Building Your First Progressive Web App with HTML, CSS, and JavaScript: A Beginner's Guide
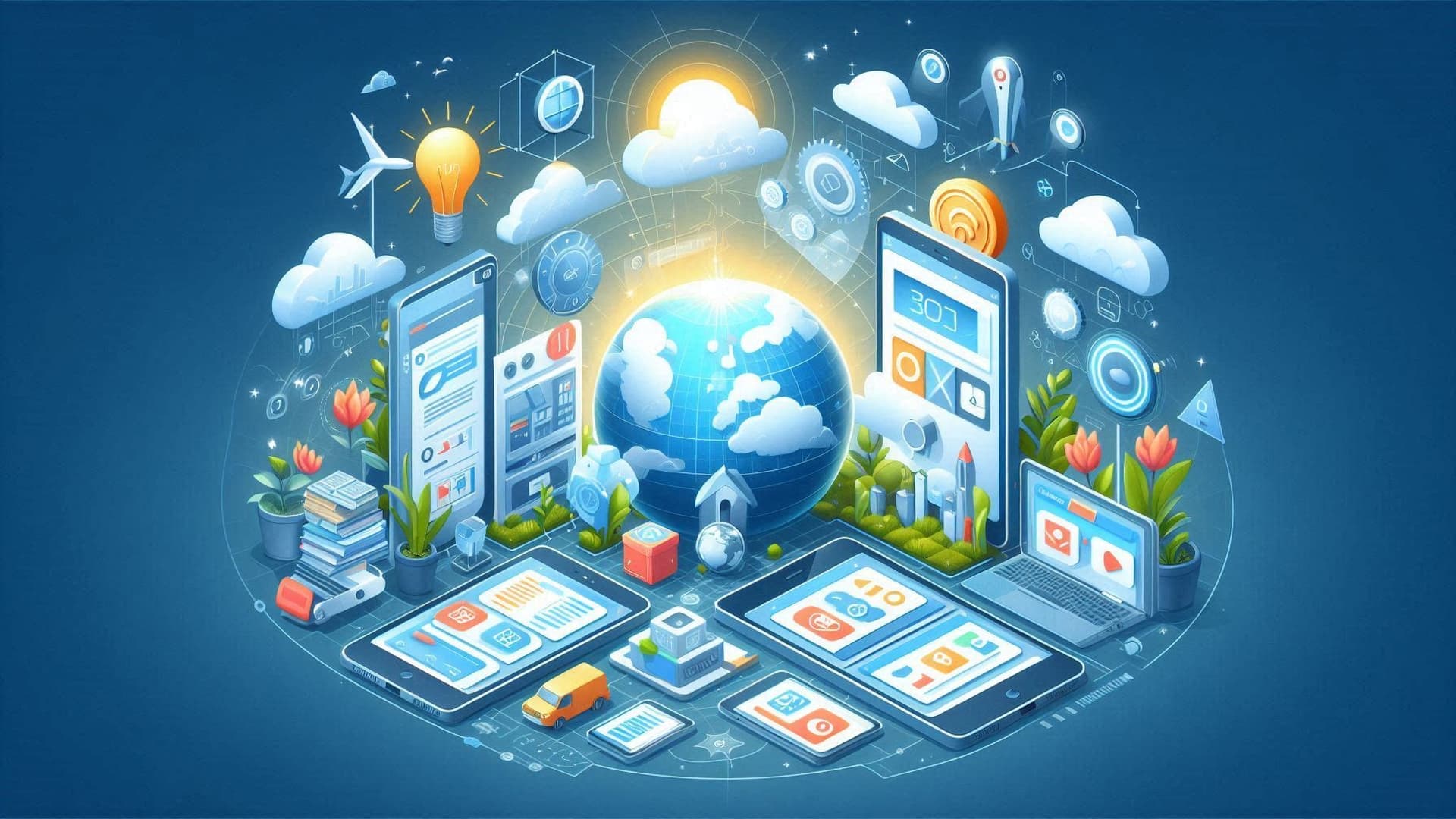
Table Of Contents
- Building Your First Progressive Web App: A Beginner's Guide
- Introduction
- What is a Progressive Web App?
