Image Compression with Python: Libraries & Use Cases
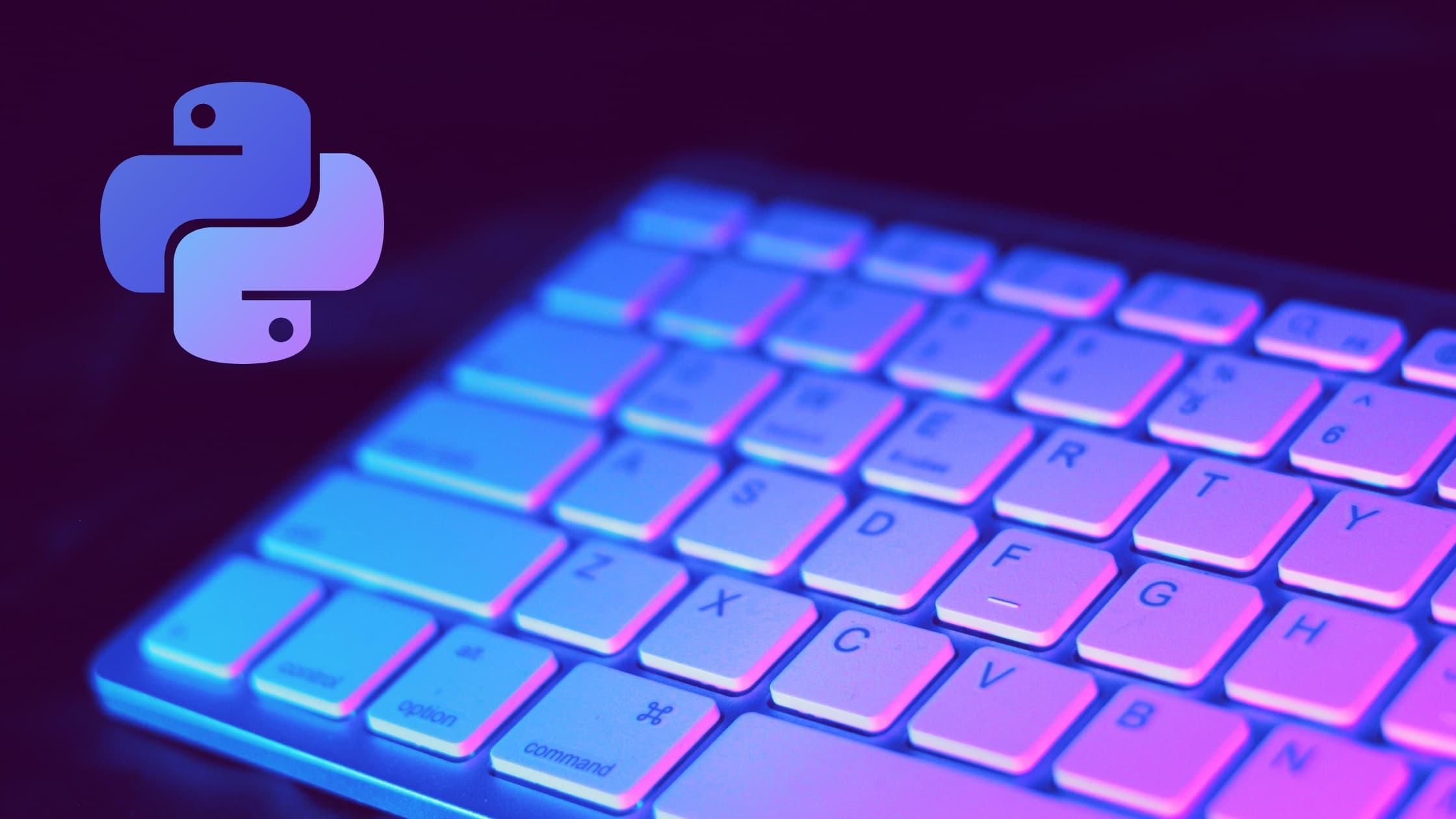
Table Of Contents
- Exploring Image Compression with Python: Libraries, Applications, and Use Cases
- Popular Python Libraries for Image Compression
- Pillow
- Basic Image Compression with Pillow
- Open an image file
- Save the image with reduced quality
- OpenCV
- Advanced Compression with OpenCV
- Read the image
- Compress the image with JPEG format and specified quality
- Save the compressed image
- imageio
- Basic Image Compression with imageio
- Open an image file
- Save the image with reduced quality
- PyTorch/TensorFlow
- Advanced Compression Techniques
- BFGS Algorithms
- Lossy vs. Lossless Compression
- Applications and Use Cases
- Web Development
- Mobile Apps
- Data Storage
- Resources
- Related Article:
- FAQ
- What is the difference between lossy and lossless image compression?
