How to Create Page Transition Animations in Next.js Apps: A Step-by-Step Tutorial
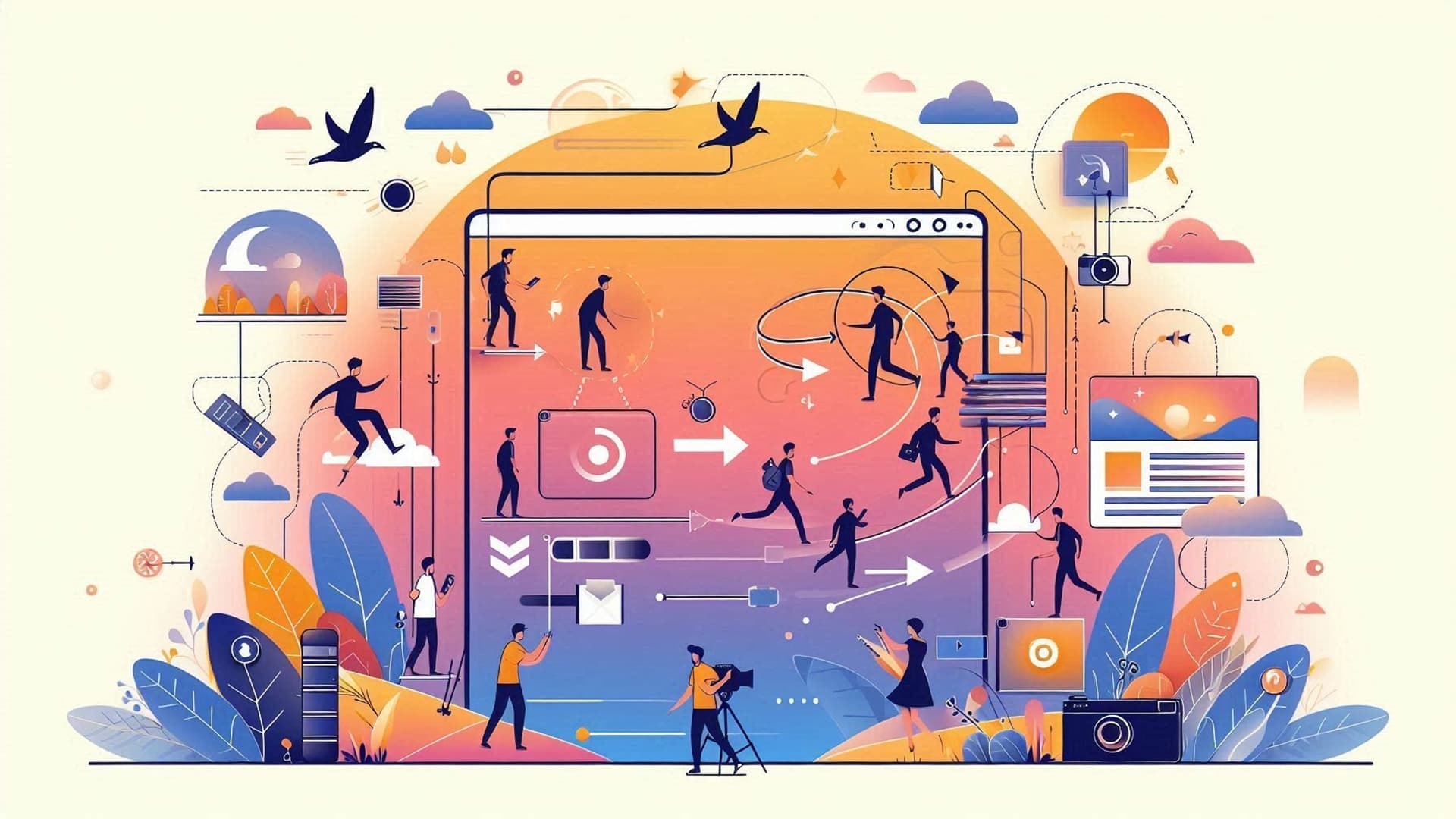
Introduction
Adding page transitions can significantly enhance the user experience by making navigation feel smoother and more intuitive. This guide will show you how to implement a reusable TransitionLink
component in a Next.js application, allowing you to apply page transitions to any link in your project.
1. Setting Up the Project
First, ensure you have a Next.js project set up. If you don't already have one, you can create it with the following command:
npx create-next-app@latest --example with-typescript --ts
Install any dependencies if needed, and make sure your project is running smoothly by using:
npm run dev
The TransitionLink component can be extended to support more complex animations and interactions.
2. Creating the TransitionLink
Component
We'll create a custom TransitionLink
component that wraps the Next.js Link
component, adding transition effects before navigating to the new page.
