Building Progressive Web Apps with Next.js: A Complete Guide
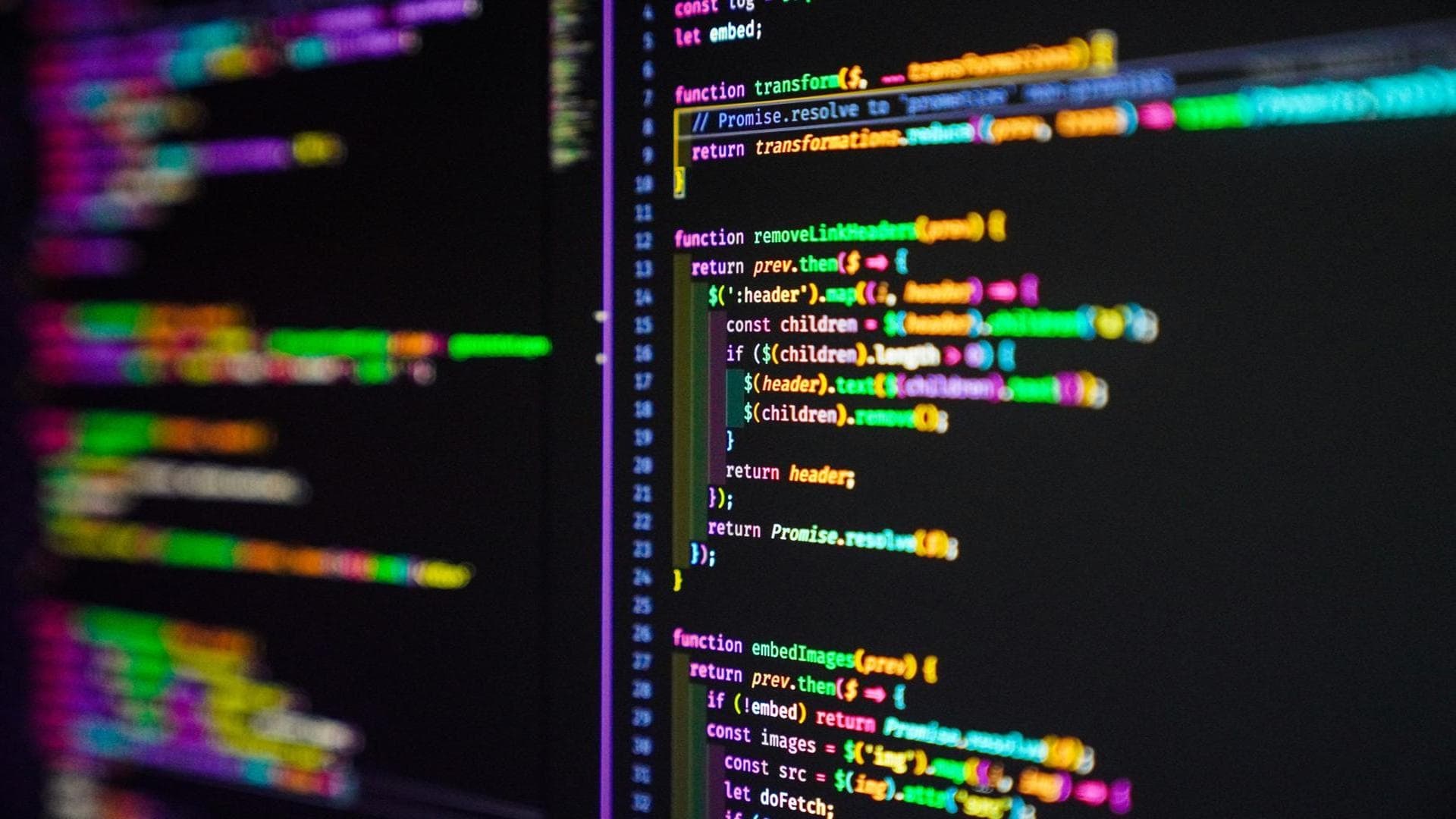
Table Of Contents
Progressive Web Apps (PWAs) offer a compelling way to deliver a native app-like experience on the web. They combine the best of web and mobile apps, providing users with a fast, reliable, and engaging experience. In this post, we'll explore how to create a PWA using Next.js, a popular React framework.
