Deploying a Flask App to Google Cloud Run
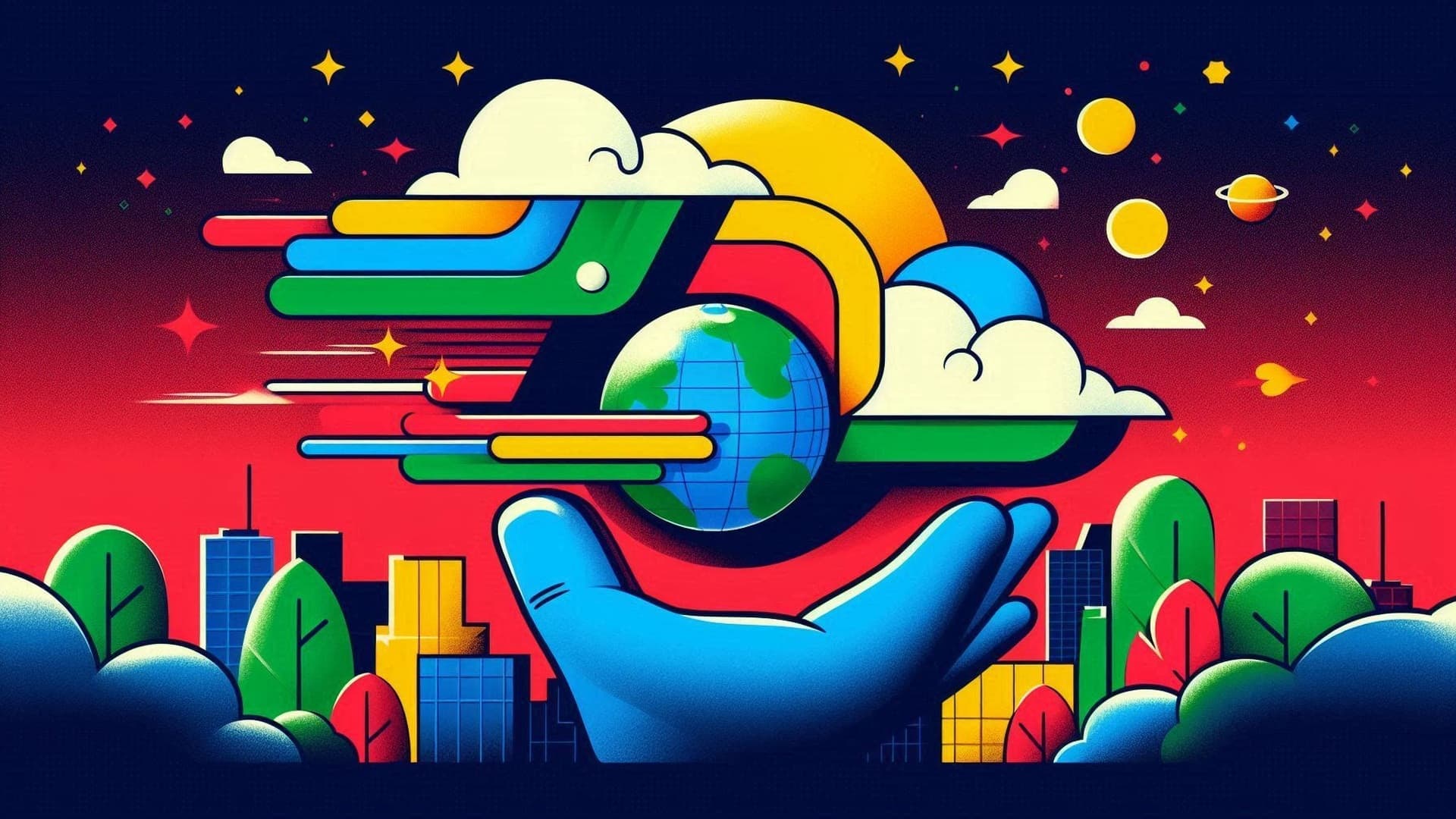
Table Of Contents
- Deploying a Flask App to Google Cloud Run
- Prerequisites
- Step-by-Step Guide
- 1. Create Project Directory
- 2. Set Up a Virtual Environment
- 3. Install Flask
- 4. Create the Flask App
- 5. Install Additional Dependencies
- 6. Create a .env File
- 7. Update main.py
- Load environment variables from .env file
- Configure FLASK_DEBUG from environment variable
- 8. Freeze Dependencies
- 9. Create app.yaml
- 10. Create Dockerfile
- Dockerfile
- Allow statements and log messages to immediately appear in the logs
- Copy local code to the container image.
- Run the web service on container startup. Here we use the gunicorn
- webserver, with one worker process and 8 threads.
