PyInstaller Guide: Create Standalone Python Executables
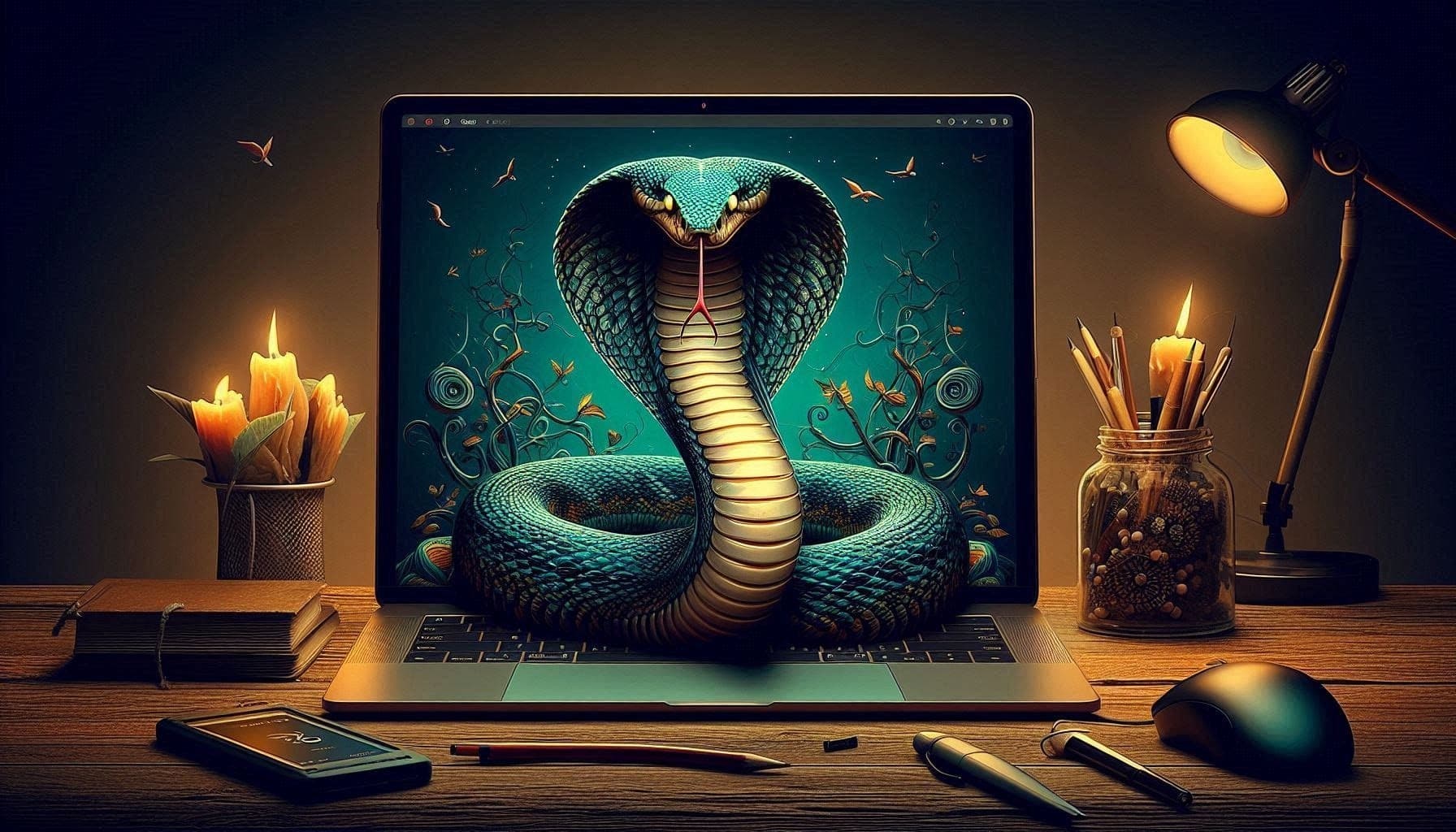
Table Of Contents
- What is PyInstaller?
- How to Install PyInstaller:
- Basic Usage:
- Advanced Usage and Optional Flags:
- Simplifying Complex PyInstaller Commands with Batch Files
- Step 1: Create a Batch File
- Step 2: Write Your PyInstaller Command
- Step 3: Save and Run
- Tips for Writing Batch Files
- Example Batch File
- @echo off
- Spec Files:
- -*- mode: python ; coding: utf-8 -*-
- To build using the spec file:
- Troubleshooting:
- Best Practices:
- Related Articles:
- Donate
- Conclusion
What is PyInstaller?
PyInstaller is a popular tool that converts Python applications into standalone executables. It bundles a Python application and all its dependencies into a single package, which can be distributed and run on systems without requiring Python to be installed.
