How to Package Python Apps into .exe with PyInstaller: Easy Guide
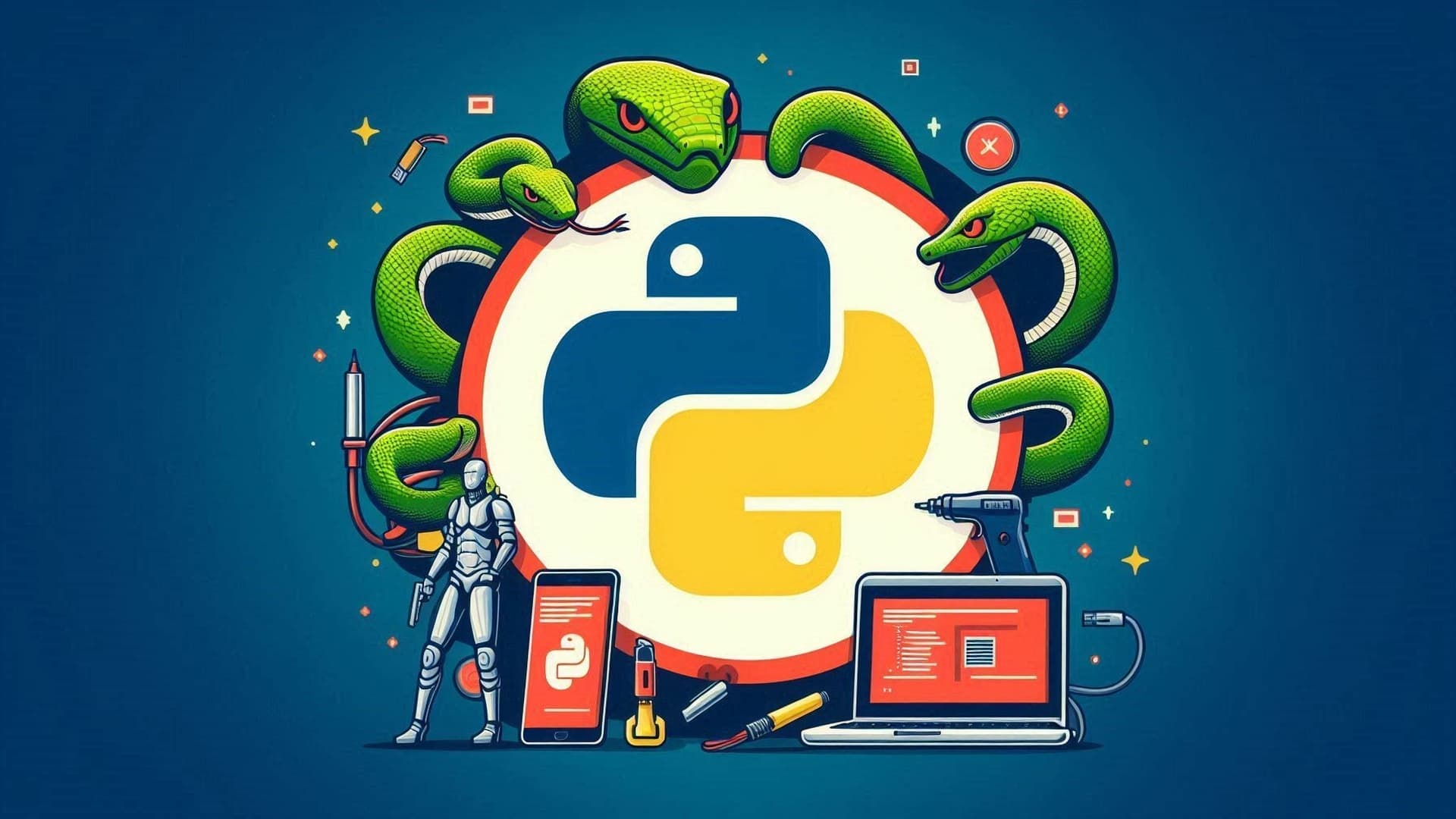
Table Of Contents
- Packaging Python Apps into .exe Using PyInstaller: A User-Friendly Guide
- Getting Started with PyInstaller
- Installation
- Basic Usage
- Customizing Your Executable
- Adding an Icon
- Preventing the Command Prompt from Appearing
- Adding a Splash Screen
- Including Additional Files
- Modifying the Spec File
- -*- mode: python ; coding: utf-8 -*-
- Common Issues and Troubleshooting
- Missing Modules
- Large Executable Size
- FAQ
- Donate
- Conclusion
Creating standalone executables from Python scripts can be essential for distributing your applications to users who do not have Python installed. PyInstaller is a popular tool for converting Python scripts into executable files (.exe). This guide will walk you through the process of using PyInstaller, including tips on common flags and customization options.