- For environments with multiple CPU cores, increase the number of workers
- to be equal to the cores available.
- Timeout is set to 0 to disable the timeouts of the workers to allow Cloud Run to handle instance scaling.
- 11. Create a New Google Cloud Project
- 12. Initialize Google Cloud SDK
- 13. Deploy to Google Cloud Run
- Spinning down the server:
- Need more help?
- Related Articles
- Donate
- Conclusion
Deploying a Flask App to Google Cloud Run
In this tutorial, we will walk through the steps of creating a simple "Hello World" Flask application and deploying it to Google Cloud Run using Docker. This guide is specifically tailored for Windows users using Git Bash.
Prerequisites
Before you begin, ensure you have the following installed on your machine:
-
Python 3.9 or later
-
Git
-
Git Bash (comes with Git for Windows)
-
Google Cloud SDK
Step-by-Step Guide
1. Create Project Directory
First, create a new directory for your project and navigate into it.
mkdir hello-flask
cd hello-flask
2. Set Up a Virtual Environment
Create a virtual environment to manage your project's dependencies.
python -m venv env
Navigate into the env directory and activate the virtual environment.
cd env
source Scripts/activate
You should now see (env) at the beginning of your terminal prompt, indicating that the virtual environment is active.
3. Install Flask
Install Flask using pip.
pip install flask
Navigate back to your project root directory.
cd ..
4. Create the Flask App
Create a new file named app.py and add the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello World!"
if __name__ == '__main__':
app.run(debug=True)
5. Install Additional Dependencies
Install python-dotenv, Flask-CORS, and gunicorn.
pip install python-dotenv
pip install Flask-CORS
pip install gunicorn
6. Create a .env File
Create a .env file in the root directory of your project and add the following content:
FLASK_DEBUG=1
advertisement
7. Update main.py
Update the contents of app.py to include environment variable loading and CORS support:
import os
from flask import Flask
from dotenv import load_dotenv
from flask_cors import CORS
# Load environment variables from .env file
load_dotenv()
app = Flask(__name__)
CORS(app) # Enable CORS for all routes and origins
# Configure FLASK_DEBUG from environment variable
app.config['DEBUG'] = os.environ.get('FLASK_DEBUG')
@app.route('/')
def hello():
return "Hello World!"
if __name__ == '__main__':
app.run()
8. Freeze Dependencies
Generate a requirements.txt file with all the necessary dependencies.
pip freeze > requirements.txt
9. Create app.yaml
Create an app.yaml file with the following content:
runtime: python39
entrypoint: gunicorn -b :$PORT app:app
10. Create Dockerfile
Create a Dockerfile with the following content, be sure to use a capital 'D' in Dockerfile and do not assign any file extension:
# Dockerfile
FROM python:3.9.17-bookworm
# Allow statements and log messages to immediately appear in the logs
ENV PYTHONUNBUFFERED True
# Copy local code to the container image.
ENV APP_HOME /back-end
WORKDIR $APP_HOME
COPY . ./
RUN pip install --no-cache-dir --upgrade pip
RUN pip install --no-cache-dir -r requirements.txt
# Run the web service on container startup. Here we use the gunicorn
# webserver, with one worker process and 8 threads.
# For environments with multiple CPU cores, increase the number of workers
# to be equal to the cores available.
# Timeout is set to 0 to disable the timeouts of the workers to allow Cloud Run to handle instance scaling.
CMD exec gunicorn --bind :$PORT --workers 1 --threads 8 --timeout 0 app:app
11. Create a New Google Cloud Project
In your browser, visit the Google Cloud Console (https://console.cloud.google.com/) and create a new project.
12. Initialize Google Cloud SDK
If you haven't already installed the Google Cloud SDK:
(New-Object Net.WebClient).DownloadFile("https://dl.google.com/dl/cloudsdk/channels/rapid/GoogleCloudSDKInstaller.exe", "$env:Temp\GoogleCloudSDKInstaller.exe")
& $env:Temp\GoogleCloudSDKInstaller.exe
After installation, initialize it with the following command:
gcloud init
advertisement
Follow the prompts to sign in and select the project you just created.
13. Deploy to Google Cloud Run
Deploy your application to Google Cloud Run:
gcloud run deploy --source .
Follow the prompts to specify a service name, choose a server region, and allow unauthenticated invocations. Your application should now be deployed, and you'll get a URL where your app is hosted.
Spinning down the server:
Make sure to replace [project-id] with your projects id and [region] with the region you selected earlier for your server.
gcloud run services delete [project-id] --region=[region]
OR
Visit https://console.cloud.google.com/run and select the project you wish to delete.
Need more help?