- Which Python library is best for image compression?
- How can image compression benefit web development?
- Are there any trade-offs with image compression?
- What are some modern alternatives to JPEG and PNG?
- Donate
- Conclusion
Exploring Image Compression with Python: Libraries, Applications, and Use Cases
Image compression is a crucial technology for managing digital content, reducing file sizes, and optimizing performance across various applications. By compressing images, we can improve website loading times, enhance mobile app performance, and manage storage more efficiently without significantly sacrificing visual quality. Python, known for its simplicity and robust ecosystem, offers several libraries to handle image compression effectively. This article delves into the most popular Python libraries for image compression, explores advanced techniques, and examines practical applications and use cases.
The JPEG image compression standard was first introduced in 1992 and has since become one of the most widely used formats for lossy image compression.
Popular Python Libraries for Image Compression
Pillow
Pillow, a fork of the Python Imaging Library (PIL), is one of the most widely used libraries for image processing in Python. It offers a straightforward interface for basic image manipulation tasks, including compression.
- Overview: Pillow provides various functions to open, manipulate, and save images in different formats. It supports operations like resizing, cropping, and format conversion, which are essential for effective image compression.
- Features: The
img.save()
function in Pillow allows you to specify the quality of the image when saving it, which directly affects the compression. The quality parameter ranges from 0 to 100, where lower values result in higher compression and reduced quality. - Example Use Case: Pillow is ideal for scenarios where you need to quickly compress images without requiring advanced features. For example, a web application that dynamically processes and serves user-uploaded images can use Pillow to ensure images are optimized for faster loading.
Basic Image Compression with Pillow
from PIL import Image
# Open an image file
img = Image.open('example.jpg')
# Save the image with reduced quality
img.save('example_compressed.jpg', quality=30) # Quality range is from 0 to 100
OpenCV
OpenCV (Open Source Computer Vision Library) is a powerful tool for computer vision and image processing. While it is known for more advanced image manipulation tasks, it also provides capabilities for image compression.
- Overview: OpenCV is a comprehensive library that supports a wide range of image processing techniques, from basic operations to complex algorithms.
- Features: OpenCV’s
cv2.imencode()
function allows for advanced compression options by specifying different parameters, including compression quality. - Example Use Case: OpenCV is useful in scenarios requiring advanced image processing alongside compression, such as pre-processing images for machine learning models or optimizing images for computer vision tasks.
Advanced Compression with OpenCV
import cv2
# Read the image
img = cv2.imread('example.jpg')
# Compress the image with JPEG format and specified quality
encode_param = [int(cv2.IMWRITE_JPEG_QUALITY), 30] # Quality range is from 0 to 100
result, encimg = cv2.imencode('.jpg', img, encode_param)
# Save the compressed image
if result:
with open('example_compressed.jpg', 'wb') as f:
f.write(encimg.tobytes())
imageio
imageio is a library designed for reading and writing images in various formats. It offers a simple API for basic image processing tasks, including compression.
- Overview: imageio supports numerous image formats and is known for its ease of use.
- Features: The library provides straightforward methods for image reading and writing, including options for adjusting image quality during compression.
- Example Use Case: imageio is ideal for scripts or applications that need to handle multiple image formats with minimal configuration.
Basic Image Compression with imageio
import imageio
# Open an image file
img = imageio.imread('example.jpg')
# Save the image with reduced quality
imageio.imwrite('example_compressed.jpg', img, format='jpeg', quality=30) # Quality range is from 0 to 100
PyTorch/TensorFlow
While not traditionally associated with image compression, deep learning frameworks like PyTorch and TensorFlow have been used to develop advanced image compression techniques using neural networks.
- Overview: These frameworks can be employed to train models for image compression, such as autoencoders, which learn to compress and reconstruct images with high fidelity.
- Advanced Techniques: Neural network-based compression can achieve better results compared to traditional methods, especially for specific types of images.
- Example Use Case: These methods are particularly useful in high-quality image storage or streaming applications, where traditional compression techniques may fall short.
advertisement
Advanced Compression Techniques
BFGS Algorithms
The Broyden-Fletcher-Goldfarb-Shanno (BFGS) algorithm is an optimization technique used in various fields, including image compression.
