Step-by-Step Tutorial: Building a RESTful API with Supabase and Express.js for Dinosaur Facts
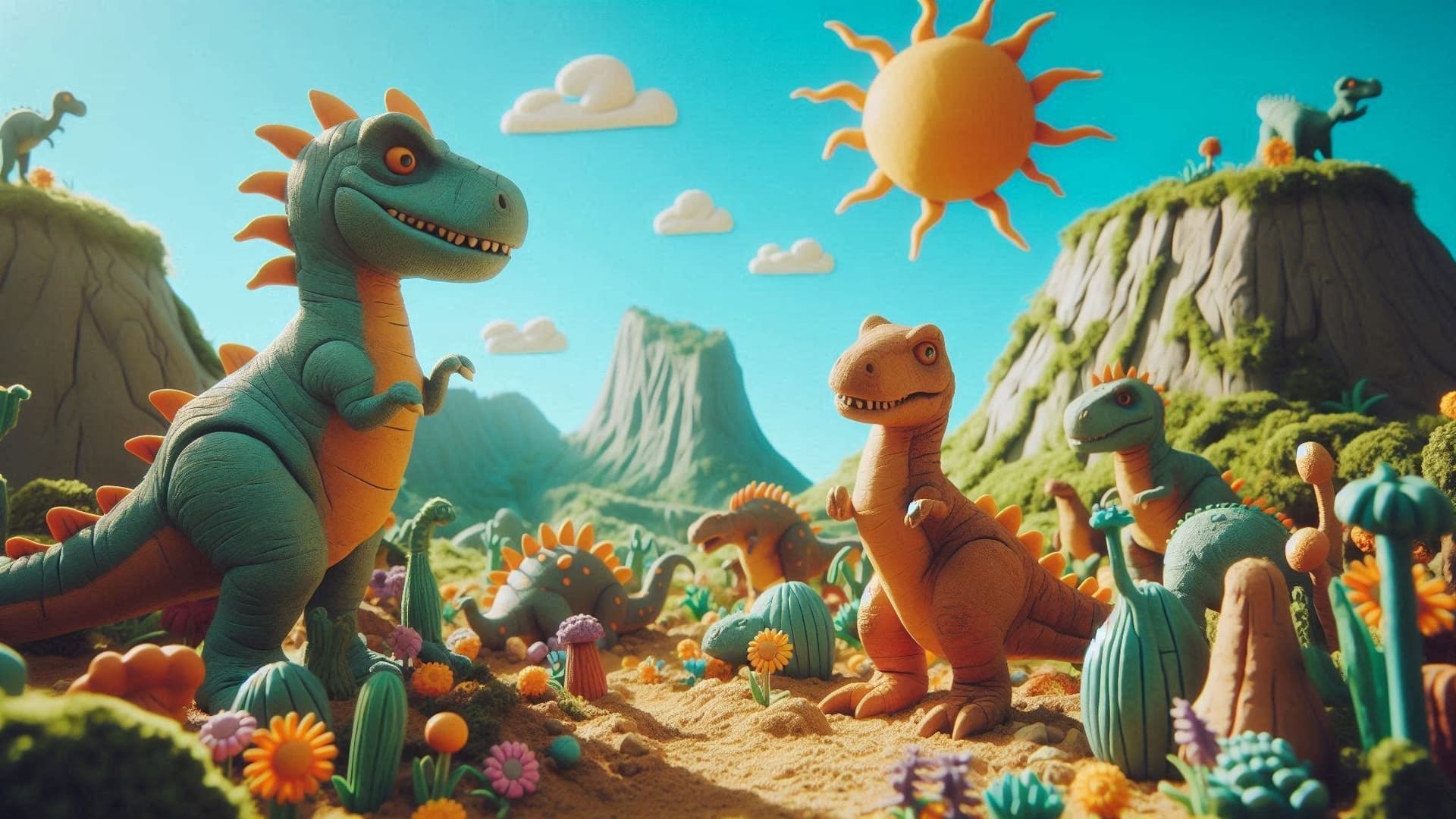
Welcome to this comprehensive tutorial on building a RESTful API using Node.js, Express.js, and Supabase for a Dinosaur Facts Database. Whether you're a new or experienced developer looking to enhance your skills, this guide will walk you through creating a robust API that allows users to query fascinating dinosaur facts by name.