If you are having issues successfully deploying your flask app to Google Cloud Run, feel free to download the complete files for this tutorial by clicking on the link below:
Download Complete Files
Related Articles
- Exploring Image Compression with Python: Libraries, Applications, and Use Cases
- Automating Image Compression and Resizing in Python: A Recursive Approach
- Packaging Python Applications with PyInstaller
- All Python Articles
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Conclusion
Congratulations! You have successfully created and deployed a "Hello World" Flask app to Google Cloud Run. You can now access your app via the URL provided by Google Cloud Run.
Feel free to customize your Flask application further and explore the various features offered by Google Cloud Run.
advertisement
About the Author
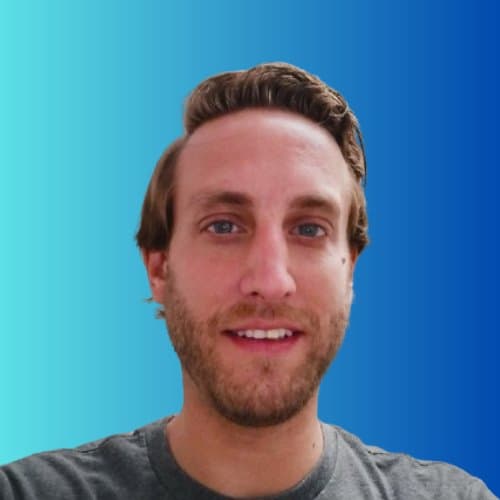
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All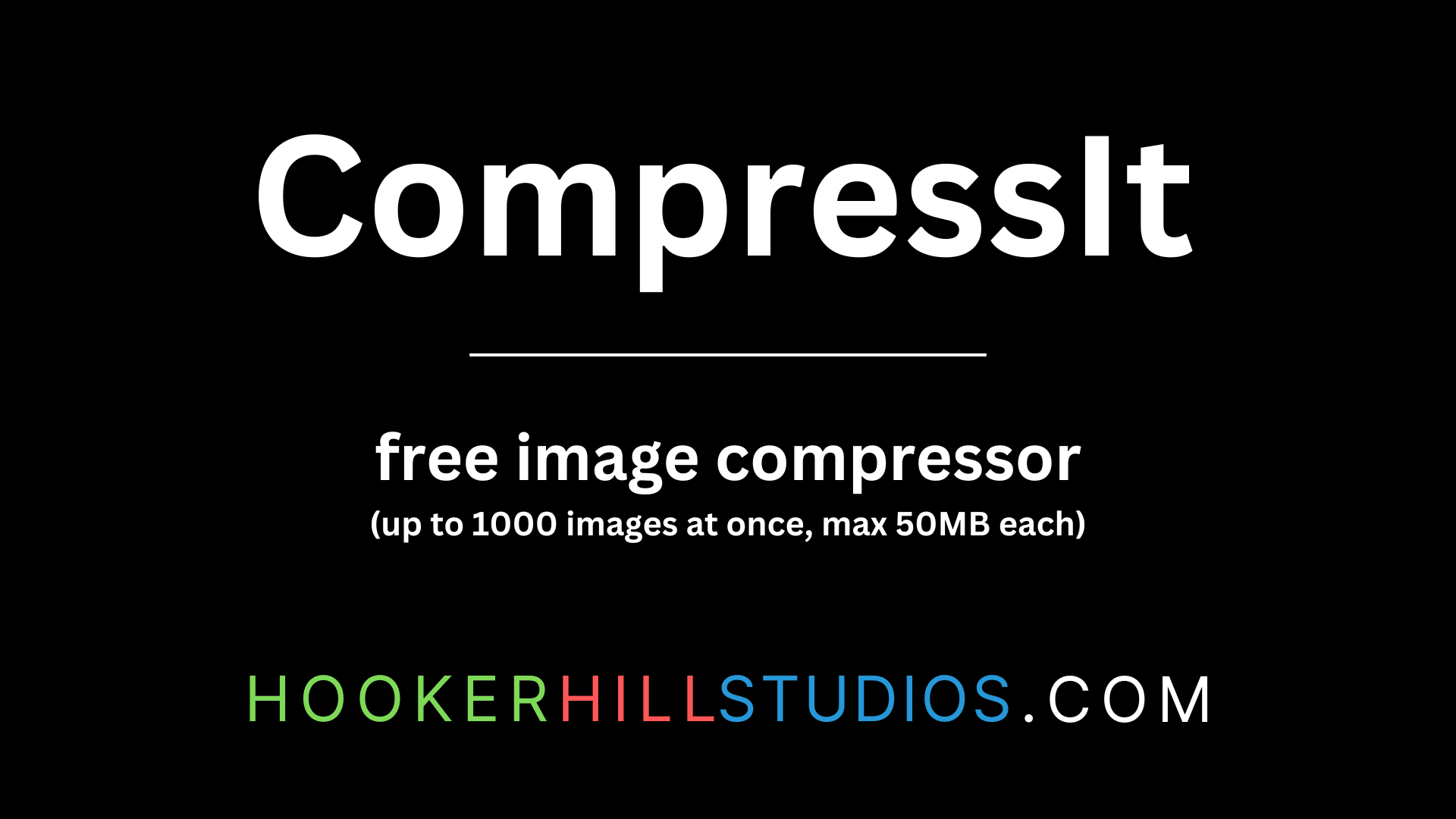
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024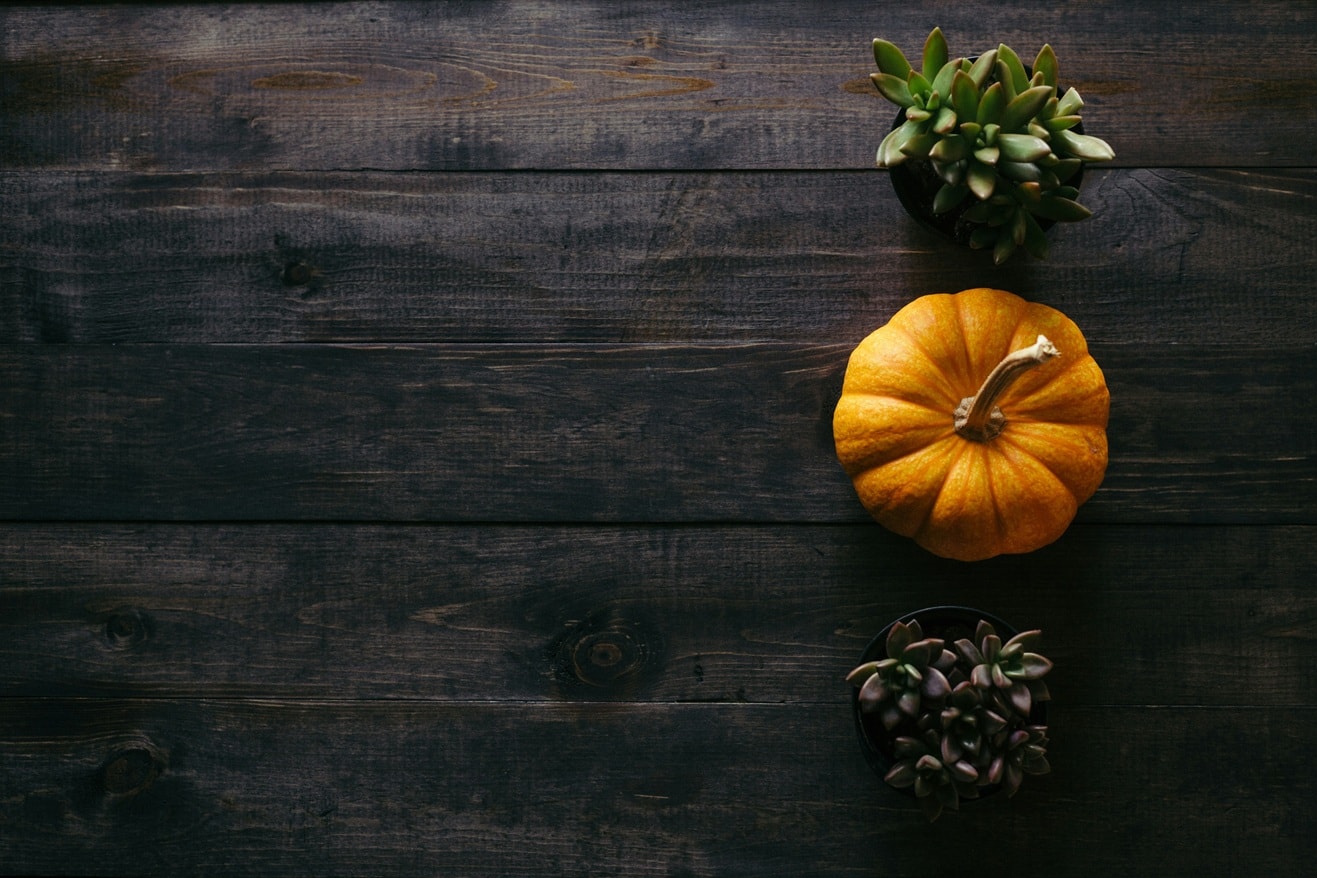
Comments
to join the conversation
Loading comments...