Next.js 15 RC: Key Features & Improvements You Need to Know
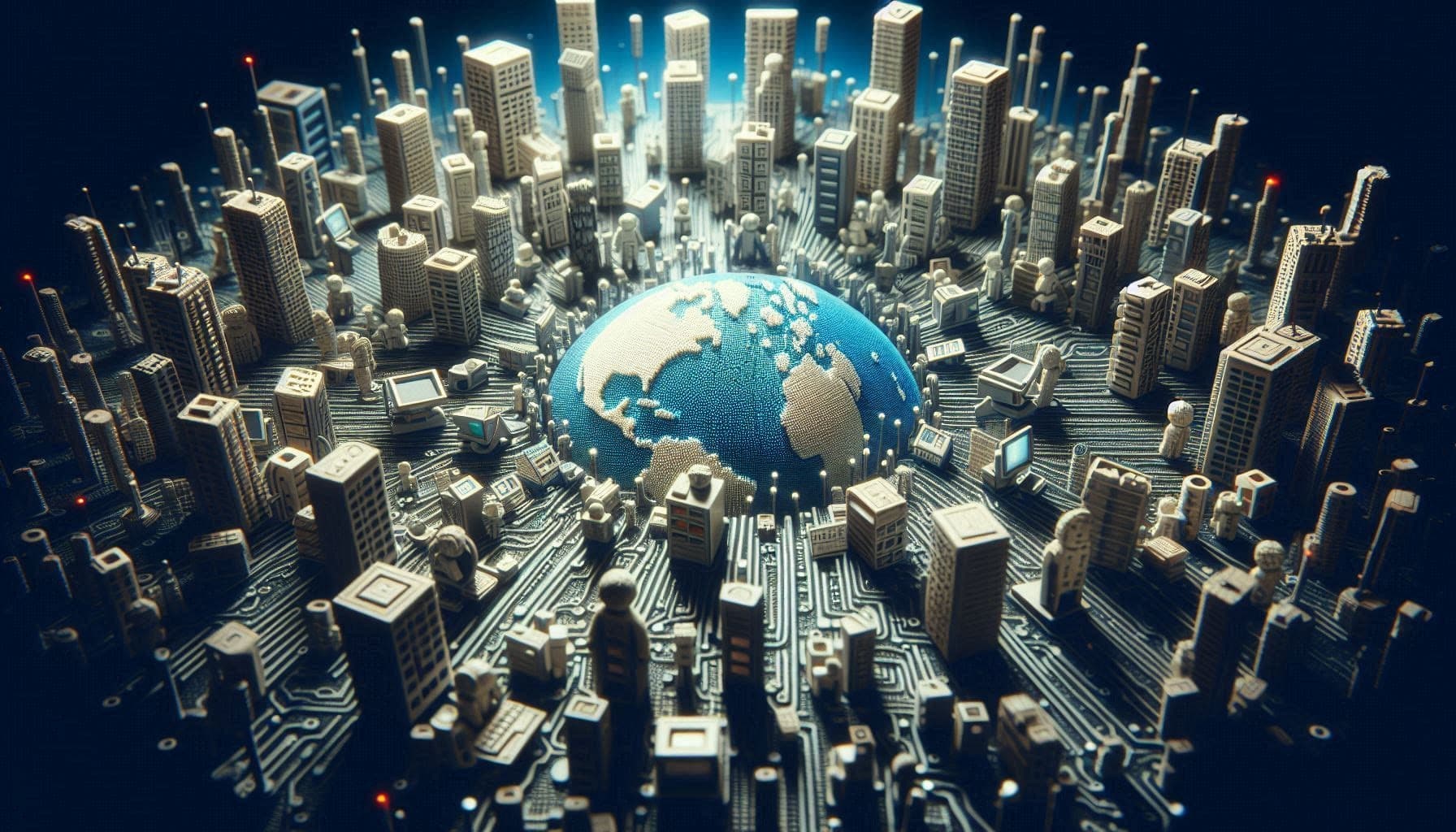
Table Of Contents
- What's New in Next.js 15 RC: An Overview
- Support for React 19 RC
- Experimental: React Compiler
- How to Enable React Compiler
- Improved Hydration Error Messages
- Caching Updates
- Fetch Requests
- GET Route Handlers
- Client Router Cache
- Experimental: Partial Prerendering (PPR)
- How to Enable PPR
- Experimental: next/after API
- How to Use next/after
- create-next-app Updates
- Bundling External Packages
- Other Changes
- Breaking Changes
- Improvements
- Documentation
- Donate
- Conclusion
What's New in Next.js 15 RC: An Overview
The release candidate (RC) for Next.js 15 has just been announced, bringing a host of new features, improvements, and breaking changes. This article delves into the key updates, experimental features, and breaking changes that developers need to be aware of. Here's everything you need to know about the Next.js 15 RC.
