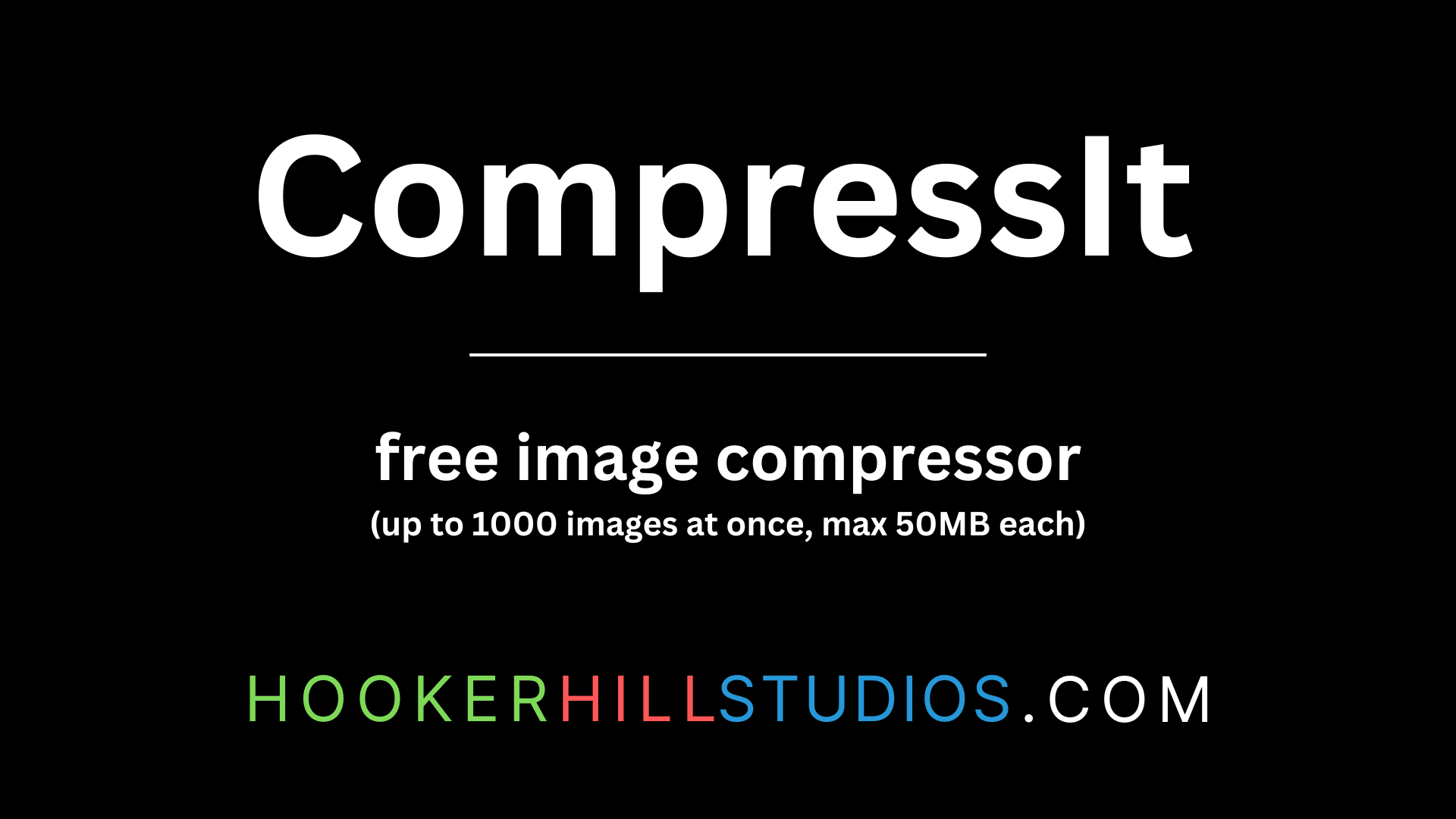
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024