Create a file named TransitionLink.tsx
in the utils
directory (or any directory you prefer) and add the following code:
'use client'
import Link, { LinkProps } from 'next/link'
import React, { ReactNode } from 'react'
import { useRouter } from 'next/navigation'
interface TransitionLinkProps extends LinkProps {
children: ReactNode;
href: string;
onClick?: (e: React.MouseEvent<HTMLAnchorElement, MouseEvent>) => void;
}
function sleep(ms: number): Promise<void> {
return new Promise(resolve => setTimeout(resolve, ms))
}
export const TransitionLink = ({
children,
href,
onClick,
...props
}: TransitionLinkProps) => {
const router = useRouter()
const handleTransition = async (e: React.MouseEvent<HTMLAnchorElement, MouseEvent>) => {
e.preventDefault()
// Call the custom onClick handler if provided
if (onClick) {
onClick(e)
}
const body = document.querySelector('body')
body?.classList.add('page-transition')
await sleep(500)
router.push(href)
await sleep(500)
body?.classList.remove('page-transition')
}
return (
<Link
onClick={handleTransition}
href={href} {...props}>
{children}
</Link >
)
}
advertisement
Explanation:
sleep(ms: number)
: A helper function that pauses the execution for a specified number of milliseconds. This allows us to control the timing of our transitions.
handleTransition function
: This function first prevents the default link behavior, applies the transition effect by adding the page-transition
class to the body, and then uses the useRouter
hook to navigate to the new page after a delay.
TransitionLink
component: This is a wrapper around the Next.js Link
component. It handles the transition effect when the link is clicked, making navigation feel more fluid.
CSS transitions are hardware-accelerated, making them more efficient than JavaScript-based animations.
3. Applying the Transitions
Next, we'll define the CSS for the transition effects. In your global CSS file (usually globals.css
or styles.css
), add the necessary styles for the transitions:
body {
transition-property: opacity, background, transform, filter;
transition-timing-function: ease-in-out;
transition-duration: 500ms;
}
.page-transition {
opacity: 0;
background: black;
transform: translateY(40px);
filter: blur(12px);
}
Explanation:
body
: We define the properties that will transition, such as opacity, background, transform, and filter. The duration and timing function control how the transition feels.
.page-transition
: This class is applied during the transition, causing the page to fade out, move slightly down, and blur, while the background changes to black.
4. Integrating the TransitionLink
Component
You can now use the TransitionLink
component in your pages. Here’s how to integrate it into your navigation menu or anywhere you want to use transition links:
import { TransitionLink } from '../utils/TransitionLink';
<div className="hidden w-full md:flex flex-grow justify-around">
<TransitionLink href="/Services" className="nav-link hover:scale-95 hover:text-gray-800 transition-transform text-gray-800 duration-300 cursor-pointer text-lg sm:text-2xl">
Services
</TransitionLink>
<TransitionLink href="/Products" className="nav-link hover:scale-95 hover:text-gray-800 transition-transform text-gray-800 duration-300 cursor-pointer text-lg sm:text-2xl">
Products
</TransitionLink>
<TransitionLink href="/Contact" className="nav-link hover:scale-95 hover:text-gray-800 transition-transform text-gray-800 duration-300 cursor-pointer text-lg sm:text-2xl">
Contact
</TransitionLink>
<TransitionLink href="/Quote" className="nav-link hover:scale-95 hover:text-gray-800 transition-transform text-gray-800 duration-300 cursor-pointer text-lg sm:text-2xl">
Quote
</TransitionLink>
<TransitionLink href="/Blog" className="nav-link hover:scale-95 hover:text-gray-800 transition-transform text-gray-800 duration-300 cursor-pointer text-lg sm:text-2xl">
Blog
</TransitionLink>
{isAdmin && (
<TransitionLink
href="/Admin"
className="nav-link hover:scale-95 hover:text-gray-800 transition-transform text-emerald-700 duration-300 cursor-pointer text-lg sm:text-2xl"
>
Admin
</TransitionLink>
)}
</div>
The
useRouter
hook in Next.js provides powerful programmatic navigation capabilities.
advertisement
5. FAQ
How can I adjust the transition duration?
You can adjust the transition duration by changing the value in the transition-duration
property in the CSS.
transition-duration: 700ms;
Can I customize the transition effects?
Absolutely! You can modify the properties in the .page-transition
class to achieve different effects.
Will this work on all pages?
Yes, as long as the TransitionLink
component is used, the transition effect will be applied when navigating to any page.
Is there any impact on SEO or accessibility? This method has minimal impact on SEO since it does not interfere with the actual navigation or content loading.
6. Resources
Next.js supports multiple types of rendering (Static, Server-Side, and Client-Side), making it versatile for building modern web applications.
7. Additonal Transition Effects:
Feel free to experiment with the transition animations. Try out the suggestions below or come up with your own. The only limits are the imagination!