Next.js has first-class support for TypeScript. You can start using TypeScript simply by adding a tsconfig.json file to your project, and Next.js will automatically handle TypeScript compilation and type checking.
Support for React 19 RC
Next.js 15 RC includes support for React 19 RC. As the Next.js App Router is built on the React canary channel, this support allows developers to experiment with new React APIs before the official React 19 release. Key features like Actions have been integrated into both client and server environments, although it’s important to note that some third-party libraries might not yet be compatible with React 19.
Experimental: React Compiler
One of the most exciting experimental features in this release is the React Compiler. Developed by the React team at Meta, this experimental compiler understands plain JavaScript semantics and the Rules of React, enabling automatic optimizations. This reduces the need for manual memoization through hooks like useMemo
and useCallback
, making code simpler and easier to maintain.
Vercel and the Next.js team have created an open-source e-commerce template called Next.js Commerce. It provides a customizable and performance-optimized foundation for building e-commerce sites with Next.js.
advertisement
How to Enable React Compiler
To enable the React Compiler in Next.js 15 RC, you need to install a specific package and configure your next.config.js
file accordingly. Optionally, you can also run the compiler in an "opt-in" mode for more controlled testing.
In the terminal:
npm install babel-plugin-react-compiler
Then update your next.config.js
file:
// next.config.js
const nextConfig = {
experimental: {
reactCompiler: true,
},
};
module.exports = nextConfig;
Note: The React Compiler can currently only be used in Next.js with a Babel plugin, which could result in slower build times. Source: https://nextjs.org/blog/next-15-rc
Improved Hydration Error Messages
Next.js 15 RC enhances the developer experience with improved hydration error messages. This version provides a more informative error view, displaying the source code that caused the error along with suggestions for resolving it.
Caching Updates
One of the significant changes in Next.js 15 RC is the shift in caching behavior. Previously, fetch requests, GET Route Handlers, and client-side navigations were cached by default. In this release, they are no longer cached by default.
Next.js automatically splits your code into smaller chunks. This ensures that users only load the JavaScript necessary for the page they are visiting, which improves load times and overall performance.
Fetch Requests
In Next.js 14, fetch
requests defaulted to a caching strategy. Now, the default is no-store
, meaning that resources will be fetched from the server on every request without updating the cache. Developers can still opt into caching by explicitly setting the appropriate options in their fetch calls or by configuring their routes to use a different strategy.
GET Route Handlers
Similarly, GET Route Handlers are now uncached by default. You can opt into caching by configuring the route settings.
Client Router Cache
Page components are also no longer cached by default in the Client Router Cache. However, shared layout data and back/forward navigations will still be cached to ensure consistent user experiences.
advertisement
Experimental: Partial Prerendering (PPR)
Partial Prerendering (PPR) was introduced in Next.js 14 as an optimization combining static and dynamic rendering on the same page. Next.js 15 RC allows for incremental adoption of PPR, enabling developers to opt specific layouts and pages into PPR with the experimental config option.
How to Enable PPR
To enable PPR incrementally, you will need to update your next.config.js
file with specific experimental settings. Once all segments have PPR enabled, you can enable it for the entire app.