In this project, we'll utilize the power of Express.js, a fast and minimalist web framework for Node.js, and Supabase, an open-source Firebase alternative that provides a real-time database. By the end of this tutorial, you'll have a fully functional API capable of serving detailed information about various dinosaurs from our curated dataset provided by https://Kaggle.com.
Step 1: Set Up the Environment
A. Create a new directory for your project:
mkdir express-dinosaur-api
cd express-dinosaur-api
B. Initialize a new Node.js project:
npm init -y
C. Install necessary packages:
npm install express @supabase/supabase-js dotenv
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It simplifies the process of building server-side applications by providing tools and libraries to handle routing, middleware, and HTTP requests.
advertisement
Step 2: Set Up Supabase
A. Create a Supabase account and project:
- Go to https://supabase.com, sign up, and create a new project.
B. Get your Supabase project credentials:
- In your Supabase dashboard, navigate to the "Settings", then "API" and get your project URL and API key.
C. Create a dinosaur_facts
table in your Supabase project:
- In the Supabase dashboard, go to the "SQL" tab and execute the following SQL script to create the
dinosaur_facts
table:
CREATE TABLE dinosaur_facts (
occurrence_no SERIAL PRIMARY KEY,
name TEXT NOT NULL,
diet TEXT,
type TEXT,
length_m FLOAT,
max_ma FLOAT,
min_ma FLOAT,
region TEXT,
lng FLOAT,
lat FLOAT,
class TEXT,
family TEXT
);
-
Download the dataset by clicking the button below:
-
Next, upload your dataset (e.g., CSV file) containing dinosaur facts into this table through the Supabase dashboard.
-
Click "Table Editor" tab on the left-side navigation menu.
-
Select the 'dinosaur_facts' table created ealier in this step (2-C).
-
Click "Import data via CSV" and follow the on screen prompts to upload the 'dinosaurs.csv' dataset.
-
Supabase is an open-source Firebase alternative that provides backend services like authentication, real-time databases, and storage. It is built on top of PostgreSQL and offers features such as auto-generated APIs, user management, and real-time subscriptions.
Step 3: Create the Express.js Application
A. Create a new file named server.js and open it in your text editor:
const express = require('express');
const { createClient } = require('@supabase/supabase-js');
require('dotenv').config();
const app = express();
const port = process.env.PORT || 3000;
// Initialize Supabase client
const supabaseUrl = process.env.SUPABASE_URL;
const supabaseKey = process.env.SUPABASE_KEY;
const supabase = createClient(supabaseUrl, supabaseKey);
// Middleware
app.use(express.json());
// Routes
app.get('/api/dinosaurs', async (req, res) => {
try {
let { data, error } = await supabase.from('dinosaur_facts').select('*');
if (error) throw error;
res.status(200).json(data);
} catch (error) {
console.error('Error fetching dinosaurs:', error.message);
res.status(500).json({ error: 'Error fetching dinosaurs' });
}
});
app.get('/api/dinosaurs/:name', async (req, res) => {
const { name } = req.params;
try {
let { data, error } = await supabase.from('dinosaur_facts').select('*').ilike('name', `%${name}%`);
if (error) throw error;
if (data.length > 0) {
res.status(200).json(data);
} else {
res.status(404).json({ message: 'Dinosaur not found' });
}
} catch (error) {
console.error('Error fetching dinosaur:', error.message);
res.status(500).json({ error: 'Error fetching dinosaur' });
}
});
// Start server
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
RESTful APIs are based on the principle of statelessness, meaning that each request from a client to a server must contain all the information needed to understand and process the request. The server does not store any state about the client session between requests, which simplifies scalability and improves performance.
advertisement
Step 4: Configure Environment Variables
A. Create a .env file in your project directory:
PORT=3000
SUPABASE_URL=your_supabase_url
SUPABASE_KEY=your_supabase_key
Replace your_supabase_url and your_supabase_key with your actual Supabase project URL and API key.
Express.js allows the use of middleware to handle requests, responses, and any other middleware logic in a flexible and modular way. Middleware functions can perform a variety of tasks like logging, authentication, data parsing, and error handling.
Step 5: Testing the API
A. Run your Express.js application:
node server.js
B. Use curl
or a tool like Postman to test the API:
Download and Install Curl:
-
Download the latest release at: https://curl.se/download.html
-
Extract the .zip
-
Add the location of the extracted folder to your system's PATH.
