Embracing JavaScript: Unveiling the Strengths Beyond Traditional Languages
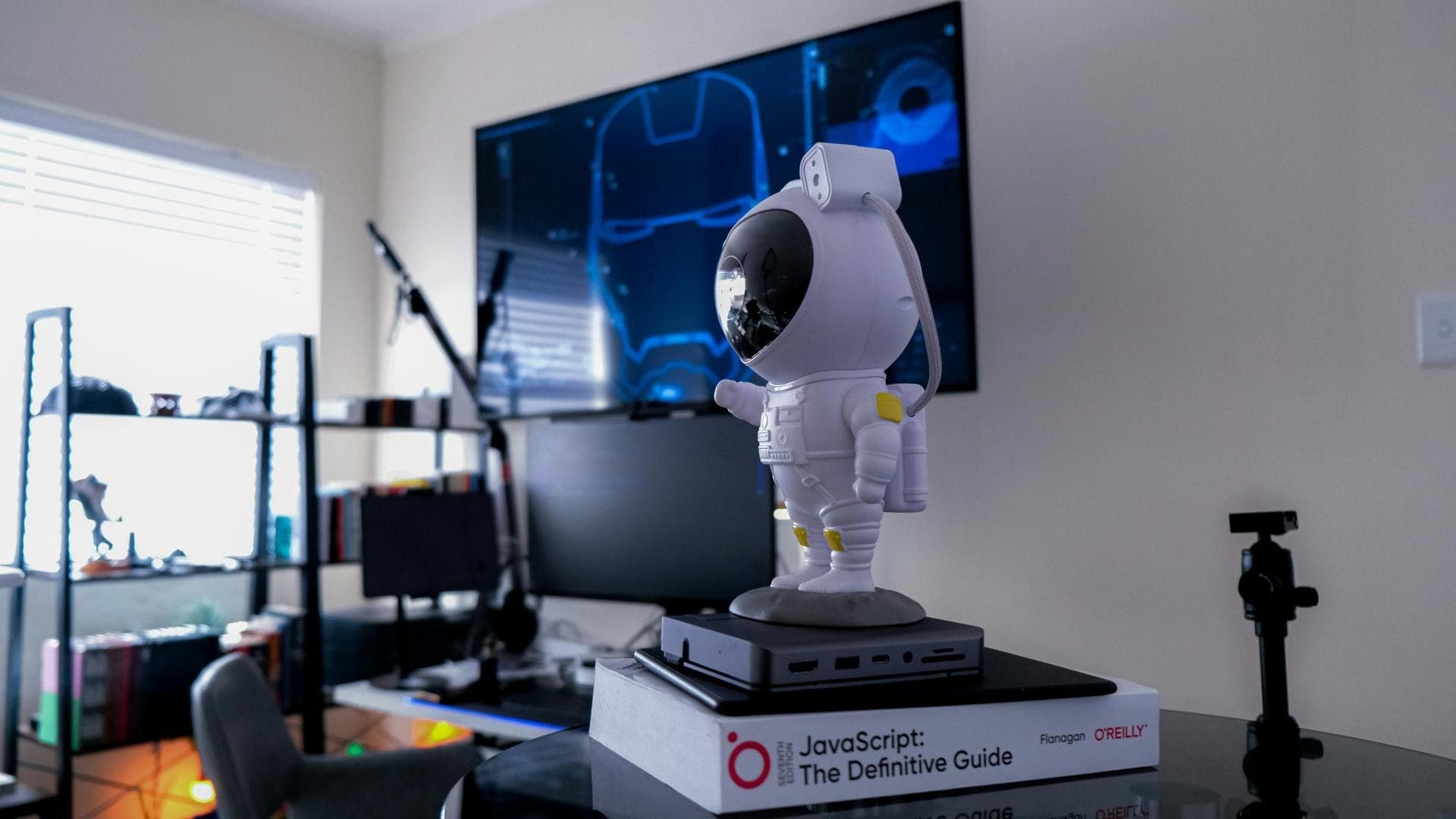
Table Of Contents
Embracing JavaScript: Unveiling the Strengths Beyond Traditional Languages
JavaScript often gets a bad rap when compared to languages like C, C++, Java, or C#. Critics point out its dynamic typing, lack of traditional class-based inheritance, and single-threaded nature as shortcomings. However, these features were intentional design choices made to suit JavaScript's primary environment—the web. Understanding why JavaScript is the way it is reveals its unique advantages, especially in web development.