// next.config.js
const nextConfig = {
experimental: {
ppr: 'incremental',
},
};
module.exports = nextConfig;
Experimental: next/after API
The next/after
API is another experimental feature in Next.js 15 RC. It allows you to execute code after a response has finished streaming, enabling secondary tasks like logging and analytics to run without blocking the primary response.
Guillermo Rauch and his team at Vercel developed Next.js to simplify the process of building server-rendered React applications, offering features like server-side rendering, static site generation, and a built-in routing system.
How to Use next/after
To use the next/after
API, you need to modify your next.config.js
file and then implement it in your server components or route handlers.
// next.config.js
const nextConfig = {
experimental: {
after: true,
},
};
module.exports = nextConfig;
Then, import and use it in your server components or route handlers:
import { unstable_after as after } from 'next/server';
import { log } from '@/app/utils';
export default function Layout({ children }) {
after(() => {
log();
});
return <>{children}</>;
}
create-next-app Updates
The create-next-app
tool has been updated with a new design and additional features. Notably, a new prompt now asks whether you want to enable Turbopack for local development. There’s also a new --empty flag to create a minimal "hello world" page, removing any extraneous files and styles.
Bundling External Packages
Next.js 15 RC introduces new configuration options for bundling external packages. In the App Router, external packages are now bundled by default. For the Pages Router, you can enable bundling with a specific configuration option.
Next.js includes an image component (next/image) that automatically optimizes images for performance. This component supports features like responsive images, lazy loading, and image optimization through a built-in image optimization service or a custom loader.
Other Changes
Next.js 15 RC includes several other updates and breaking changes that developers should be aware of:
Breaking Changes
- Minimum React version is now 19 RC.
- next/image: Removed
squoosh
in favor ofsharp
as an optional dependency. - next/image: Changed default
Content-Disposition
toattachment
. - next/image: An error is now thrown when
src
has leading or trailing spaces. - Middleware: The
react-server
condition now applies to limit unrecommended React API imports. - next/font: Removed support for the external
@next/font
package. - next/font: Removed font-family hashing.
- Caching:
force-dynamic
will now set ano-store
default to the fetch cache. - Config: Enabled
swcMinify
,missingSuspenseWithCSRBailout
, andoutputFileTracing
behavior by default, removing deprecated options. - Speed Insights: Removed auto-instrumentation for Speed Insights; developers must now use the dedicated
@vercel/speed-insights
package. - Sitemap: Removed
.xml
extension for dynamic sitemap routes and aligned sitemap URLs between development and production.
advertisement
Improvements
- Metadata: Updated environmental variable fallbacks for
metadataBase
when hosted on Vercel. - Tree-shaking: Fixed tree-shaking with mixed namespace and named imports from
optimizePackageImports
. - Parallel Routes: Provided unmatched catch-all routes with all known params.
- Configuration:
bundlePagesExternals
is now stable and renamed tobundlePagesRouterDependencies
.serverComponentsExternalPackages
is now stable and renamed toserverExternalPackages
.
- create-next-app: New projects now ignore all
.env
files by default.
Documentation
- Auth Documentation: Improved documentation for authentication.
- @next/env Package: Updated documentation for the
@next/env
package.
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Next.js supports both server-side rendering (SSR) and static site generation (SSG). SSR allows pages to be generated on the server at request time, while SSG generates pages at build time, which can be served as static files. This flexibility helps optimize performance and SEO.
Conclusion
Next.js 15 RC brings a plethora of new features, experimental tools, and breaking changes. As this is a release candidate, it’s essential to test these changes in your development environment before the stable release. You can install Next.js 15 RC with specific commands.