Packaging Python Apps into .exe Using PyInstaller: A User-Friendly Guide
Python was created by Guido van Rossum and was first released in 1991. Guido named the language after "Monty Python's Flying Circus," a British comedy series, which is why Python has a playful and approachable design philosophy. The language was designed to be easy to read and write, making it accessible to both beginners and experienced programmers.
Getting Started with PyInstaller
Installation
First, ensure you have PyInstaller installed. You can install it using pip:
pip install pyinstaller
Basic Usage
The simplest way to create an executable is by running:
pyinstaller your_script.py
This will generate several files and directories in your working directory:
-
dist/
- Contains the executable and its dependencies. -
build/
- Contains temporary files used during the build process. -
your_script.spec
- The spec file which contains the build configuration. -
__pycache__/
- Bytecode cache files.
To create a single executable file, use the --onefile flag:
pyinstaller --onefile your_script.py
Customizing Your Executable
PyInstaller offers several options to customize your executable. Below are some common flags and how to use them.
Adding an Icon
You can specify an icon for your executable using the --icon flag:
pyinstaller --onefile --icon=your_icon.ico your_script.py
Preventing the Command Prompt from Appearing
If your application is a GUI and you want to prevent the command prompt from popping up, use the --noconsole flag:
pyinstaller --onefile --noconsole your_script.py
Python is a highly versatile programming language used in a wide range of fields, from web development and data analysis to artificial intelligence and scientific computing. Libraries like Django and Flask make it popular for web development, while Pandas, NumPy, and SciPy are widely used in data science. Additionally, TensorFlow and PyTorch have made Python a leading language in machine learning and AI.
advertisement
Adding a Splash Screen
To add a splash screen that appears while your application is loading, use the --splash flag:
pyinstaller --onefile --splash=splashfile.png your_script.py
Including Additional Files
To include additional files (e.g., data files, configuration files) with your executable, use the --add-data flag:
pyinstaller --onefile --add-data 'data_file.txt;.' your_script.py
Modifying the Spec File
For more complex customizations, you can modify the generated .spec file. This file is created the first time you run PyInstaller on your script and allows for detailed configuration of the build process.
For example, to add an icon and include a data file, your spec file might look like this:
# -*- mode: python ; coding: utf-8 -*-
block_cipher = None
a = Analysis(
['your_script.py'],
pathex=['/path/to/your/script'],
binaries=[],
datas=[('data_file.txt', '.')], # Include data file
hiddenimports=[],
hookspath=[],
runtime_hooks=[],
excludes=[],
win_no_prefer_redirects=False,
win_private_assemblies=False,
cipher=block_cipher,
noarchive=False,
)
pyz = PYZ(a.pure, a.zipped_data, cipher=block_cipher)
exe = EXE(
pyz,
a.scripts,
[],
exclude_binaries=True,
name='your_script',
debug=False,
bootloader_ignore_signals=False,
strip=False,
upx=True,
console=False, # Prevent command prompt
icon='your_icon.ico', # Add icon
)
coll = COLLECT(
exe,
a.binaries,
a.zipfiles,
a.datas,
strip=False,
upx=True,
upx_exclude=[],
name='your_script',
)
To build your application using the modified spec file, run:
pyinstaller your_script.spec
Common Issues and Troubleshooting
Missing Modules
Sometimes PyInstaller may not automatically detect all necessary modules. You can explicitly add hidden imports using the --hidden-import flag:
pyinstaller --onefile --hidden-import=module_name your_script.py
Large Executable Size
To reduce the size of the executable, you can use the UPX packer. UPX compresses the executable, making it smaller:
pip install upx
pyinstaller --onefile --upx-dir=/path/to/upx your_script.py
FAQ
How do I update my application?
To update your application, modify your script and re-run PyInstaller. You may need to clean up the previous build and dist directories.
Can I use PyInstaller for multi-platform builds?
PyInstaller needs to be run on the target platform. For example, to create a Windows executable, you must run PyInstaller on a Windows machine.
Why does my executable not run on another machine?
Ensure all dependencies are included and compatible with the target system. Missing Visual C++ Redistributable packages can also cause issues.
How do I debug my executable?
You can enable debug mode by setting the debug parameter in the spec file to True or using the --debug flag when running PyInstaller.
