Leveraging WebView in Android Apps: Tips for Web Developers
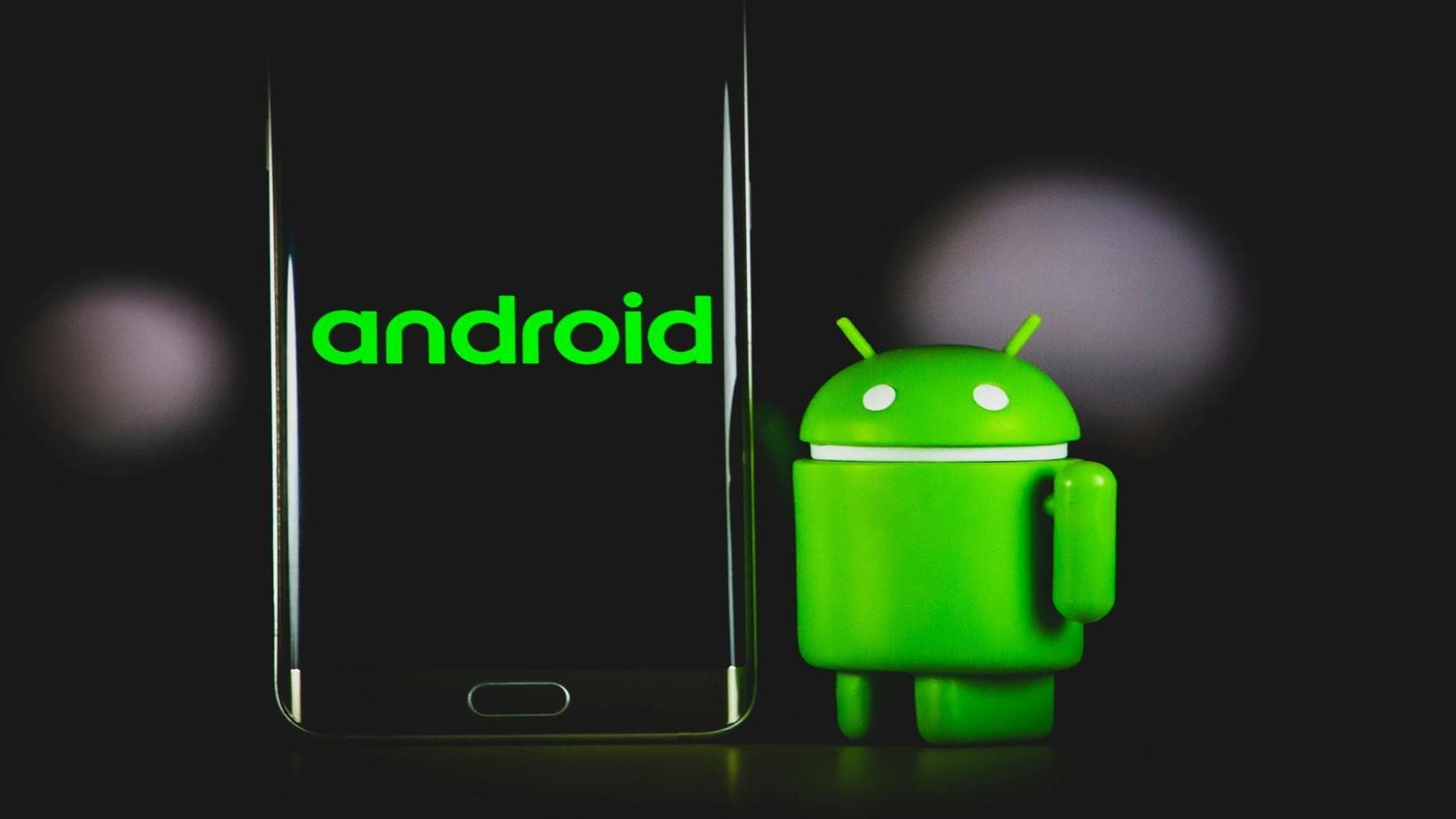
Leveraging WebView in Android Apps: Tips for Web Developers
Are you a web developer venturing into the world of Android app development? WebView can be a powerful tool for embedding web content directly into your Android application. However, ensuring a seamless user experience across both web and app contexts requires careful consideration.