For more detailed information, visit the Next.js documentation.
advertisement
About the Author
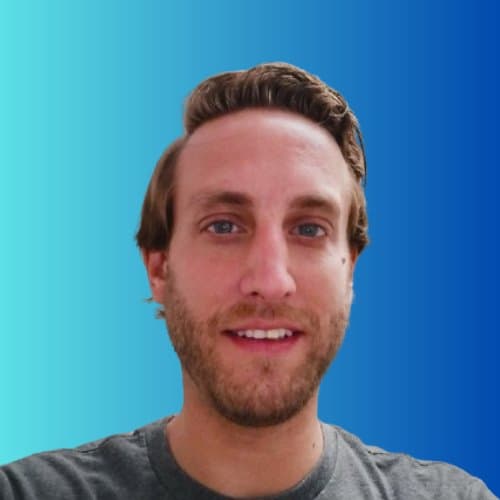
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All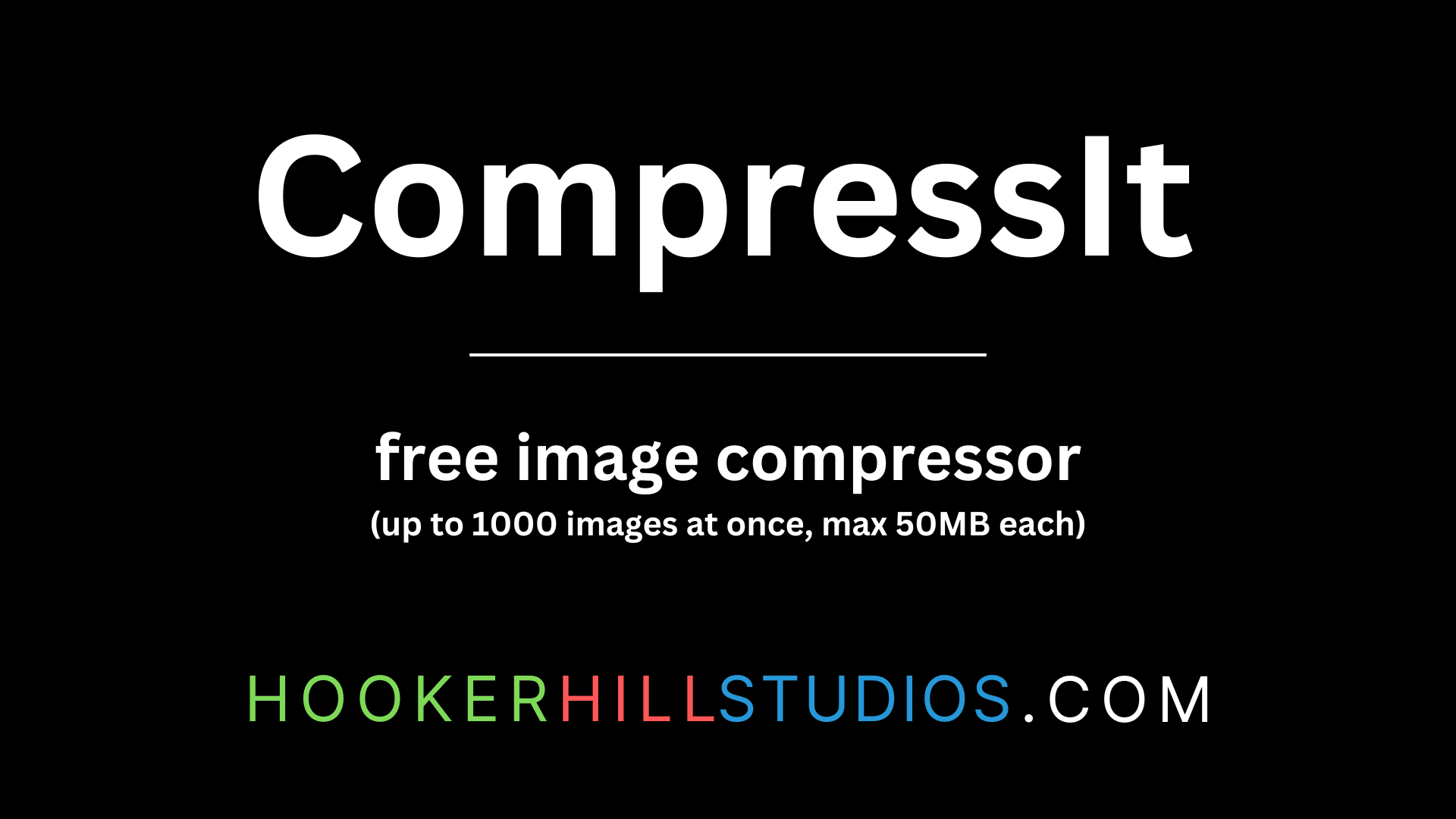
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024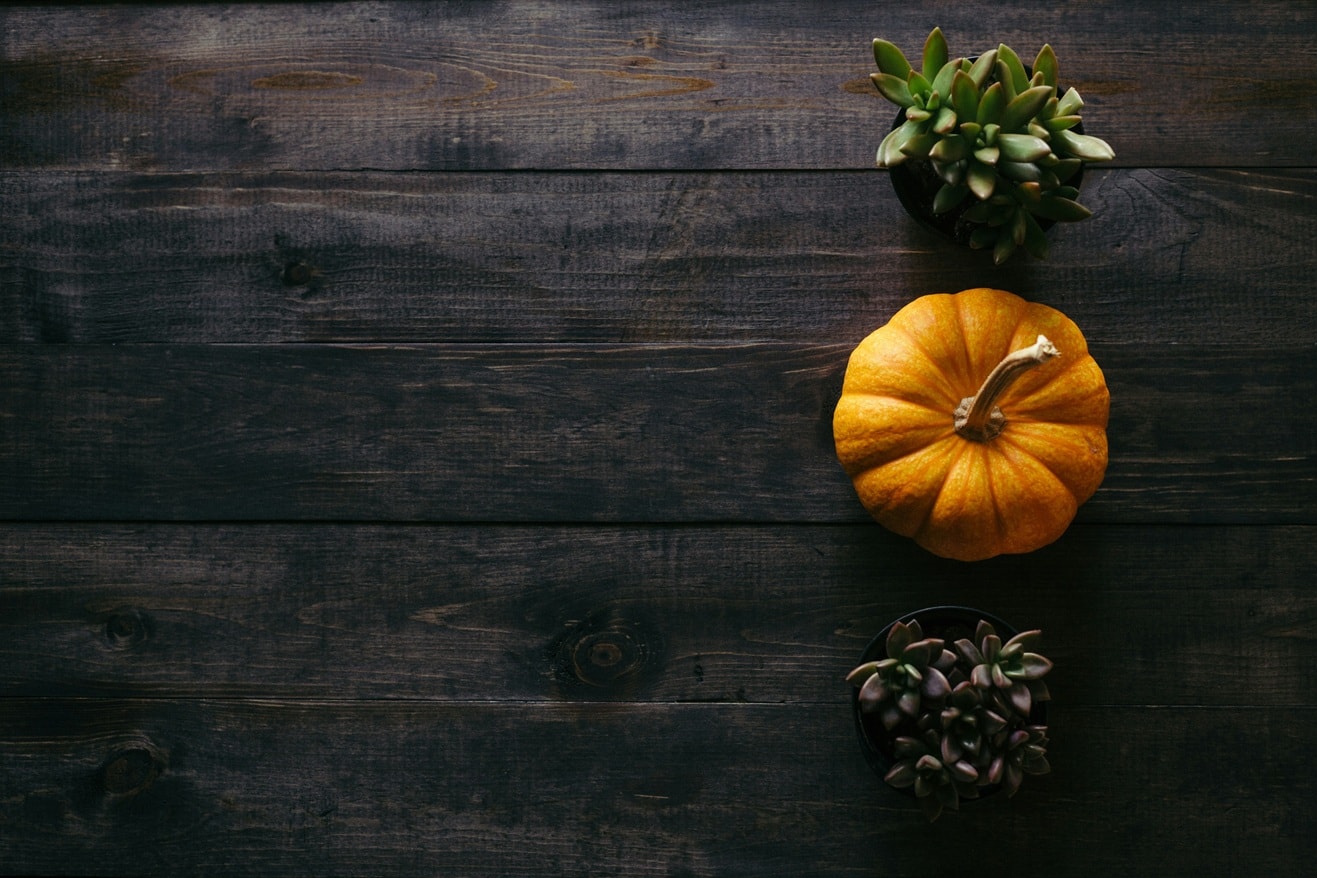
to join the conversation