(e.g. 'C:\curl-8.8.0\curl-8.8.0')
Example Usage:
Get dinosaur by name (e.g., "Gorgosaurus"):
curl http://localhost:3000/api/dinosaurs/Gorgosaurus
Response:
The JSON response for this query might look like this:
[
{
"occurrence_no": 139242,
"name": "Gorgosaurus",
"diet": "carnivorous",
"type": "large theropod",
"length_m": 8.6,
"max_ma": 83.5,
"min_ma": 70.6,
"region": "Alberta",
"lng": -111.528732,
"lat": 50.740726,
"class": "Saurischia",
"family": "Tyrannosauridae"
},
{
"occurrence_no": 139250,
"name": "Gorgosaurus",
"diet": "carnivorous",
"type": "large theropod",
"length_m": 8.6,
"max_ma": 83.5,
"min_ma": 70.6,
"region": "Alberta",
"lng": -111.549347,
"lat": 50.737015,
"class": "Saurischia",
"family": "Tyrannosauridae"
}
]
Supabase leverages PostgreSQL, a powerful, open-source relational database known for its robustness and scalability. This allows developers to use advanced SQL features and ensures compatibility with a wide range of tools and libraries.
Explanation:
-
Express.js Setup: The application uses Express.js to handle HTTP requests and responses.
-
Supabase Integration: Supabase is integrated using the supabase-js client to perform database operations (selecting dinosaurs).
-
Environment Variables: Environment variables (PORT, SUPABASE_URL, SUPABASE_KEY) are used to configure the application and connect to Supabase securely.
advertisement
FAQ
What is Node.js? Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside a web browser. It's used for building fast and scalable server-side applications.
What is Express.js? Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It simplifies the development of server-side logic.
What is a RESTful API? A RESTful API is an architectural style for designing networked applications. It relies on a stateless, client-server, cacheable communications protocol — the HTTP protocol.
How does Express.js handle routing? Express.js handles routing using its .get(), .post(), .put(), .delete() methods, which correspond to HTTP GET, POST, PUT, and DELETE requests, respectively. Routes are defined based on URL paths.
What are middleware functions in Express.js? Middleware functions in Express.js are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. They can perform various tasks such as logging, authentication, and request parsing.
Why use Supabase with Express.js? Supabase is an open-source alternative to Firebase that provides a suite of backend services like authentication, real-time databases, and storage. Integrating Supabase with Express.js allows you to easily add these functionalities to your application without managing infrastructure.
advertisement
Resources and Documentation
Node.js Documentation:
- Node.js Official Documentation: https://nodejs.org/en/docs/
- Node.js API Reference: https://nodejs.org/en/docs/guides/
Express.js Documentation:
- Express.js Official Documentation: https://expressjs.com/en/starter/installing.html
- Express.js Guide: https://expressjs.com/en/guide/routing.html
RESTful API Design:
- REST API Tutorial: https://restfulapi.net/
- RESTful API Design Guide: https://www.restapitutorial.com/
Supabase Documentation:
- Supabase Official Documentation: https://supabase.io/docs
- Getting Started with Supabase: https://supabase.io/docs/guides/with-js
HTTP Methods:
- MDN Web Docs: HTTP Methods: https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods
JSON Guide:
- JSON Official Website: https://www.json.org/json-en.html
- MDN Web Docs: JSON: https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/JSON
Related Articles:
-
Embracing JavaScript: Unveiling the Strengths Beyond Traditional Languages
-
Create a Customizable and Reusable React Hook for Using Local Storage: A Step-by-Step Tutorial
-
Comparing Modern JavaScript Frameworks: React, Angular, and Vue in 2024
advertisement
Donate
If you enjoyed this article, please consider making a donation. Your support means a lot to me.
- Cashapp: $hookerhillstudios
- Paypal: Paypal
Conclusion
By following this tutorial, you've set up a RESTful API using Express.js and Supabase for managing and retrieving dinosaur facts. Users can query the API to fetch all dinosaur records or retrieve specific dinosaurs by name. This setup leverages Supabase for database management and Express.js for creating the API endpoints, providing a scalable and efficient solution for building your dinosaur fact database API using JavaScript.