Historical Context
JavaScript was created in 1995 by Brendan Eich at Netscape. It was developed in just 10 days as a lightweight scripting language for adding interactivity to web pages. The language was designed to complement Java but not replace it, targeting non-professional programmers like web designers. This context is crucial for understanding its design choices:
-
Web Focused: JavaScript was made to run in web browsers, interacting with the DOM (Document Object Model) to create dynamic web pages.
-
Ease of Use: It needed to be easy to learn and use by non-professional programmers.
-
Rapid Development: Given its initial rapid development, simplicity and flexibility were prioritized over strictness and performance.
Design Choices and Benefits
1. Dynamic typing
JavaScript:
-
Variables in JavaScript can hold any type of value and can change types at runtime.
-
This allows for rapid development and easier experimentation.
Example:
let value = 42; // Initially a number
value = "Hello"; // Now a string
value = true; // Now a boolean
Benefits:
-
Flexibility: Enables quick prototyping and dynamic changes.
-
Less Boilerplate: No need to declare variable types explicitly.
Comparison with C/Java:
- C and Java are statically typed, meaning variable types are fixed at compile time.
Example in Java:
int value = 42;
value = "Hello"; // Compile-time error
JavaScript was developed in just 10 days by Brendan Eich in 1995, highlighting its initial goal of simplicity and rapid development.
advertisement
2. First-Class Funtions
JavaScript:
-
Functions are first-class citizens; they can be assigned to variables, passed as arguments, and returned from other functions.
-
This supports functional programming paradigms and callbacks.
Example:
function greet(name) {
return `Hello, ${name}!`;
}
const sayHello = greet;
console.log(sayHello("Alice")); // "Hello, Alice!"
function execute(func, value) {
return func(value);
}
console.log(execute(greet, "Bob")); // "Hello, Bob!"
Benefits:
-
Higher-Order Functions: Enables powerful abstractions and reusable code.
-
Callbacks and Async Operations: Essential for non-blocking I/O operations.
Comparison with C/Java:
-
In C, functions are not first-class, although function pointers exist.
-
In Java, functions are not first-class, but similar functionality is achieved with interfaces and lambda expressions.
Example in Java:
import java.util.function.Function;
Function<String, String> greet = name -> "Hello, " + name + "!";
System.out.println(greet.apply("Alice")); // "Hello, Alice!"
JavaScript is the only language that can run natively in all web browsers, making it the backbone of web interactivity and front-end development.
3. Event-Driven, Non-Blocking I/O
JavaScript:
-
JavaScript’s single-threaded, event-driven architecture with non-blocking I/O is suited for handling asynchronous operations efficiently.
-
This is crucial for web applications needing to remain responsive.
Example:
console.log("Start");
setTimeout(() => {
console.log("This runs after 1 second");
}, 1000);
console.log("End");
Benefits:
-
Responsiveness: Keeps UI responsive and handles many I/O operations efficiently.
-
Scalability: Suitable for high-concurrency scenarios like web servers.
Comparison with C/Java:
-
C often uses multi-threading for concurrent operations, which is more complex to manage.
-
Java also uses multi-threading and has more overhead with thread management.
Example in Java:
System.out.println("Start");
new Thread(() -> {
try {
Thread.sleep(1000);
System.out.println("This runs after 1 second");
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
System.out.println("End");
First-class functions and closures in JavaScript enable powerful patterns like callbacks and asynchronous programming, which are fundamental for modern web applications.
advertisement
4. Prototypal Inheritance
JavaScript:
-
Uses prototypes for inheritance instead of classical inheritance.
-
Objects can directly inherit from other objects.
Example:
const person = {
greet() {
console.log("Hello!");
}
};
const alice = Object.create(person);
alice.greet(); // "Hello!"
Benefits:
-
Flexibility: Objects can be extended or modified dynamically.