/*
Slide in from right
*/
.page-transition {
opacity: 0;
transform: translateX(100%);
transition: transform 500ms ease-in-out, opacity 500ms ease-in-out;
}
/*
Zoom in with blur
*/
.page-transition {
opacity: 0;
transform: scale(1.1);
filter: blur(8px);
transition: transform 500ms ease-in-out, opacity 500ms ease-in-out, filter 500ms ease-in-out;
}
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Using transitions can improve the perceived performance of your application by making navigation feel smoother.
Conclusion
By following this guide, you’ve learned how to implement smooth page transitions in your Next.js application using a custom TransitionLink
component. This enhances the user experience by providing visually appealing navigation effects. Feel free to experiment with different styles and durations to match your site's aesthetics.
This approach is scalable, reusable, and easily customizable, making it a valuable addition to your Next.js toolkit.
About the Author
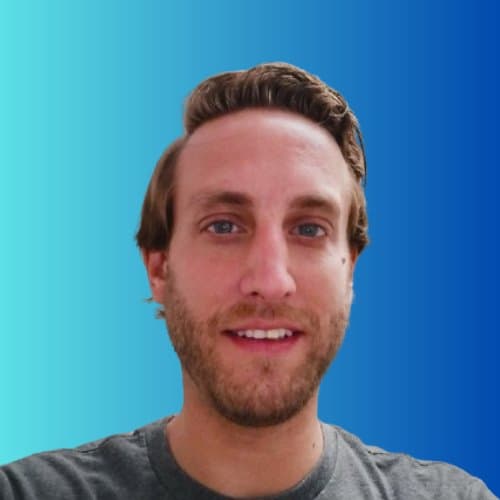
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All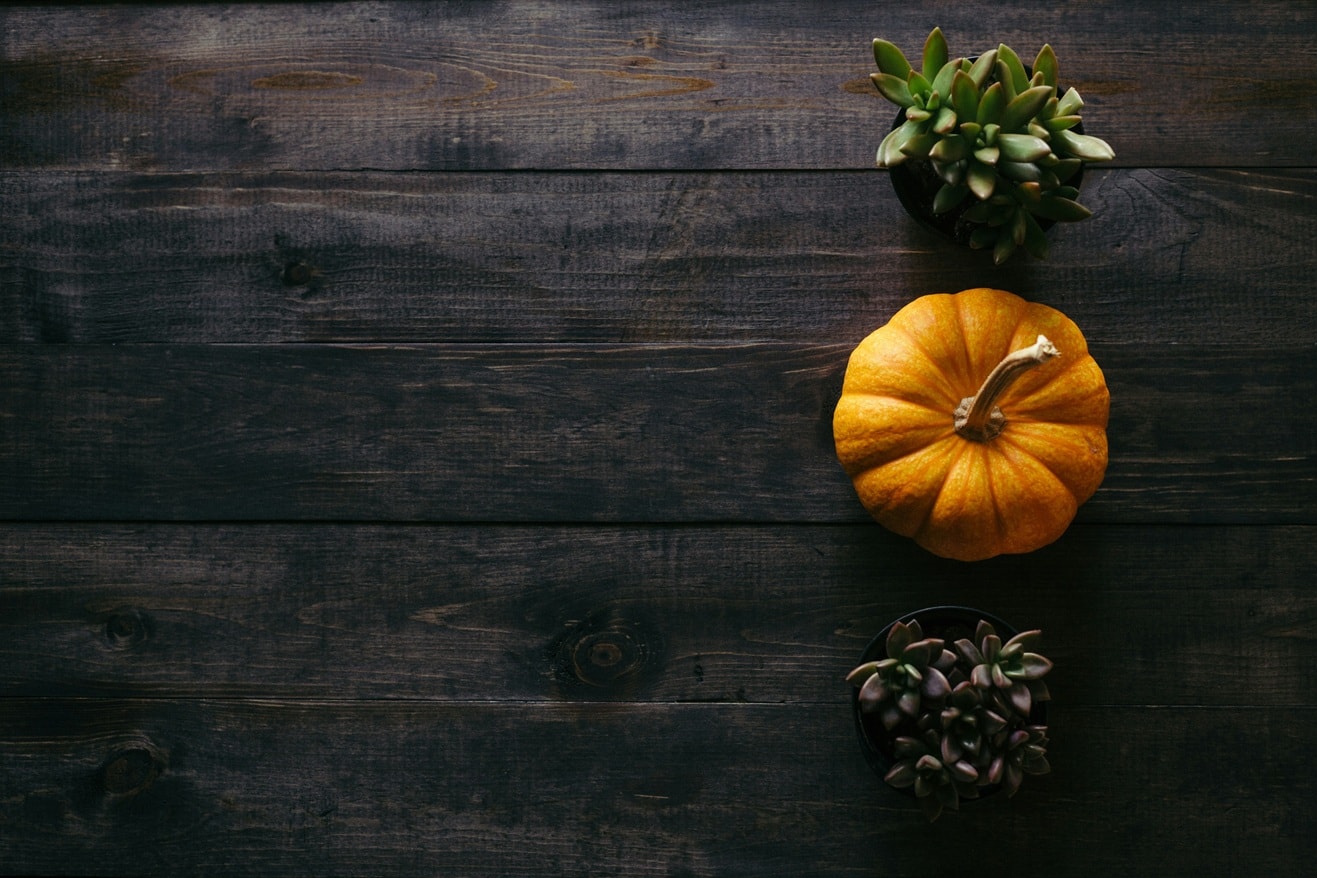
Software Development Services in Redlands: Powered by Hooker Hill Studios
November 20, 2024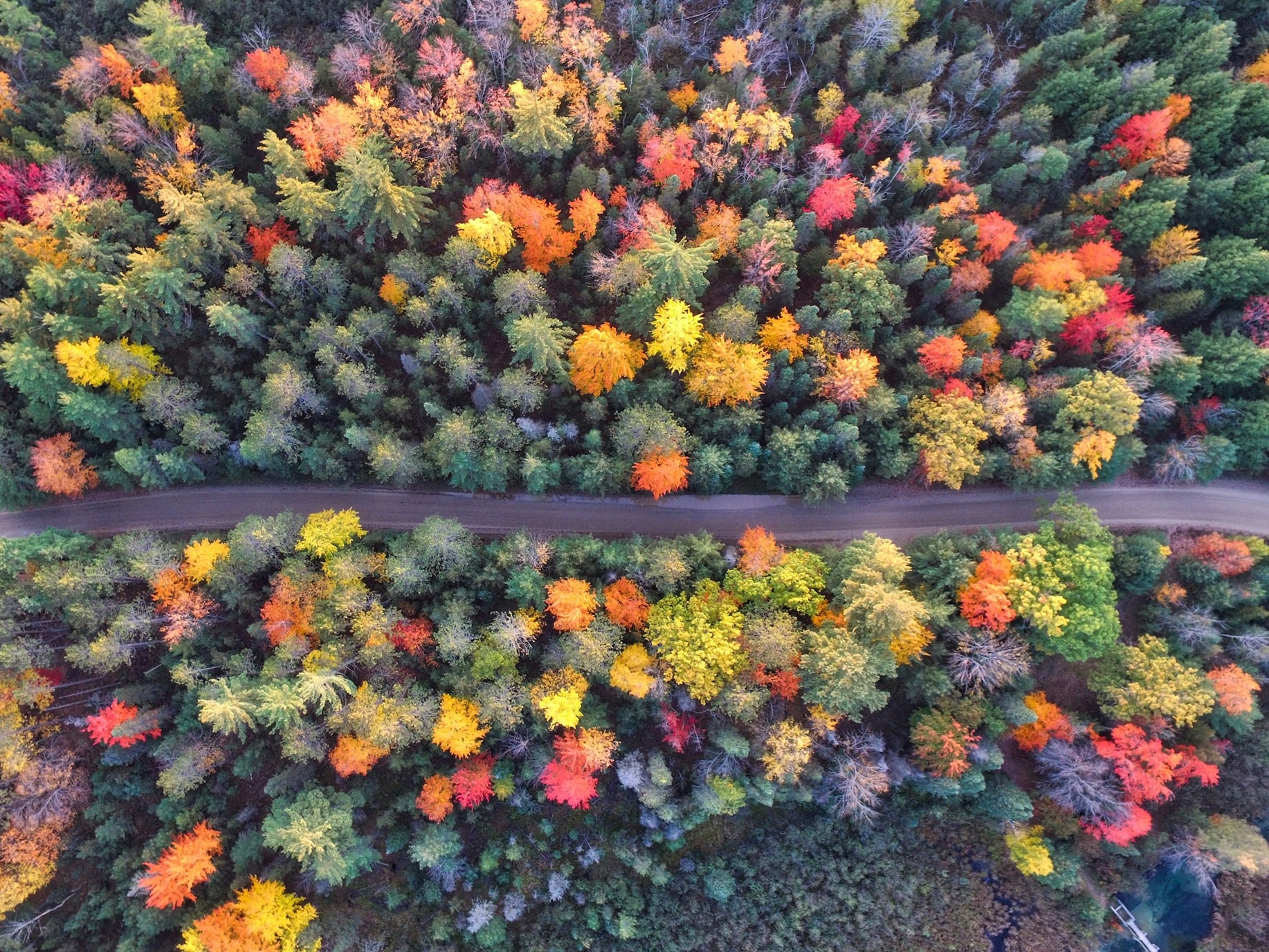
Comments
to join the conversation
Loading comments...