About the Author
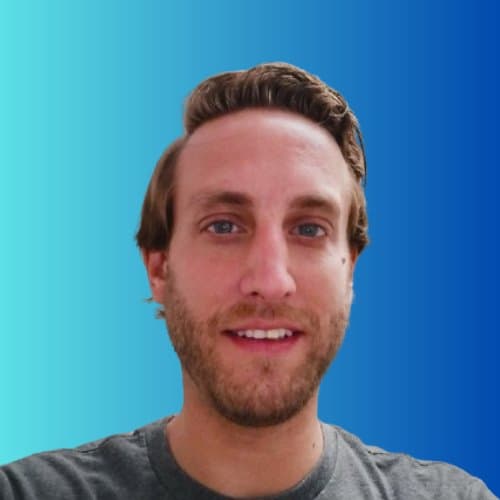
Hi, I'm Jared Hooker, and I have been passionate about coding since I was 13 years old. My journey began with creating mods for iconic games like Morrowind and Rise of Nations, where I discovered the thrill of bringing my ideas to life through programming.
Over the years, my love for coding evolved, and I pursued a career in software development. Today, I am the founder of Hooker Hill Studios, where I specialize in web and mobile development. My goal is to help businesses and individuals transform their ideas into innovative digital products.
Read Recent Articles
Recent Articles
View All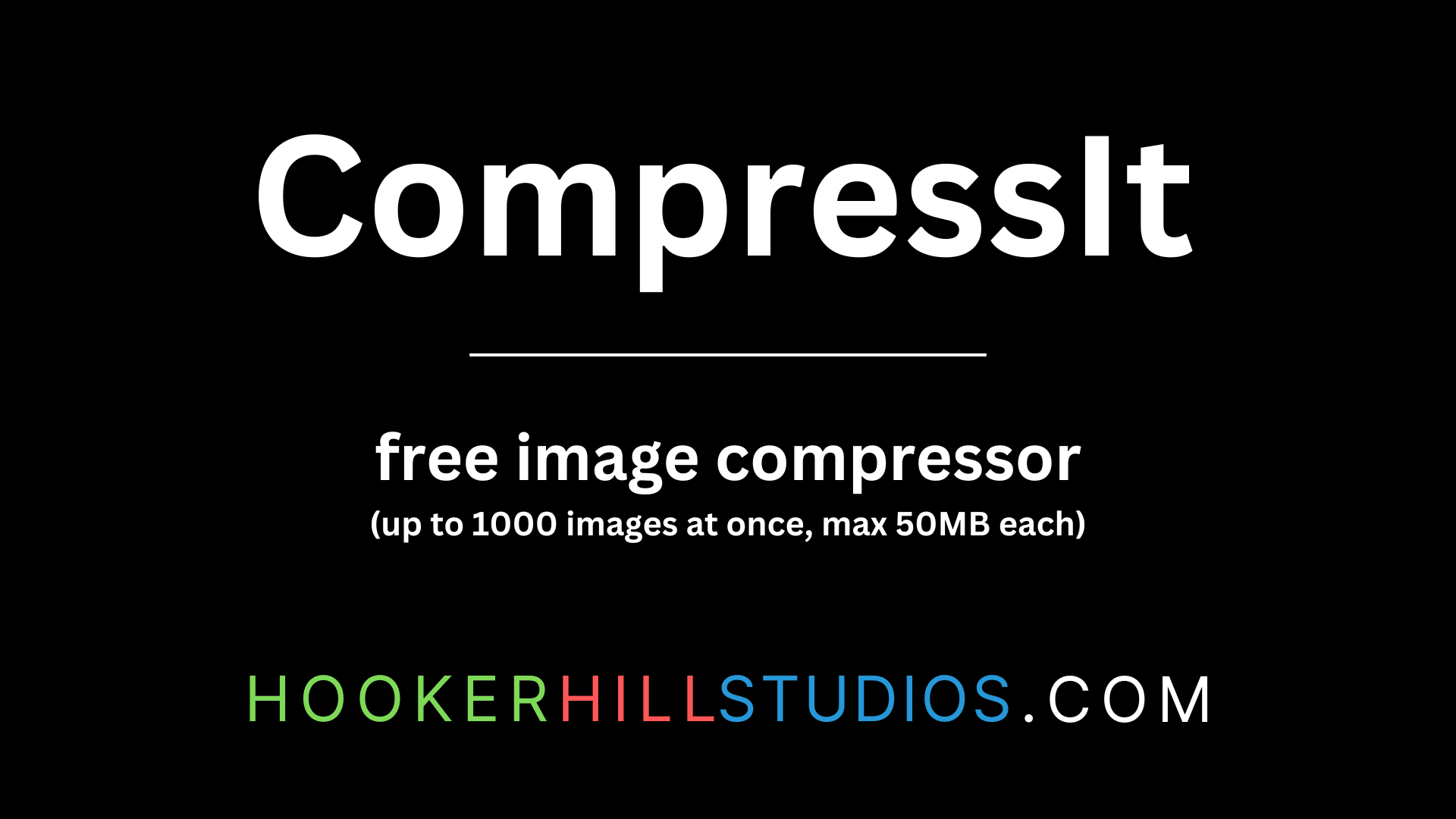
CompressIt - Fast & Efficient Image Compression Tool for Web Optimization
December 02, 2024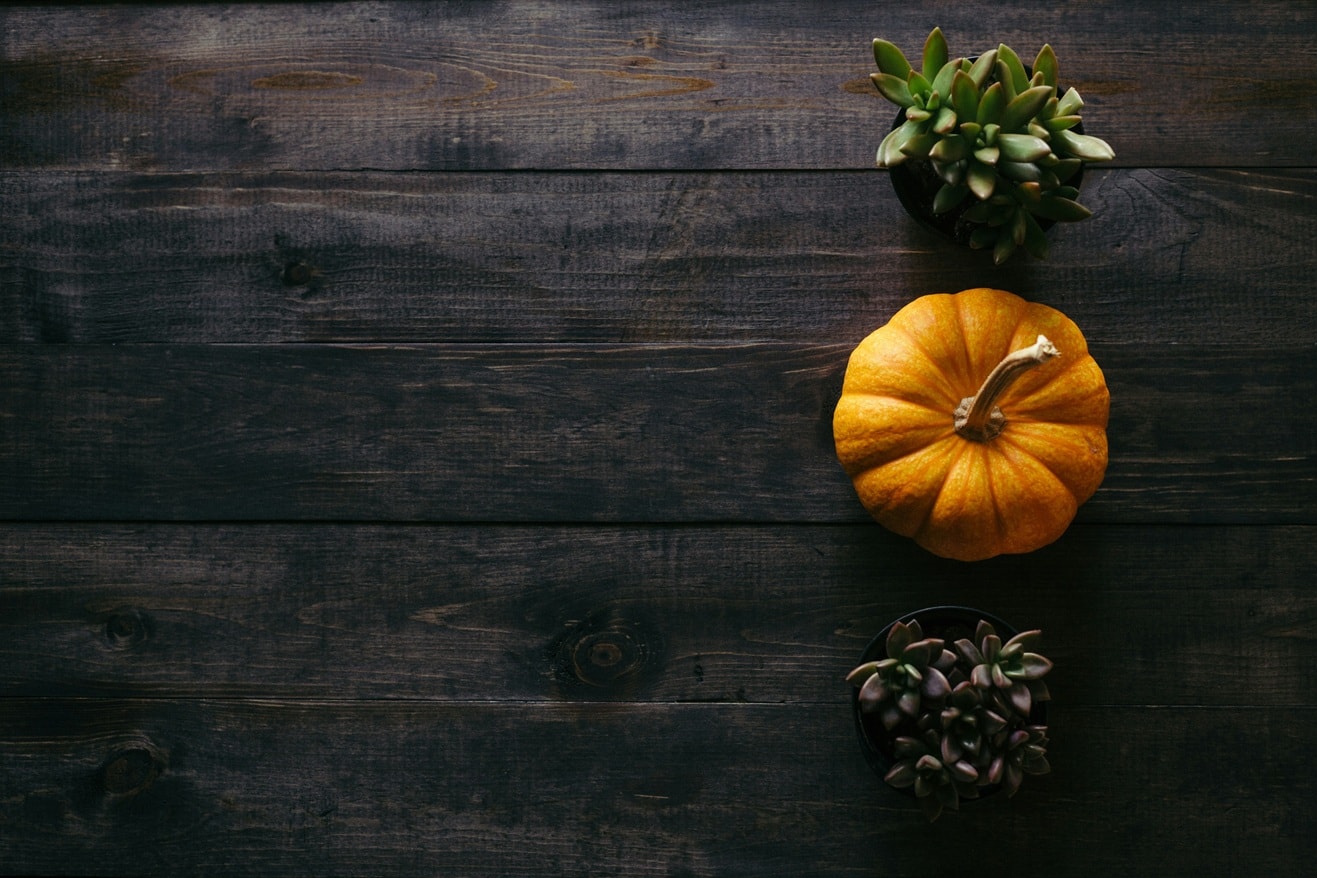
to join the conversation