-
Simplicity: Simpler inheritance model for many use cases.
Comparison with C/Java:
-
C++ and Java use classical inheritance with classes and hierarchies.
-
More rigid and complex compared to JavaScript’s dynamic nature.
Example in Java:
class Person {
void greet() {
System.out.println("Hello!");
}
}
class Alice extends Person {
}
Alice alice = new Alice();
alice.greet(); // "Hello!"
Node.js extends JavaScript to the server side, allowing developers to use a single language for both client-side and server-side development, streamlining full-stack development processes.
5. Ubiquity and Versatility
JavaScript:
-
Runs natively in all web browsers, making it the de facto language for web development.
-
With Node.js, it can also be used for server-side development.
Example:
// Client-side JavaScript
document.getElementById('myButton').addEventListener('click', () => {
alert('Button clicked!');
});
// Server-side JavaScript with Node.js
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, () => {
console.log('Server running at http://127.0.0.1:3000/');
});
Benefits:
-
Single Language: Enables full-stack development with a single language.
-
Community and Ecosystem: Rich ecosystem of libraries, tools, and frameworks.
Comparison with C/Java:
-
C and Java are traditionally used for different domains (system programming and enterprise applications, respectively).
-
They do not run natively in web browsers and require separate languages or technologies for web front-end development.
Prototypal inheritance in JavaScript allows for more dynamic and flexible object relationships compared to classical inheritance, making it easier to create complex data structures and behaviors on the fly.
advertisement
FAQ
Why is JavaScript dynamically typed?
JavaScript is dynamically typed to offer greater flexibility and speed in development. This allows for quick prototyping and reduces the need for verbose type declarations, which is ideal for the rapid, iterative nature of web development.
What are first-class functions in JavaScript, and why are they important?
First-class functions in JavaScript can be assigned to variables, passed as arguments, and returned from other functions. This is crucial for functional programming, enabling powerful abstractions and making asynchronous programming more manageable.
How does JavaScript handle asynchronous operations?
JavaScript uses an event-driven, non-blocking I/O model. This approach allows it to handle multiple asynchronous operations efficiently without blocking the main execution thread, which is essential for maintaining responsive web applications.
What is prototypal inheritance, and how does it differ from classical inheritance?
Prototypal inheritance in JavaScript allows objects to inherit directly from other objects, providing a more flexible and dynamic inheritance model compared to the rigid class-based inheritance in languages like Java and C++.
Why is JavaScript considered ubiquitous and versatile?
JavaScript is the only language that runs natively in all web browsers, making it indispensable for web development. With the advent of Node.js, it has extended its reach to server-side development, enabling full-stack development with a single language.
More JavaScript Articles:
-
Create a Customizable and Reusable React Hook for Using Local Storage: A Step-by-Step Tutorial
-
Comparing Modern JavaScript Frameworks: React, Angular, and Vue in 2024
Conclusion
JavaScript's design choices, while often misunderstood or undervalued by those familiar with more traditional languages like C and Java, are what make it uniquely powerful for web development. Its dynamic typing, first-class functions, event-driven non-blocking I/O, and prototypal inheritance are not shortcomings but strengths tailored for its primary environment—the web. These features enable rapid development, high responsiveness, and scalability, which are critical for creating modern, interactive web applications.
As we continue to witness the evolution of web technologies and the growing importance of full-stack development, JavaScript's role remains indispensable. By understanding and embracing the reasons behind its design, developers can leverage JavaScript's capabilities to their fullest potential, crafting dynamic and efficient applications that meet the demands of today's digital landscape. Rather than viewing JavaScript through the lens of traditional programming paradigms, recognizing its unique advantages allows for a more nuanced appreciation of why it has become the backbone of the web.