- Step 1: Setting Up Your Project
- index.html
- styles.css
- app.js
- Step2: Creating the Service worker
- service-worker.js
- app.js
- Step 3: Adding a Web App Manifest
- mainifest.json
- index.html
- Step 4: Testing Your PWA
- FAQ
- Conclusion
- Complete Files for Your First PWA
- index.html
- styles.css
- app.js
- service-worker.js
- manifest.json
- Related Articles
Building Your First Progressive Web App: A Beginner's Guide
Introduction
Progressive Web Apps (PWAs) combine the best of web and mobile apps. They offer the reliability and engagement of a native app but are built using web technologies. In this guide, you'll learn the essential steps to create your first PWA, complete with code examples and best practices.
Offline Capabilities: PWAs can work offline or in low network conditions using service workers.
What is a Progressive Web App?
A PWA is a web application that uses modern web capabilities to deliver an app-like experience to users. Key characteristics of PWAs include:
- Reliable: Loads instantly and reliably, even on flaky networks.
- Fast: Responds quickly to user interactions with smooth animations.
- Engaging: Feels like a natural app on the device with an immersive user experience.
Step 1: Setting Up Your Project
First, create a simple web app structure. For this guide, we'll use a basic HTML, CSS, and javascript setup.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>My First PWA</title>
<link rel="stylesheet" href="styles.css" />
<link rel="manifest" href="/manifest.json" />
</head>
<body>
<h1>Welcome to My First PWA</h1>
<p>This is a simple Progressive Web App.</p>
<script src="app.js"></script>
</body>
</html>
styles.css
body {
font-family: Arial, sans-serif;
text-align: center;
margin-top: 50px;
}
h1 {
color: #333;
}
p {
color: #666;
}
app.js
if ("serviceWorker" in navigator) {
window.addEventListener("load", () => {
navigator.serviceWorker.register("/service-worker.js").then(
(registration) => {
console.log(
"ServiceWorker registration successful with scope: ",
registration.scope
);
},
(error) => {
console.log("ServiceWorker registration failed: ", error);
}
);
});
}
Step2: Creating the Service worker
A service worker is a script that your browser runs in the background, separate from the web page. It allows you to manage network requests, cache resources, and provide offline functionality.
service-worker.js
self.addEventListener("install", (event) => {
event.waitUntil(
caches.open("v1").then((cache) => {
return cache.addAll(["/", "/index.html", "/styles.css", "/app.js"]);
})
);
});
self.addEventListener("fetch", (event) => {
event.respondWith(
caches.match(event.request).then((response) => {
return response || fetch(event.request);
})
);
});
In your app.js, register the service worker.
app.js
if ("serviceWorker" in navigator) {
window.addEventListener("load", () => {
navigator.serviceWorker.register("/service-worker.js").then(
(registration) => {
console.log(
"ServiceWorker registration successful with scope: ",
registration.scope
);
},
(error) => {
console.log("ServiceWorker registration failed: ", error);
}
);
});
}
Push Notifications: PWAs can send push notifications to re-engage users.
advertisement
Step 3: Adding a Web App Manifest
A web app manifest provides metadata about your app and allows users to install it on their home screen.
mainifest.json
{
"name": "My First PWA",
"short_name": "PWA",
"start_url": "/index.html",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
Link the manifest in your HTML file.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>My First PWA</title>
<link rel="stylesheet" href="styles.css" />
<link rel="manifest" href="/manifest.json" />
</head>
<body>
<h1>Welcome to My First PWA</h1>
<p>This is a simple Progressive Web App.</p>
<script src="app.js"></script>
</body>
</html>
Ensure you have the necessary icons (icon-192x192.png and icon-512x512.png) in your project directory.