- Explanation: BFGS is a quasi-Newton method for solving unconstrained optimization problems. In image compression, it can be used to optimize the parameters of compression algorithms to achieve better balance between quality and file size.
- Application in Compression: By optimizing the compression parameters, BFGS can help in achieving more efficient compression with minimal quality loss.
Advanced image compression techniques using neural networks, such as autoencoders, are an active area of research and can offer better compression rates and image quality compared to traditional methods.
Lossy vs. Lossless Compression
Understanding the difference between lossy and lossless compression is essential for choosing the right approach based on application requirements.
- Definitions:
- Lossy Compression: Reduces file size by permanently removing some data, which can result in a loss of quality. Common in formats like JPEG.
- Lossless Compression: Reduces file size without losing any data, preserving the original quality. Used in formats like PNG and GIF.
- Pros and Cons:
- Lossy: Offers higher compression rates but at the cost of quality. Suitable for web images where perfect quality is less critical.
- Lossless: Maintains quality but achieves less compression. Ideal for images where quality preservation is crucial, such as archiving or professional photography.
- When to Use: Choose lossy compression for web and mobile applications where performance is a priority, and lossless compression for scenarios requiring high-quality images, such as archiving or professional photography.
Lossy compression algorithms like JPEG can reduce image file sizes by up to 90% without significantly impacting perceived image quality for typical web use.
Applications and Use Cases
Web Development
Image compression plays a significant role in web development by improving page load times and overall user experience.
- Impact on Performance: Compressed images reduce bandwidth usage and loading times, leading to faster and more responsive websites.
- Techniques: Utilize tools like WebP or responsive image techniques to serve appropriately compressed images based on device capabilities.
Mobile Apps
In mobile app development, image compression is crucial for optimizing performance and managing data usage.
- Performance Considerations: Compressed images lead to faster app performance and reduced data consumption, which is especially important for users with limited data plans.
- Best Practices: Implement image compression techniques during image upload or processing to ensure efficient storage and display.
Data Storage
Efficient image compression can significantly impact data storage, reducing costs and improving management.
- Efficiency: Compressed images take up less space, which can lead to cost savings in data storage and improved system performance.
- Real-World Examples: Large-scale storage solutions, such as cloud storage services, benefit from image compression to manage vast amounts of data efficiently.
PNG, a common format for lossless compression, supports transparency, making it ideal for images requiring clear backgrounds, like logos and icons.
advertisement
Resources
- Pillow Documentation: Pillow Docs
- OpenCV Documentation: OpenCV Docs
- imageio Documentation: imageio Docs
- PyTorch Documentation: PyTorch Docs
- TensorFlow Documentation: TensorFlow Docs
- WebP Format: WebP Information
Related Article:
FAQ
What is the difference between lossy and lossless image compression?
Lossy compression reduces file size by permanently removing some data, which can result in a loss of image quality. Common formats include JPEG. Lossless compression reduces file size without losing any data, preserving the original quality. Common formats include PNG and GIF.
Which Python library is best for image compression?
It depends on your needs:
- Pillow is great for basic compression tasks and ease of use.
- OpenCV offers advanced compression features and is useful for complex image processing.
- imageio provides a simple API for handling various formats.
- PyTorch/TensorFlow are used for advanced techniques involving neural networks.
How can image compression benefit web development?
Compressed images lead to faster page load times, reduced bandwidth usage, and an overall improved user experience. This is crucial for enhancing the performance of websites, especially those with high-resolution images.
Are there any trade-offs with image compression?
Yes, with lossy compression, you may experience a reduction in image quality, which might be noticeable in some cases. Lossless compression preserves quality but may not achieve as high a reduction in file size.
What are some modern alternatives to JPEG and PNG?
WebP is a modern image format developed by Google that provides superior compression and quality compared to JPEG and PNG. It supports both lossy and lossless compression and is gaining broader support in web browsers.
WebP is a modern image format developed by Google that provides superior compression and quality compared to JPEG and PNG, but its support varies across browsers and platforms.
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Conclusion
Image compression is a vital process in modern digital applications, enabling efficient storage, faster performance, and better user experiences. Python provides a range of libraries, from basic tools like Pillow and imageio to advanced options like OpenCV and deep learning frameworks, to handle image compression tasks. By understanding the different libraries and techniques available, developers can make informed decisions on how to best compress images for their specific needs. As technology evolves, staying updated on the latest advancements in image compression will continue to be important for optimizing digital content.