What is a PWA?
A Progressive Web App is a web application that uses modern web capabilities to deliver an app-like experience to users. Key features of PWAs include:
- Offline Support: PWAs can work offline or on low-quality networks.
- App-like Experience: PWAs feel like a native app to the user with app-style interactions and navigation.
- Installable: Users can add PWAs to their home screens without going through an app store.
- Push Notifications: PWAs can send push notifications to keep users engaged.
Setting Up a Next.js Project
To get started, you'll need to set up a new Next.js project. If you don't already have Next.js installed, you can create a new project using the following command:
npm create-next-app my-pwa
cd my-pwa
Configuring the Manifest File
A web app manifest is a JSON file that defines the appearance and behavior of your PWA when it's installed on a user's device. Create a public/manifest.json file with the following content:
{
"name": "My PWA",
"short_name": "PWA",
"description": "My Progressive Web App",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "/icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "/icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
Add the following link tag to your apps layout.js file to reference the manifest file:
<Head>
<link rel="manifest" href="/manifest.json" />
</Head>
advertisement
Adding a Service Worker
Service workers are scripts that run in the background and provide features like offline support, background sync, and push notifications. To add a service worker to your Next.js project, you can use the next-pwa plugin.
First, install the 'next-pwa' package:
npm install next-pwa
Then, create or update the 'next.config.js' file to configure the 'next-pwa' plugin:
const withPWA = require("next-pwa");
module.exports = withPWA({
pwa: {
dest: "public",
register: true,
skipWaiting: true,
},
});
Next, create a 'public/sw.js' file for the service worker. For a basic setup, you can use the following content:
self.addEventListener("install", (event) => {
console.log("Service Worker installing.");
});
self.addEventListener("activate", (event) => {
console.log("Service Worker activating.");
});
self.addEventListener("fetch", (event) => {
event.respondWith(fetch(event.request));
});
Improving Performance and User Experience
Lazy Loading
Lazy loading allows you to load components only when they are needed, reducing the initial load time and improving performance.
Code Splitting
Code splitting helps in breaking down your code into smaller chunks, which can be loaded on demand. This improves the initial load time and makes your app more performant.
Optimizing Images
Images often contribute to the bulk of the download size for web apps. Using modern image formats like WebP, compressing images, and serving them at appropriate sizes can significantly improve load times.
Caching Strategies
Caching is crucial for PWAs to work offline and load quickly. Service workers provide powerful caching strategies.
Deploying Your PWA
Deploying a PWA involves serving your web app over HTTPS, ensuring your service worker is registered, and the manifest file is correctly configured.
Hosting Options
Several hosting services are well-suited for deploying PWAs, including Vercel, Netlify, and GitHub Pages.
Continuous Deployment
Setting up continuous deployment ensures that your PWA is always up-to-date with the latest changes.
advertisement
More JavaScript Articles:
-
Embracing JavaScript: Unveiling the Strengths Beyond Traditional Languages
-
Create a Customizable and Reusable React Hook for Using Local Storage: A Step-by-Step Tutorial
-
Comparing Modern JavaScript Frameworks: React, Angular, and Vue in 2024
Conclusion
Progressive Web Apps offer a compelling way to build fast, reliable, and engaging web experiences. With Next.js, you have the tools to create a PWA that delivers a native app-like experience. By leveraging service workers, optimizing performance, and utilizing modern web features, you can create PWAs that work seamlessly across devices and provide a delightful user experience.
In this post, we've covered the key concepts behind PWAs, including offline support, app-like experiences, and installability. We've also walked through the process of setting up a Next.js project, configuring the manifest file, adding a service worker, and optimizing performance.
With the knowledge gained from this post, you're well-equipped to create your own Progressive Web Apps using Next.js. Whether you're building a simple blog or a complex web application, PWAs can help you deliver a better experience to your users.