Key features of PyInstaller:
- Cross-platform support (Windows, macOS, Linux)
- Works with Python 2.7 and 3.5+
- Automatic detection of dependencies
- Support for most popular Python packages
- Customizable through spec files
How to Install PyInstaller:
You can install PyInstaller using pip:
pip install pyinstaller
Basic Usage:
To create a basic executable from your Python script, use the following command:
pyinstaller your_script.py
This will create a dist
folder containing the executable and all necessary files.
Advanced Usage and Optional Flags:
- Single-file executable:
pyinstaller --onefile your_script.py
- Specify name for the output executable:
pyinstaller --name=MyApp your_script.py
- Add an icon (Windows and macOS):
pyinstaller --icon=path/to/icon.ico your_script.py
- Include additional files or folders:
pyinstaller --add-data "path/to/file:." your_script.py
- Exclude modules:
pyinstaller --exclude-module module_name your_script.py
- Hidden imports (for modules not automatically detected):
pyinstaller --hidden-import=module_name your_script.py
- Debug mode:
pyinstaller --debug your_script.py
advertisement
Simplifying Complex PyInstaller Commands with Batch Files
When working with PyInstaller, you may find yourself using multiple flags and options, resulting in long, complex commands. Creating a batch file can simplify this process, making your build commands more manageable and consistent. Here's a step-by-step guide on how to do this:
Step 1: Create a Batch File
- Open a text editor (like Notepad or Visual Studio Code).
- Create a new file.
- Save it with a
.bat
extension (e.g.,build_app.bat
).
Step 2: Write Your PyInstaller Command
In your batch file, write your PyInstaller command, using the ^
symbol to split it across multiple lines:
pyinstaller ^
--name="MyApp" ^
--onefile ^
--windowed ^
--icon=app_icon.ico ^
--add-data="resources;resources" ^
--splash=splash_image.png ^
main.py
Step 3: Save and Run
- Save your batch file.
- To run it, either:
- Double-click the
.bat
file in File Explorer, or - Open a command prompt, navigate to the directory containing the batch file, and type its name.
Tips for Writing Batch Files
- Use ^ at the end of each line to continue the command on the next line.
- Ensure there are no spaces after the ^ symbol.
- You can add comments in your batch file using REM or :: at the beginning of a line.
- To set environment variables or perform other operations before running PyInstaller, add those commands at the beginning of your batch file.
Example Batch File
### @echo off
REM This batch file builds our Python application using PyInstaller
REM Activate virtual environment (if you're using one)
call venv\Scripts\activate
REM Set any necessary environment variables
set PYTHONPATH=.
REM Run PyInstaller
pyinstaller ^
--name="MyAwesomeApp" ^
--onefile ^
--windowed ^
--icon=assets/icon.ico ^
--add-data="resources;resources" ^
--add-data="config.ini;." ^
--splash=assets/splash.png ^
--hidden-import=pyttsx3.drivers ^
--hidden-import=pyttsx3.drivers.sapi5 ^
src/main.py
REM Deactivate virtual environment
call deactivate
echo Build complete!
pause
By using a batch file, you can easily rerun complex PyInstaller commands, share your build configuration with team members, and maintain consistency in your build process.
Spec Files:
Spec files provide more control over the build process. To generate a spec file:
pyi-makespec your_script.py
This creates a your_script.spec
file. You can modify this file to customize the build process. Here's an example of a simple spec file:
# -*- mode: python ; coding: utf-8 -*-
block_cipher = None
a = Analysis(['your_script.py'],
pathex=['/path/to/your/script'],
binaries=[],
datas=[],
hiddenimports=[],
hookspath=[],
runtime_hooks=[],
excludes=[],
win_no_prefer_redirects=False,
win_private_assemblies=False,
cipher=block_cipher,
noarchive=False)
pyz = PYZ(a.pure, a.zipped_data,
cipher=block_cipher)
exe = EXE(pyz,
a.scripts,
[],
exclude_binaries=True,
name='your_script',
debug=False,
bootloader_ignore_signals=False,
strip=False,
upx=True,
console=True )
coll = COLLECT(exe,
a.binaries,
a.zipfiles,
a.datas,
strip=False,
upx=True,
upx_exclude=[],
name='your_script')
To build using the spec file:
pyinstaller your_script.spec
advertisement
Troubleshooting:
- Missing modules: Use
--hidden-import
to include modules that aren't automatically detected. - File not found errors: Use
--add-data
to include necessary data files. - Anti-virus false positives: Some antivirus software may flag PyInstaller executables. You may need to add exceptions or use code signing.
Best Practices:
- Always test your executable on a clean system to ensure all dependencies are included.