In this guide, we'll explore how web developers can use WebView effectively to conditionally render content based on whether the app is viewed from a traditional browser or within an Android app WebView. Additionally, we'll cover the basics of creating an Android app using Android Studio with just a WebView pointing to a website.
Developing Android apps is more than just writing code; it's about unlocking new markets and reaching a vast, diverse user base. Each app has the potential to transform lives, foster connections, and create opportunities in ways we've never imagined.
Understanding WebView Context
When your website is viewed within an Android app's WebView, it's essential to recognize this context and tailor the user experience accordingly. Unlike a traditional web browser, WebView may present limitations or differences in functionality that can impact how your website renders and behaves.
advertisement
1. Detecting WebView Environment
To determine if your website is viewed within a WebView, you can use JavaScript to detect specific characteristics unique to WebView environments. For example, you can check if certain native methods or properties are available, indicating that the content is being viewed within an Android app WebView.
// Check if the website is viewed within an Android app WebView
function isWebView() {
return window.Android !== undefined; // Example: checking for Android native methods
}
By implementing such checks, you can conditionally adjust your website's behavior and appearance when viewed within WebView.
2. Conditional Rendering
Once you've detected the WebView environment, you can selectively render or hide certain elements to optimize the user experience for app users. For instance, you may want to hide elements like footers, advertisements, or navigation bars that are unnecessary or redundant within the app context.
// Conditionally hide elements when viewed within WebView
if (isWebView()) {
document.getElementById("footer").style.display = "none";
}
This approach allows you to tailor the presentation of your website when accessed through WebView, ensuring a more cohesive and native-like experience within the Android app.
3. Handling External Links
WebView in Android apps may not handle external links or navigation in the same way as traditional web browsers. To maintain control over user navigation and prevent unwanted behaviors, such as opening new tabs or navigating away from the app, you can intercept and handle link clicks within WebView.
// Prevent external links from opening in WebView
if (isWebView()) {
document.addEventListener("click", function (event) {
if (
event.target.tagName === "A" &&
!event.target.href.startsWith("your-app://")
) {
event.preventDefault(); // Prevent the default link behavior
// Handle link click within WebView
// Example: display a message or perform an action
}
});
}
By intercepting link clicks and implementing custom handling logic, you can ensure a consistent and controlled browsing experience for users within your Android app.
Android Studio, the official integrated development environment (IDE) for Android app development, offers developers a robust platform equipped with powerful tools and resources, streamlining the process of creating innovative and engaging mobile applications for the Android ecosystem.
Creating an Android App with WebView
Now, let's walk through the basics of creating an Android app using Android Studio with just a WebView pointing to a website.
-
Create a New Project: Open Android Studio and select "Create New Project." Choose "Empty Activity" and proceed with the default settings.
-
Add WebView to Layout: In the activity_main.xml layout file, add a WebView element to display your website. For example:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<WebView
android:id="@+id/webView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</RelativeLayout>
- Load Website in WebView: In the MainActivity.java file, load your website URL into the WebView.
import android.os.Bundle;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
WebView webView = findViewById(R.id.webView);
webView.setWebViewClient(new WebViewClient());
webView.loadUrl("https://www.example.com");
}
}
advertisement
- Permissions (Optional): Depending on your website's requirements, you may need to add permissions in the AndroidManifest.xml file, such as internet access permission.
<uses-permission android:name="android.permission.INTERNET" />
With these steps, you've created a basic Android app with a WebView that loads your website. You can further customize and enhance the app to suit your requirements.
Conclusion
WebView offers web developers a convenient means of integrating web content into Android applications. By leveraging WebView effectively and adapting your website's behavior for the app context, you can deliver a seamless and tailored user experience to your app users.