About the Author
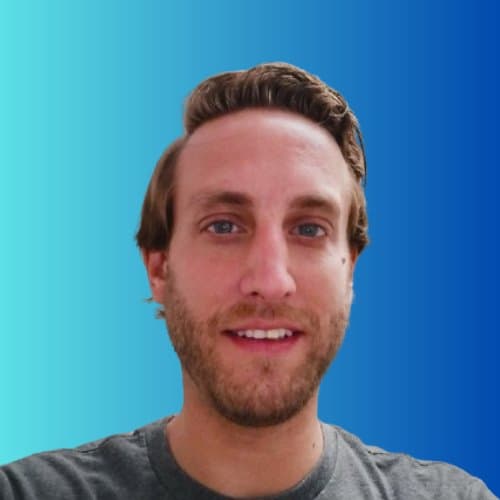
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All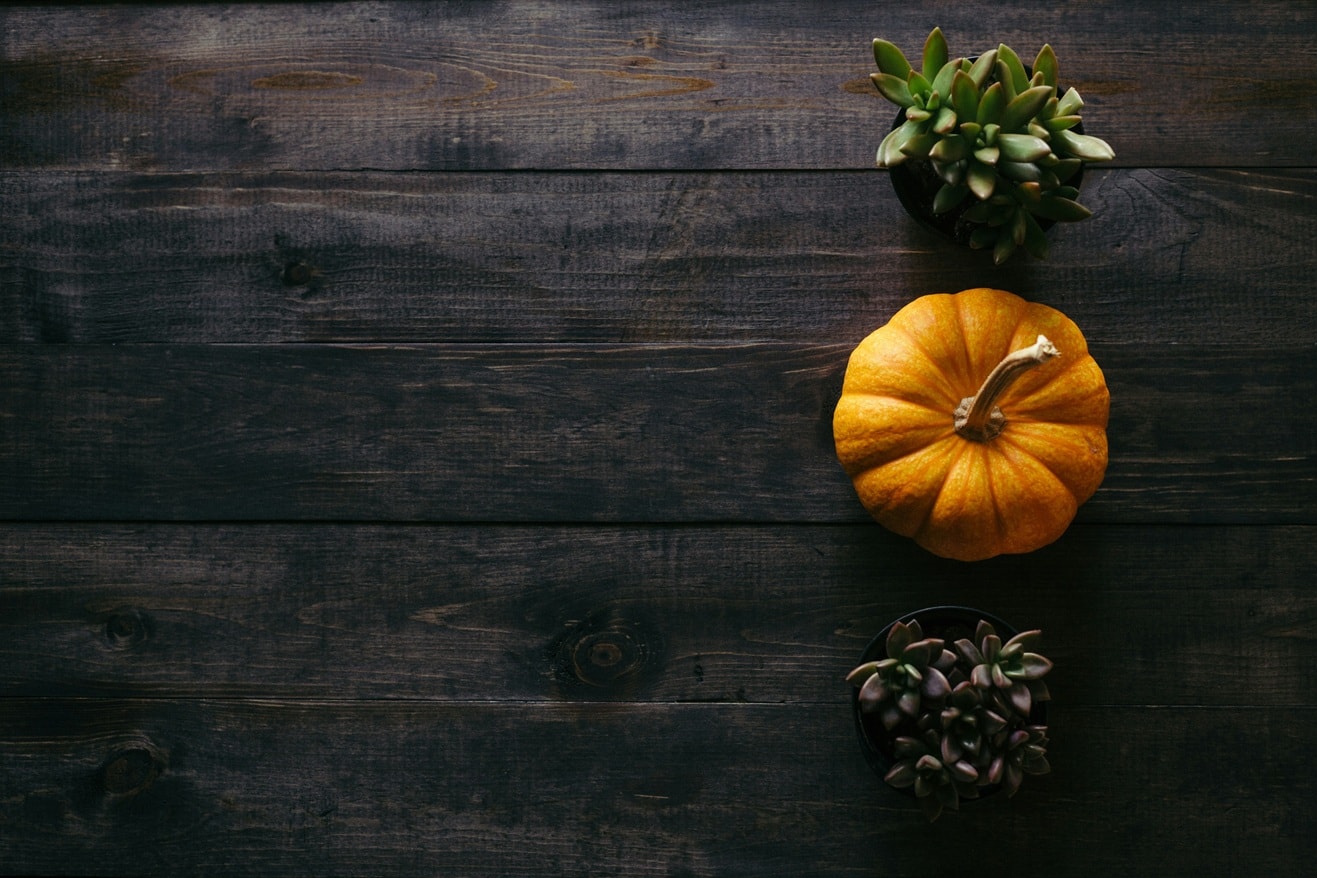