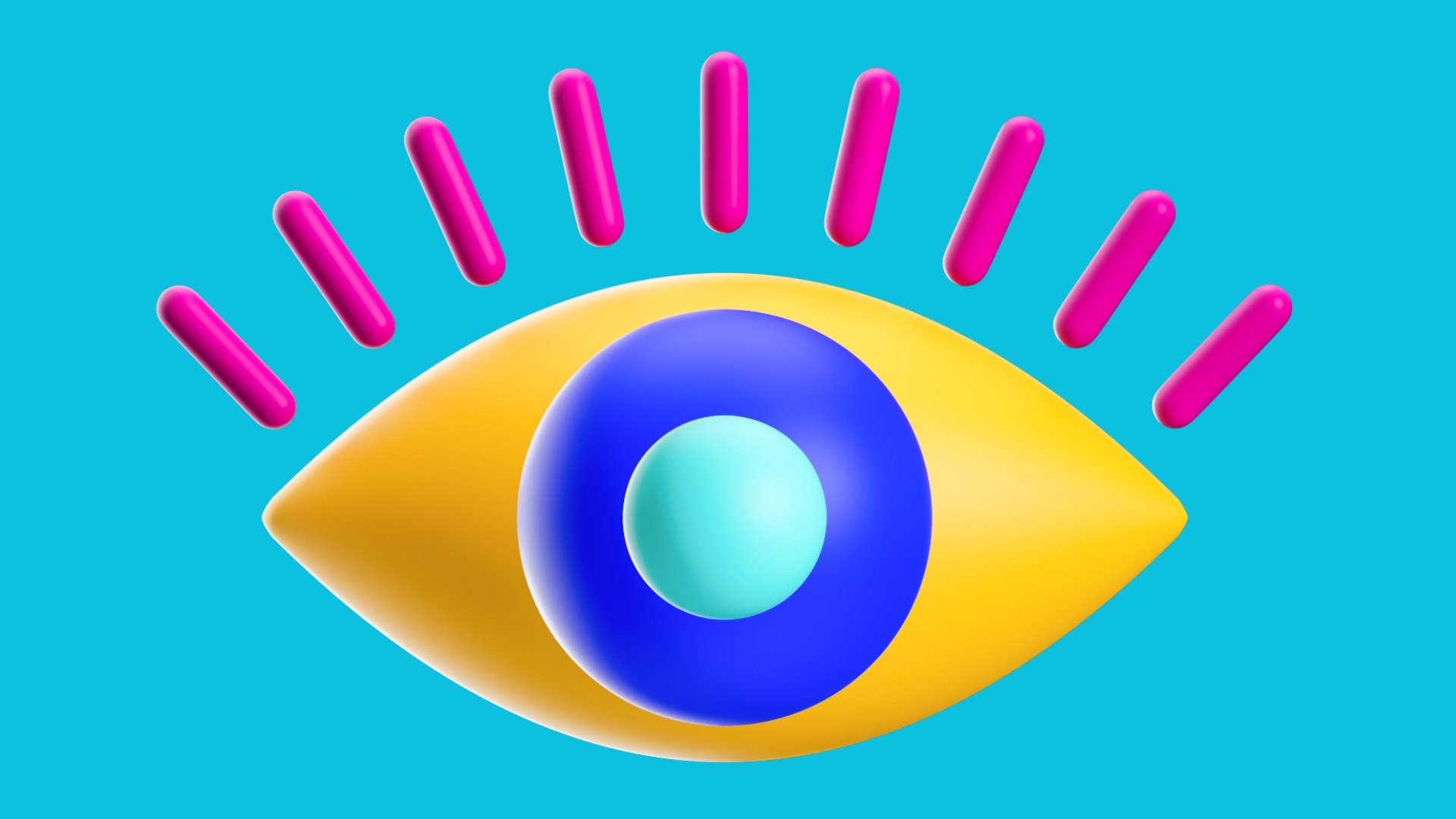
Effortless Interactivity with HTML's details and summary Tags