advertisement
About the Author
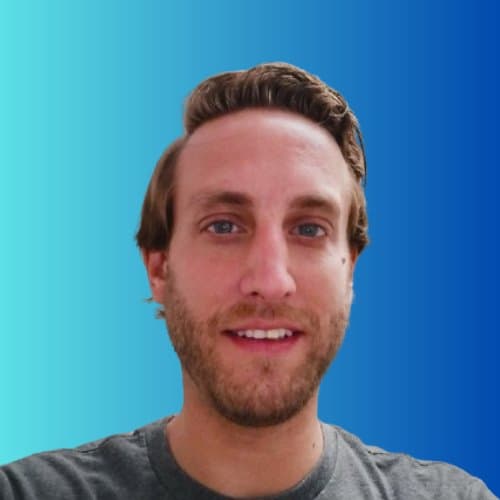
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All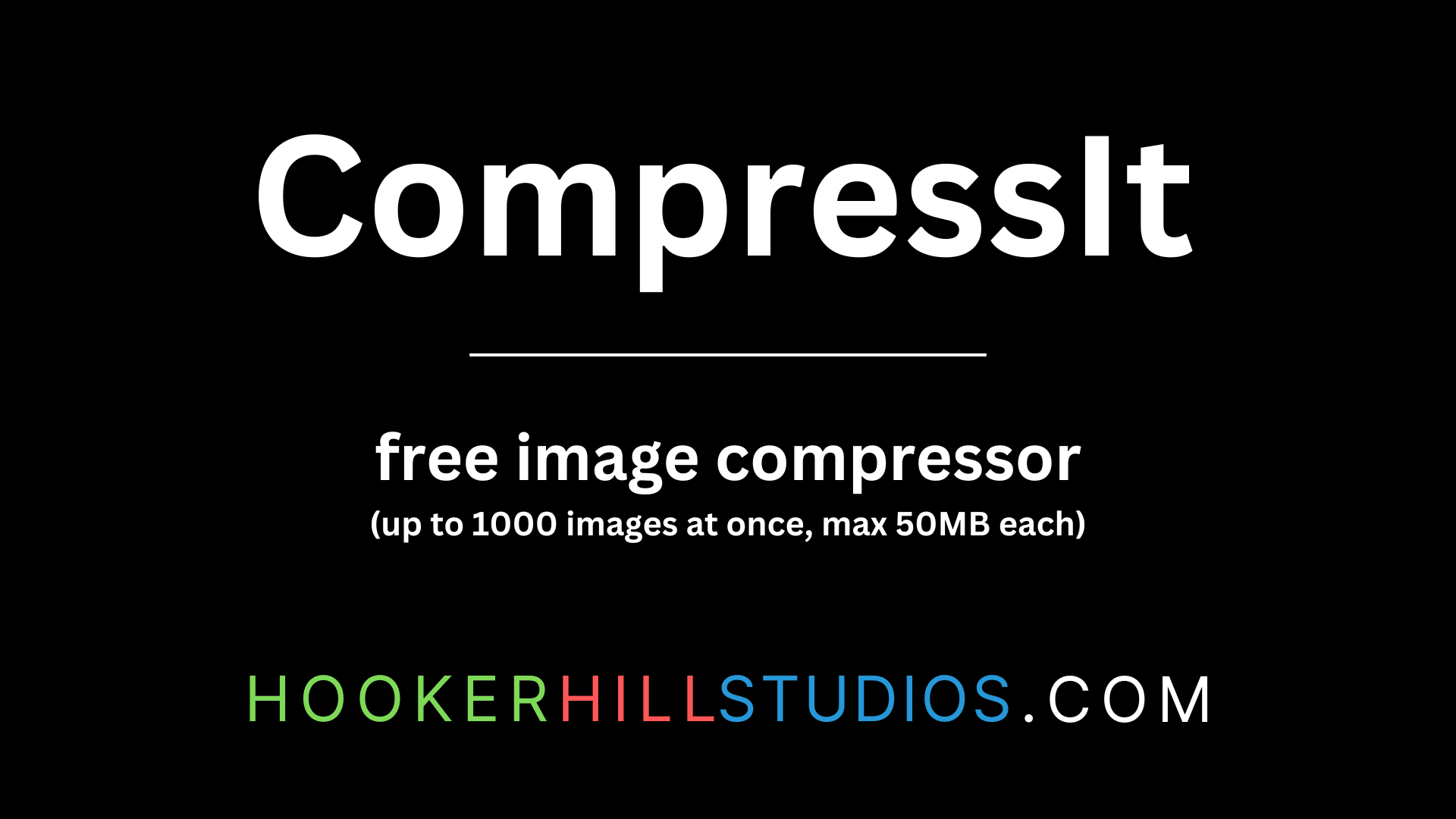