Software Development Services in Redlands: Powered by Hooker Hill Studios
November 20, 2024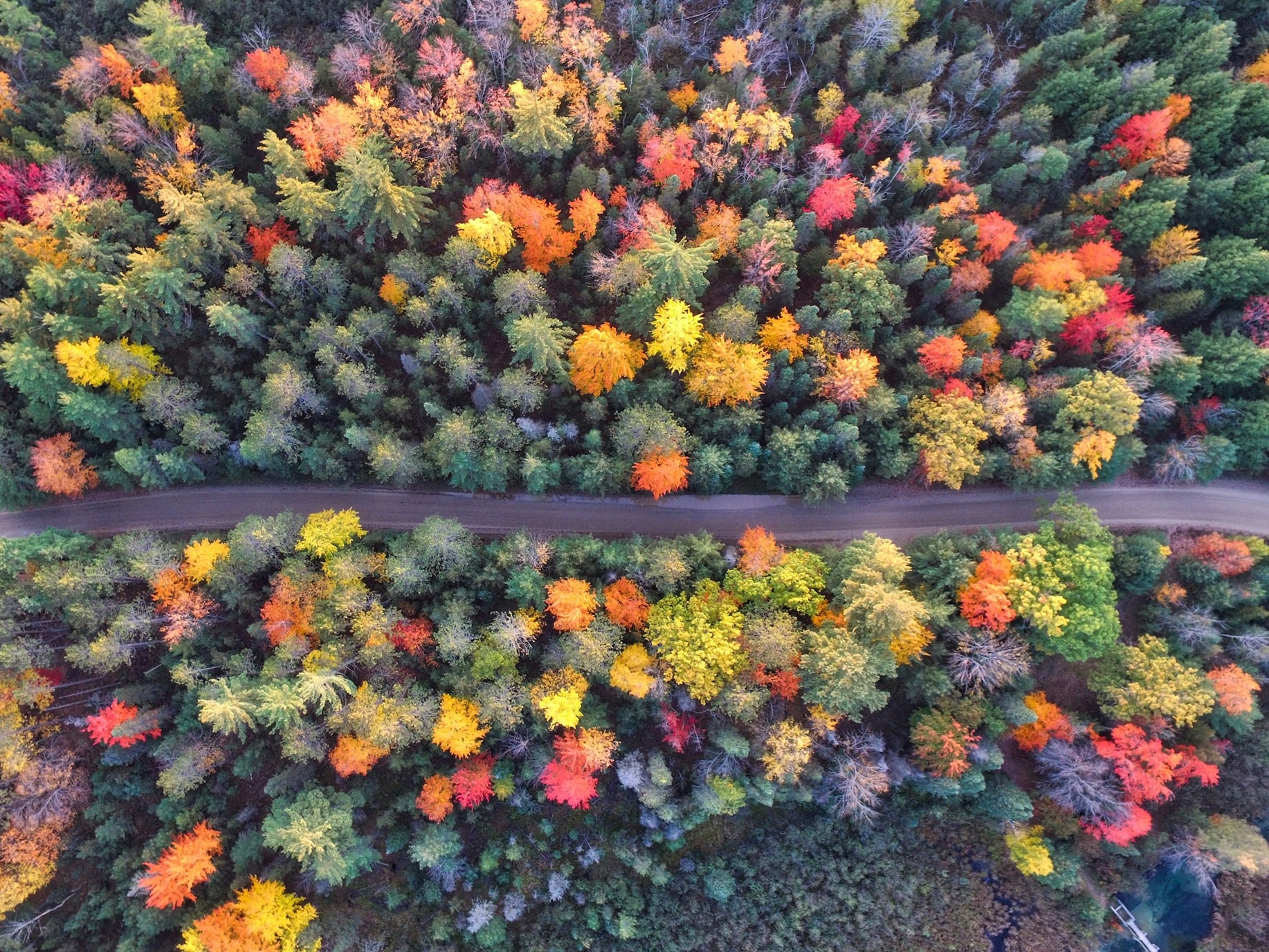
Comments
to join the conversation
Loading comments...