November 22, 2024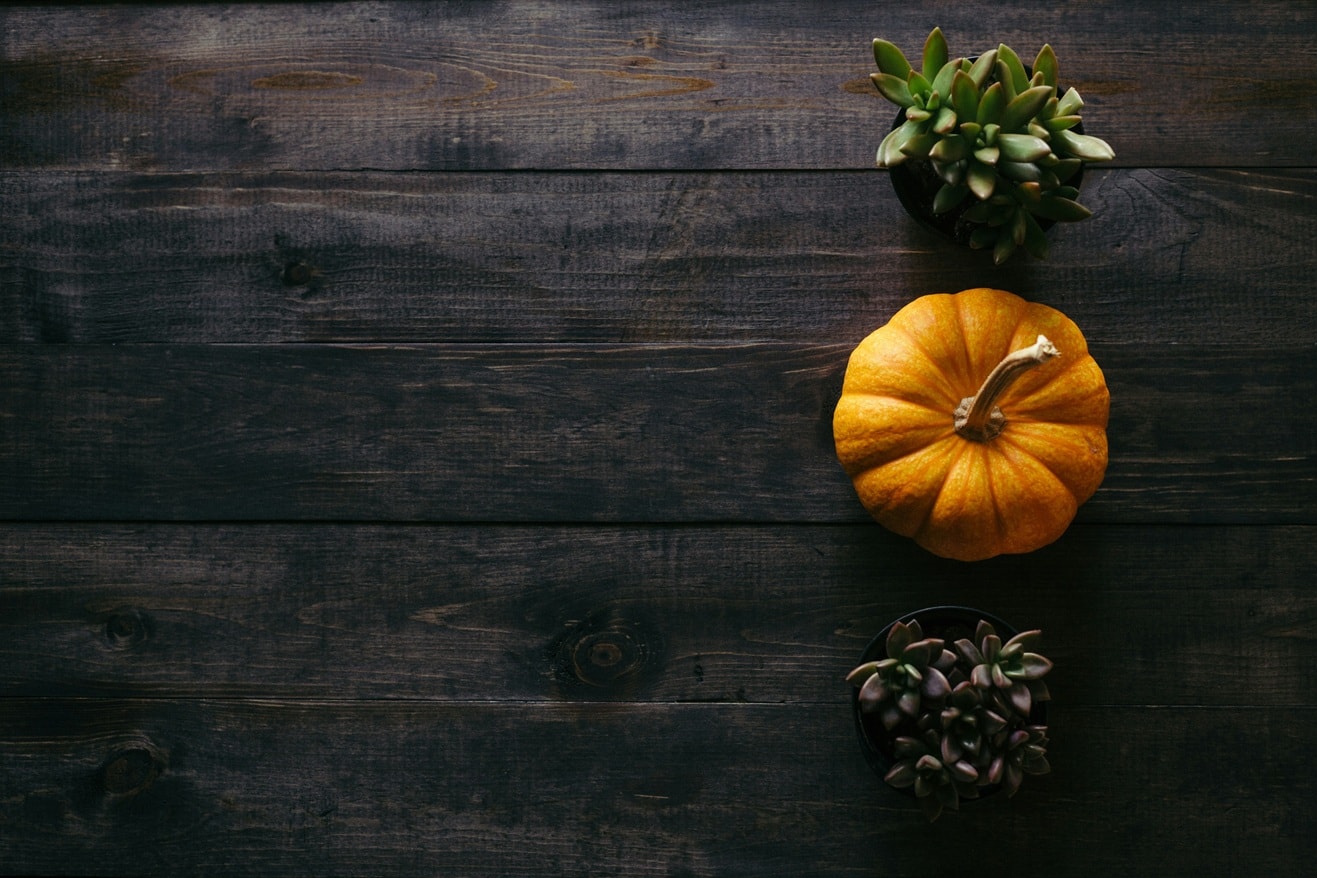
Comments
to join the conversation
Loading comments...