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024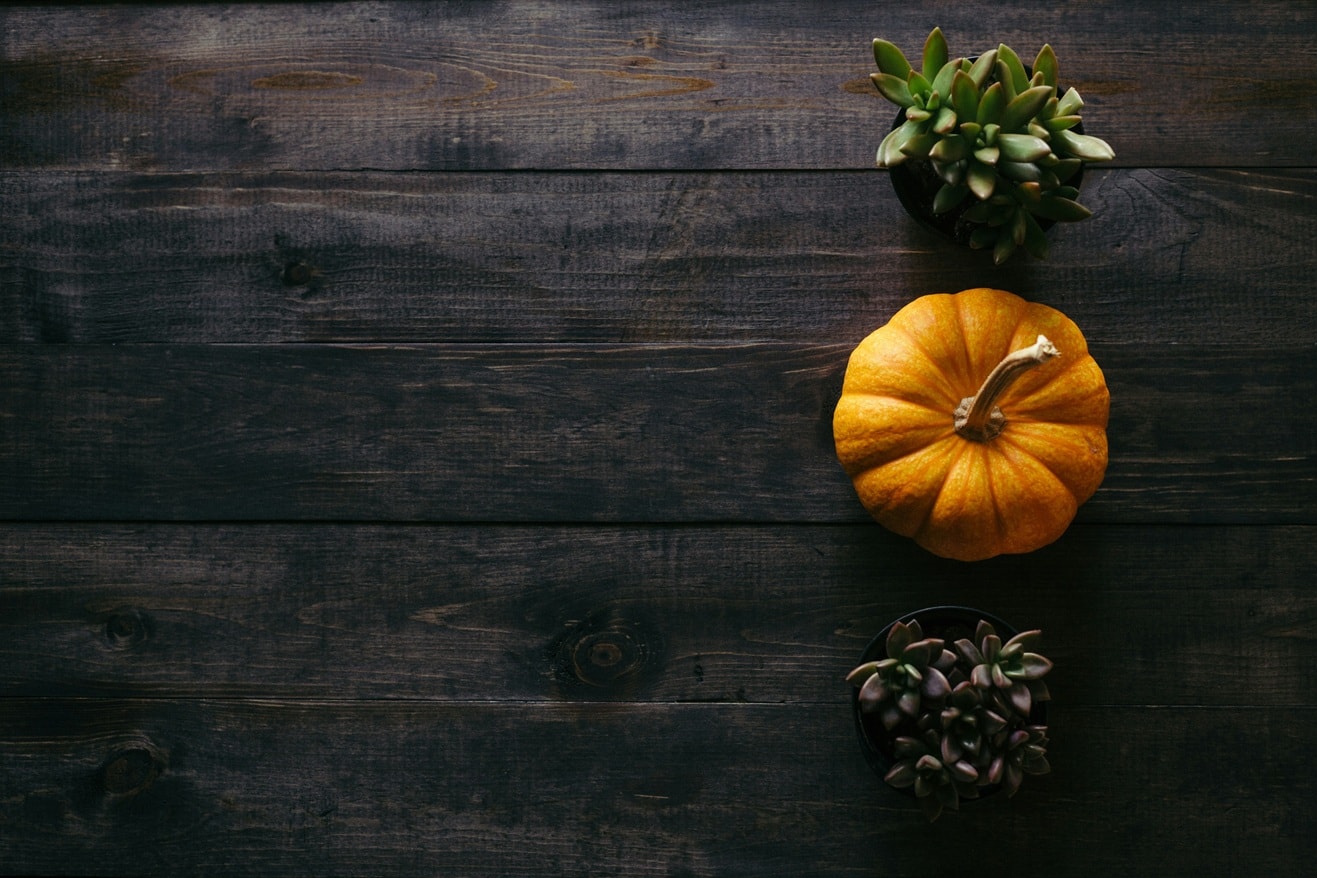
Comments
to join the conversation
Loading comments...