Unlike many other programming languages, Python uses indentation to define code blocks instead of curly braces or keywords. This reliance on indentation (whitespace) promotes readability and enforces a clean, consistent coding style. While this can be surprising to new programmers, it helps maintain a visually uncluttered structure and reduces the likelihood of errors related to code block delimiters.
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Conclusion
Packaging Python applications into .exe files using PyInstaller is a powerful way to distribute your software. With a range of customization options and flags, you can tailor the executable to meet your specific needs.
For more detailed information, refer to the PyInstaller documentation here https://pyinstaller.readthedocs.io/.
advertisement
About the Author
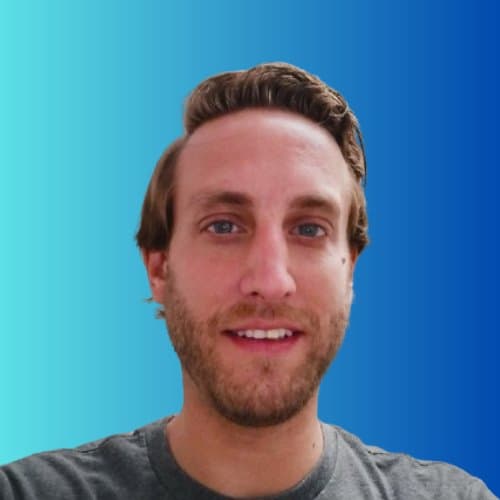
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All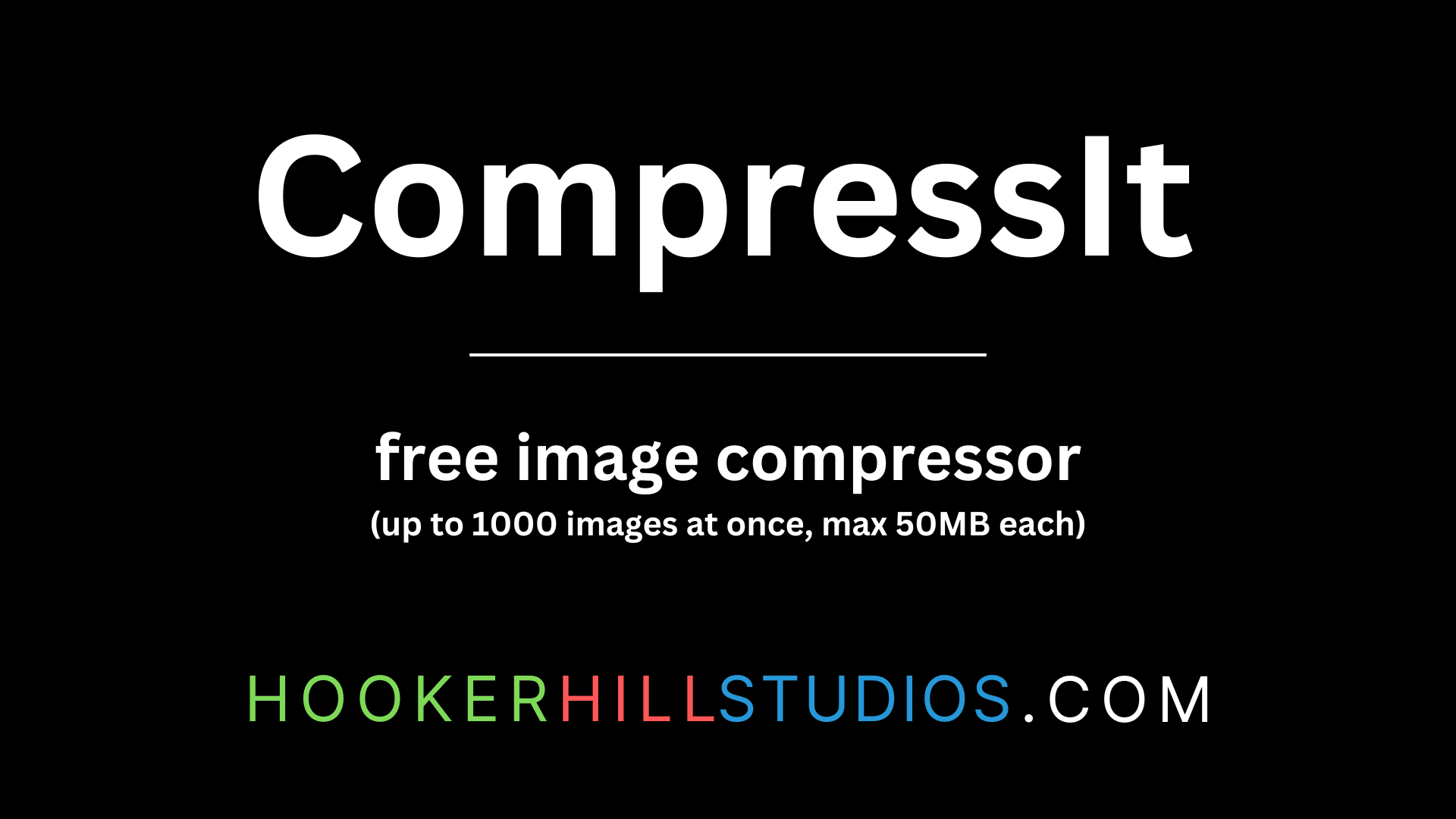
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024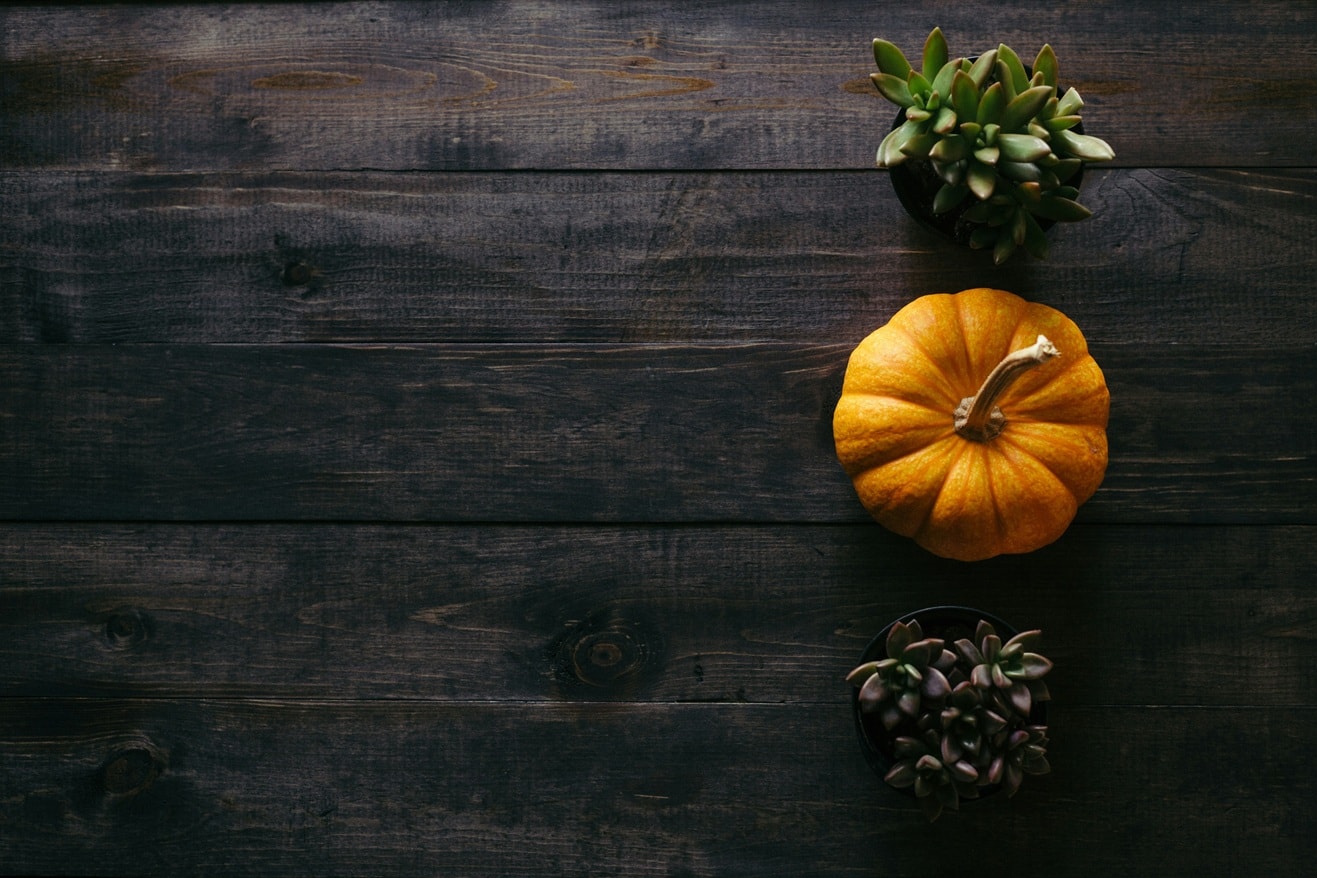
Comments
to join the conversation
Loading comments...