Step 4: Testing Your PWA
To test your PWA, you can use the "Live Server" extension in Visual Studio Code (VSCode). This extension allows you to launch a local development server with a live reload feature, which is perfect for testing your PWA.
- Install Live Server Extension in VSCode:
- Open VSCode.
- Go to the Extensions view by clicking the Extensions icon in the Activity Bar on the side of the window or by pressing Ctrl+Shift+X.
- Search for "Live Server" and install it.
-
Open your project folder in VSCode.
-
Start Live Server:
- Right-click on your index.html file and select "Open with Live Server."
- This will start a local server and open your application in the browser.
Your app should be fully functional, and you should see the option to install it as a PWA.
FAQ
Q: What browsers support PWAs? A: Most modern browsers including Chrome, Firefox, Safari, and Edge support PWAs.
Q: Can PWAs access device features? A: Yes, PWAs can access various device features such as camera, geolocation, and push notifications through web APIs.
Q: Are PWAs secure? A: PWAs require HTTPS, ensuring that all data is transmitted securely.
Q: How do I distribute a PWA? A: PWAs can be accessed via a URL and installed directly from the browser. They can also be published in app stores.
Automatic Updates: Service workers update in the background, ensuring users always have the latest version.
Conclusion
Congratulations! You've built your first Progressive Web App. By following these steps, you’ve created a reliable, fast, and engaging web app that provides an excellent user experience. Continue exploring PWAs to unlock their full potential and provide even more value to your users. Don't forget to share your experience and any additional tips in the comments below!
Complete Files for Your First PWA
Here are the complete versions of the files you'll need:
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>My First PWA</title>
<link rel="stylesheet" href="styles.css" />
<link rel="manifest" href="/manifest.json" />
</head>
<body>
<h1>Welcome to My First PWA</h1>
<p>This is a simple Progressive Web App.</p>
<script src="app.js"></script>
</body>
</html>
styles.css
body {
font-family: Arial, sans-serif;
text-align: center;
margin-top: 50px;
}
h1 {
color: #333;
}
p {
color: #666;
}
app.js
if ("serviceWorker" in navigator) {
window.addEventListener("load", () => {
navigator.serviceWorker.register("/service-worker.js").then(
(registration) => {
console.log(
"ServiceWorker registration successful with scope: ",
registration.scope
);
},
(error) => {
console.log("ServiceWorker registration failed: ", error);
}
);
});
}
service-worker.js
self.addEventListener("install", (event) => {
event.waitUntil(
caches.open("v1").then((cache) => {
return cache.addAll(["/", "/index.html", "/styles.css", "/app.js"]);
})
);
});
self.addEventListener("fetch", (event) => {
event.respondWith(
caches.match(event.request).then((response) => {
return response || fetch(event.request);
})
);
});
manifest.json
{
"name": "My First PWA",
"short_name": "PWA",
"start_url": "/index.html",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
App-like Experience: PWAs provide a full-screen experience and can be launched from the home screen without a browser.