- Use virtual environments to isolate your project dependencies.
- Keep your Python environment up-to-date, including PyInstaller.
- For complex applications, use spec files for better control over the build process.
- Consider using
--windowed
or--noconsole
for GUI applications to hide the console window.
Related Articles:
Explore these articles to learn more about Python, PyInstaller, and Image Compression with Python:
- yt-dlp GUI and PyInstaller Tutorial
- Exploring Image Compression with Python: Libraries, Applications, and Use Cases
- Automating Image Compression and Resizing in Python: A Recursive Approach
- Packaging Python Applications with PyInstaller
- All Python Articles
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Conclusion
This guide covers the main aspects of using PyInstaller. Remember that PyInstaller is actively developed, so it's a good idea to check the official documentation for the most up-to-date information and advanced features.
About the Author
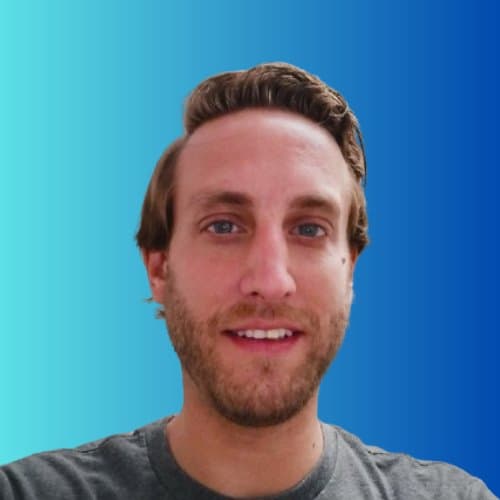
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All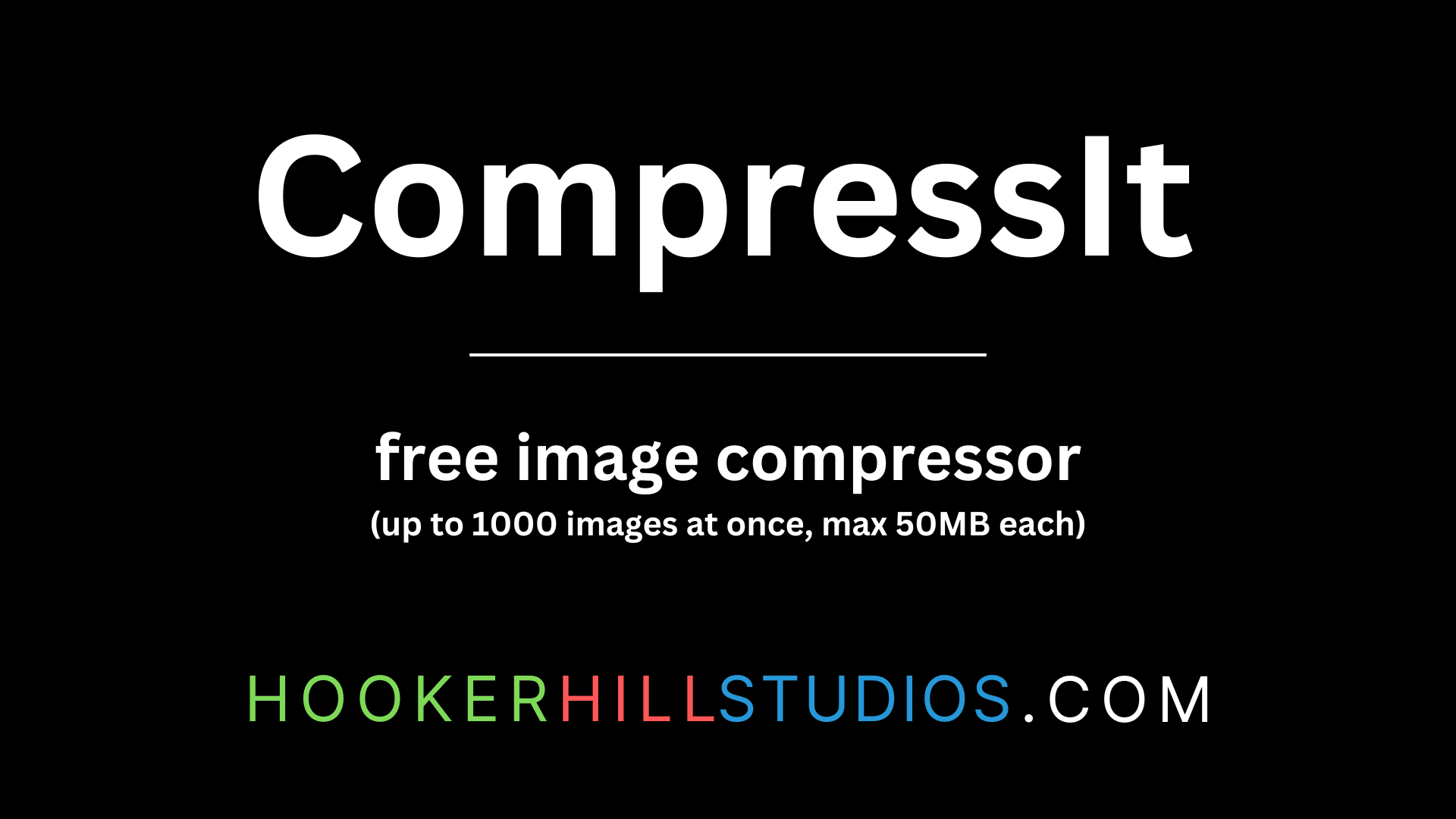
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024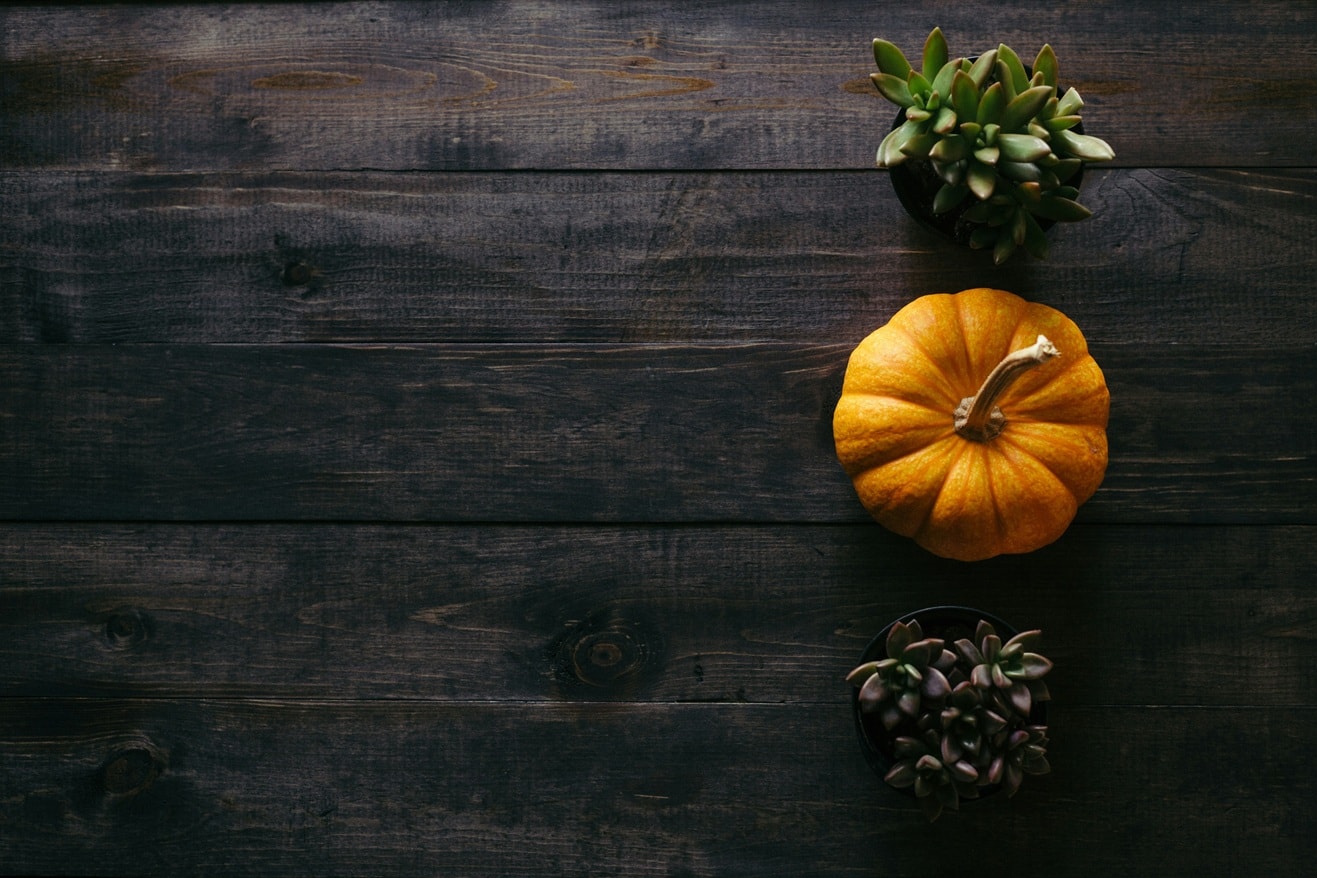
to join the conversation