With these tips and techniques, you're equipped to optimize your website for WebView in Android apps, providing a cohesive and native-like experience for users transitioning between web and app environments.
advertisement
About the Author
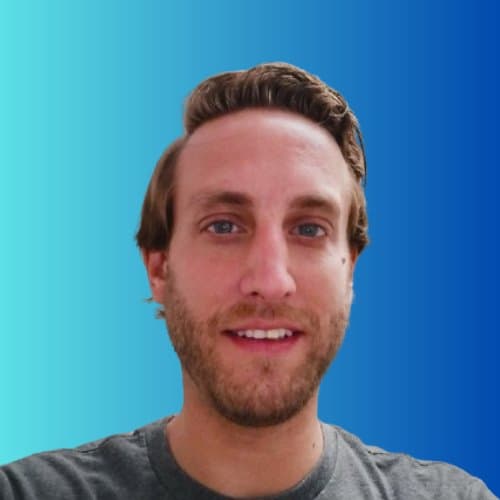
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All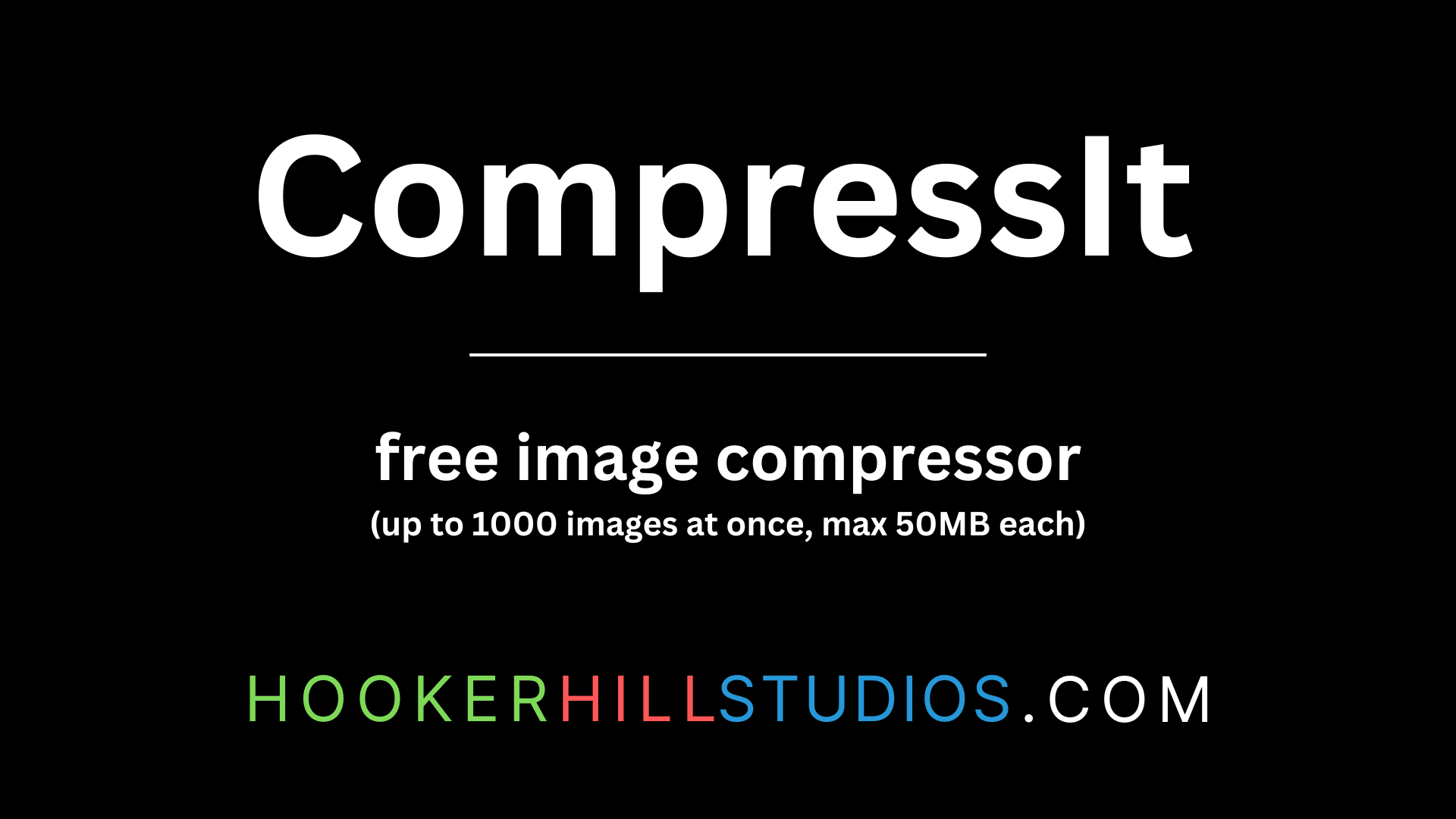
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024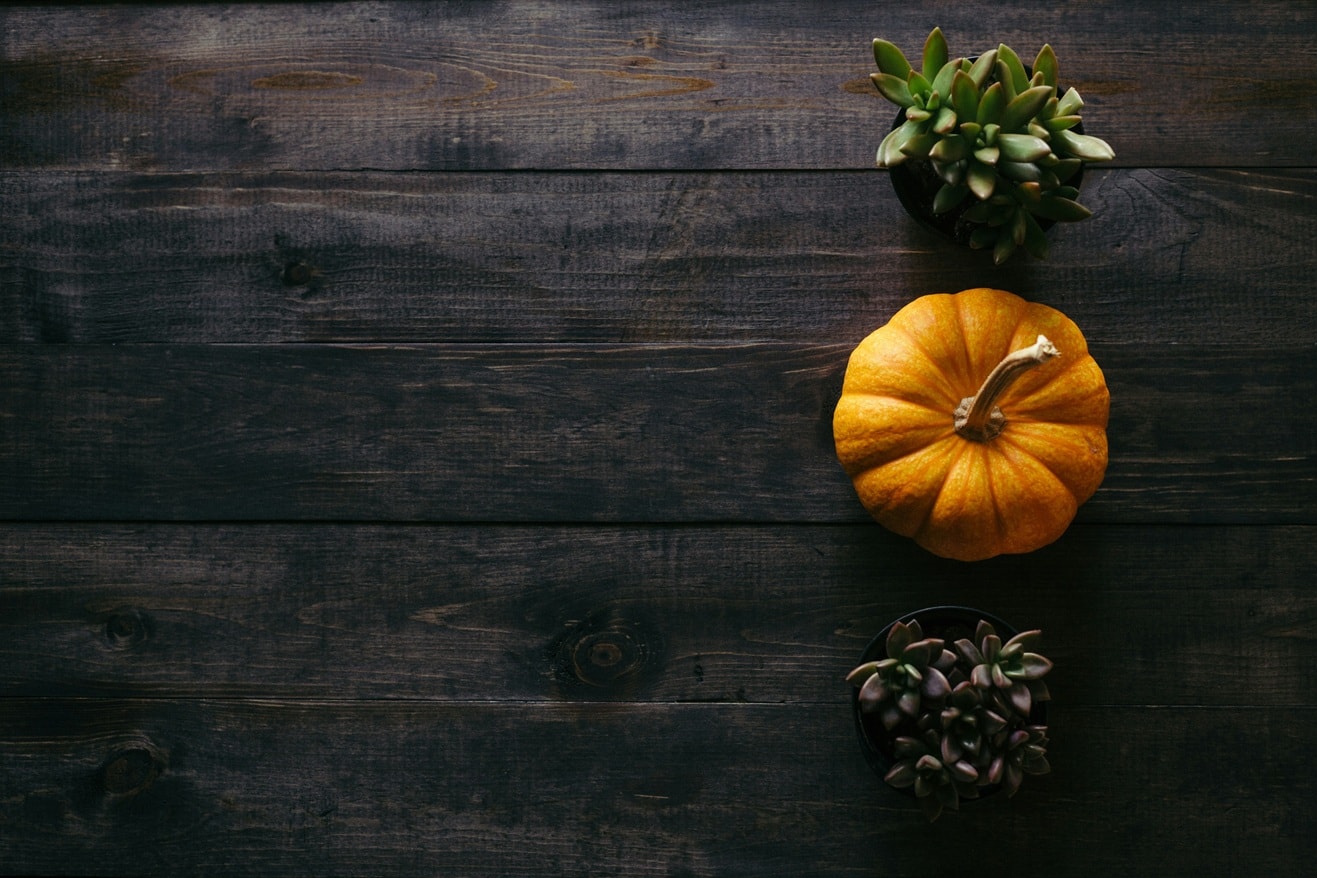
Comments
to join the conversation
Loading comments...