advertisement
Related Articles
About the Author
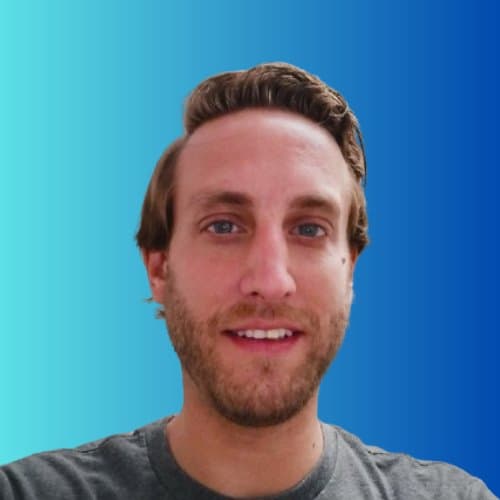
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All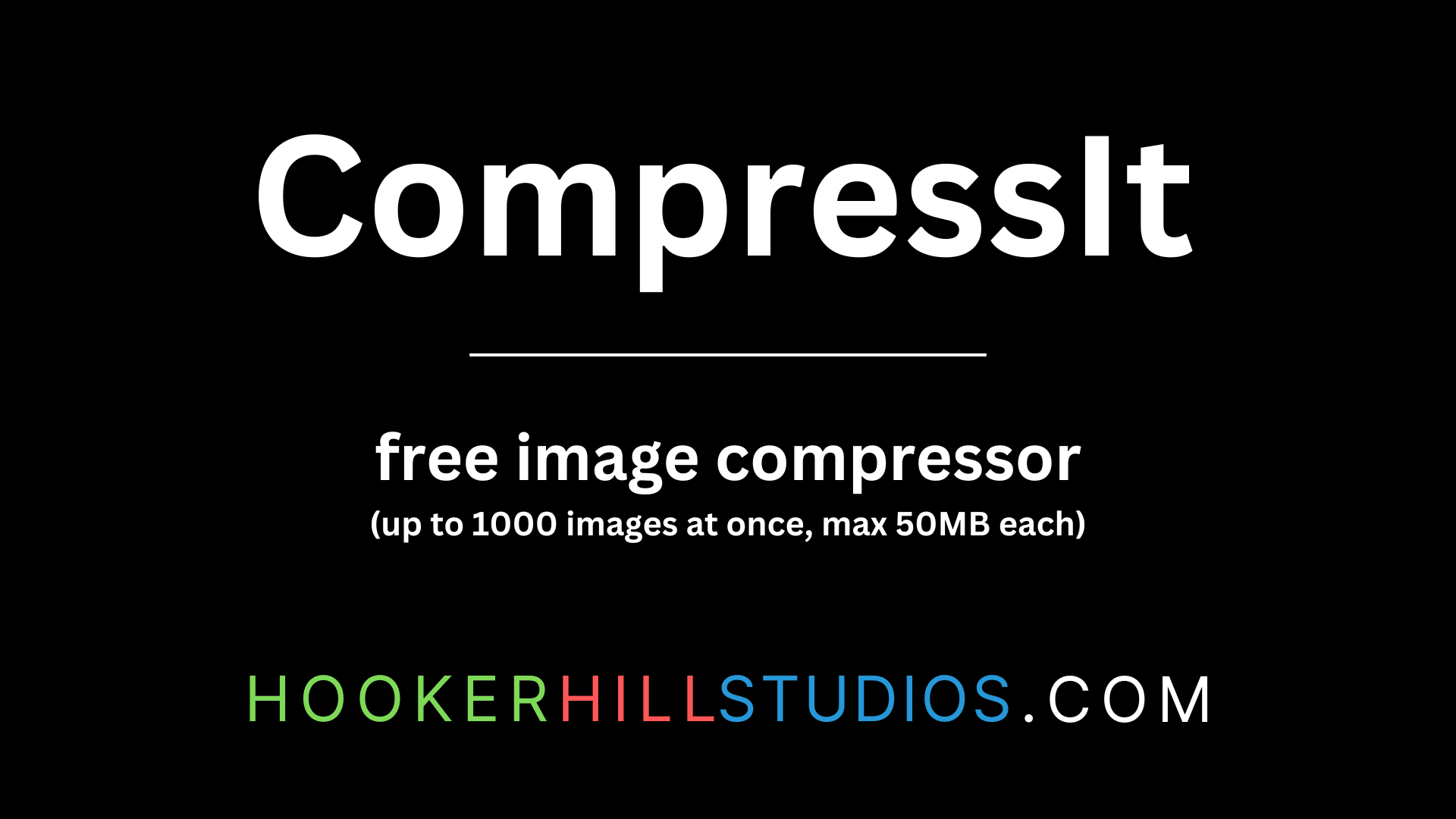