Feel free to leave a comment below with your thoughts and suggestions.
advertisement
About the Author
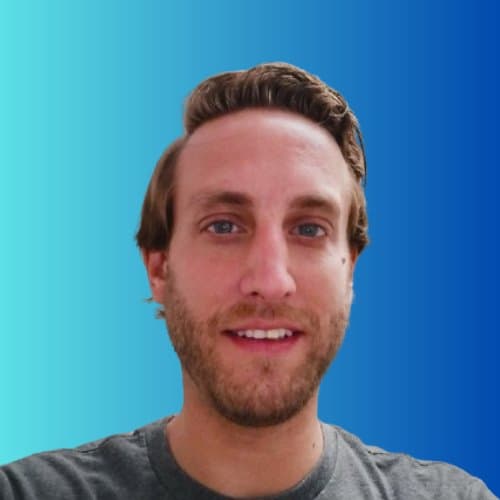
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All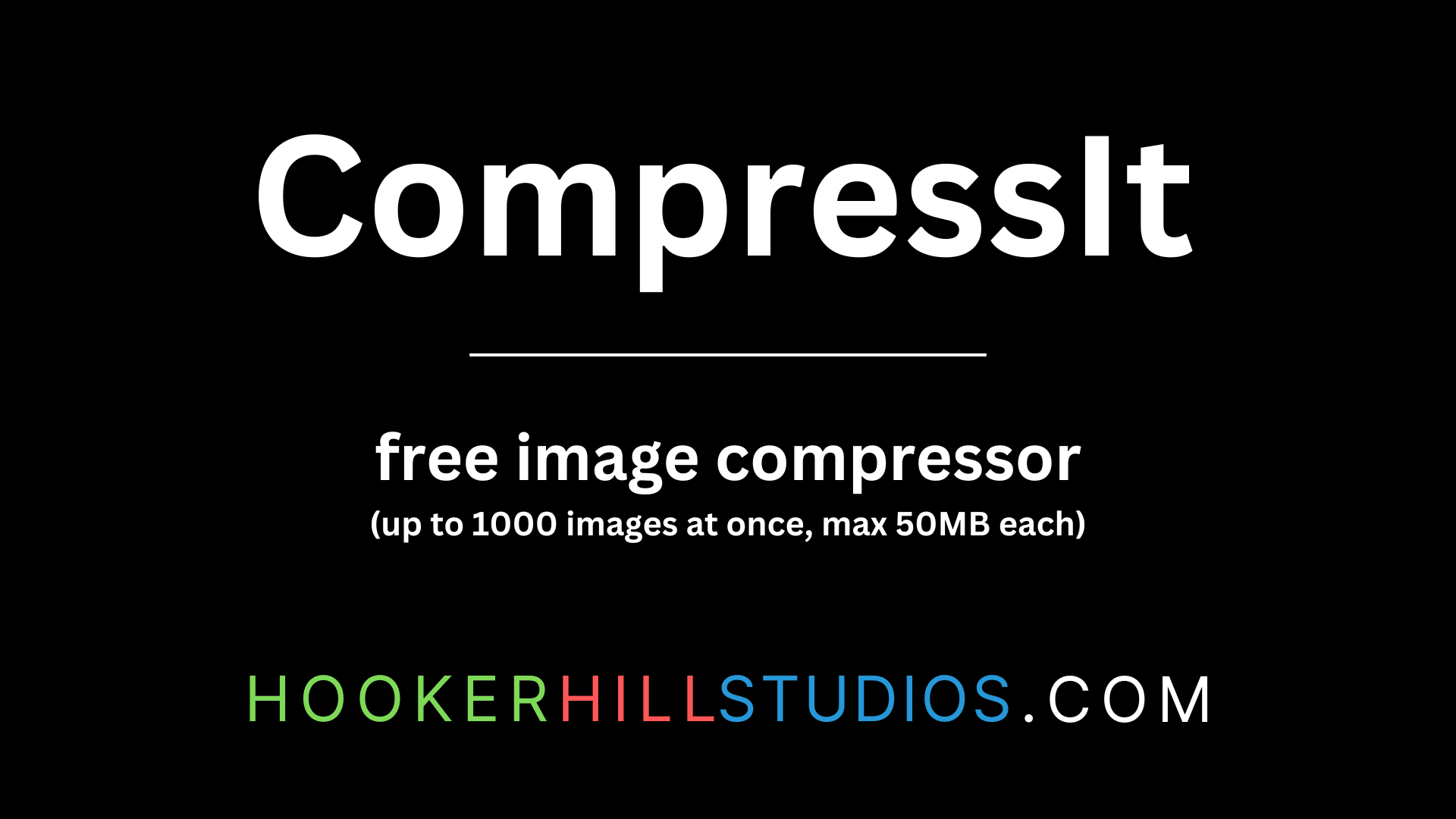
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024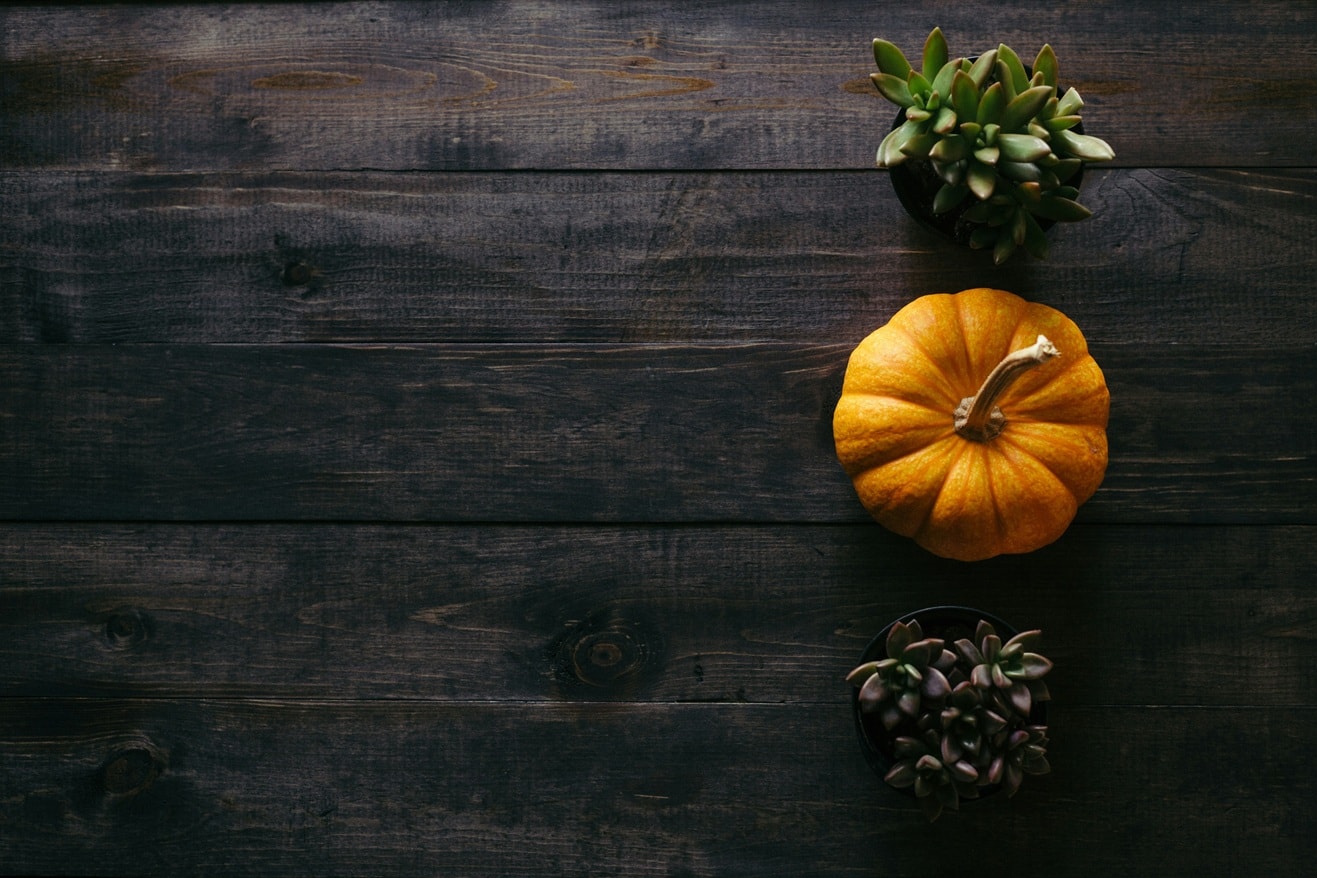
to join the conversation