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024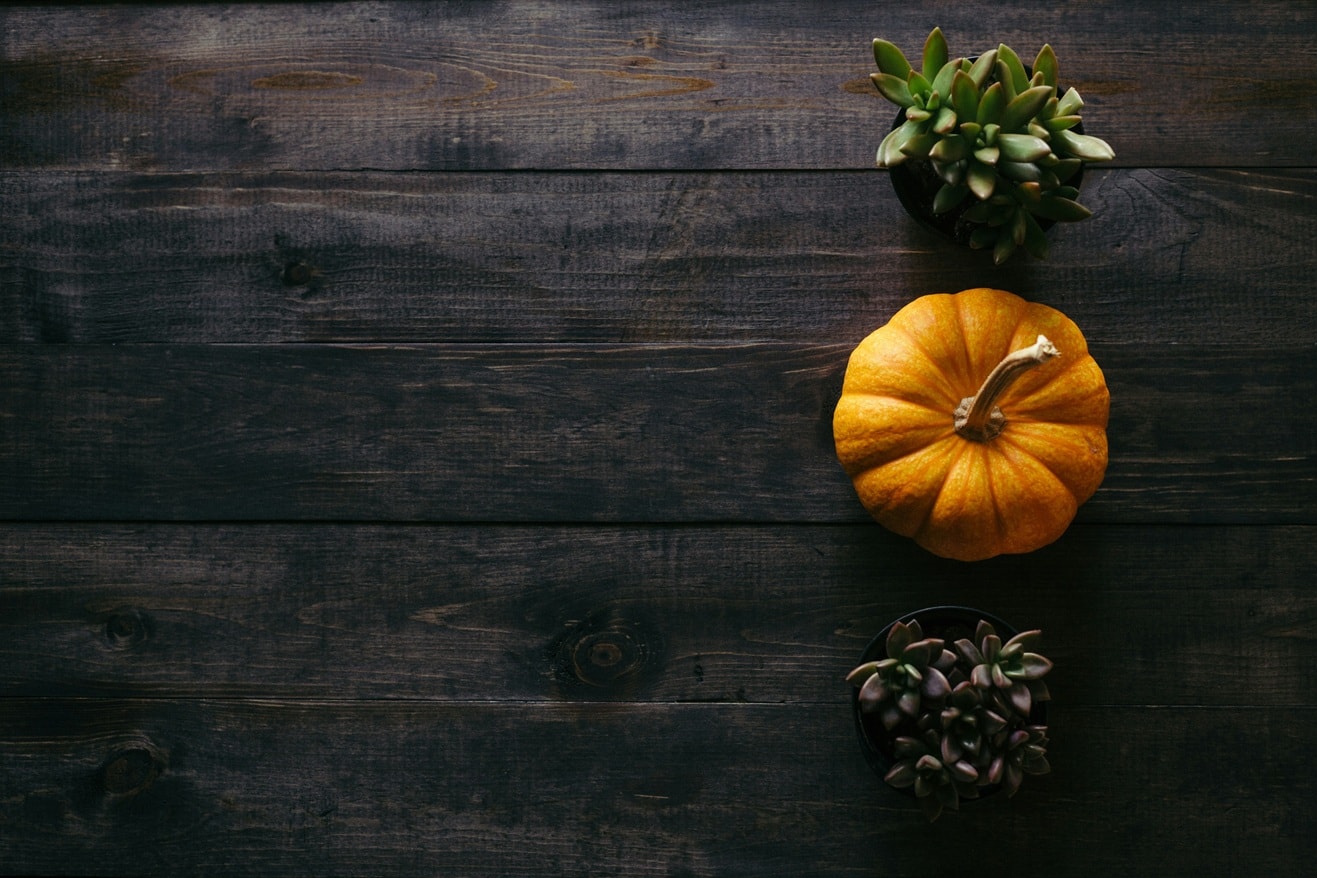
Comments
to join the conversation
Loading comments...